Question
Add a sorting method to sort th ArrayList of Chicken objects by weight (lowest to highest), using any one of these sorting algorithms: Selection sort,
Add a sorting method to sort th ArrayList of Chicken objects by weight (lowest to highest), using any one of these sorting algorithms: Selection sort, Insertion sort, Quick sort, or Mergesort. Also in the comments above the method, describe why you chose this sorting algorithm over the others. [20 points]
Add a binary search method to search an ArrayList of Chicken objects, and return the index of the Chicken by a specified weight [20 points]
Add a recursive method called eatChicken(Chicekn c) that will recursively eat a chicken, by subtracting 1 lb of chicken for each recursive call. Use 0 lbs as the base case and print out "Done" if the chicken weight is 0. For each recursive case, print out "Yee Haw, there are N lbs. of chicken remaining" [30 points]
In the main method, you will make the following method calls
Call your sorting method to sort the ArrayList of Chicken objects [10 points]
Call the binary search method to find a Chicken that weighs 32 lbs, and get the index of that Chicken [10 points]
Call the recursive method by passing the Chicken object at the specified index found by the binary search method [10 points]
------------
public class Chicken {
private String type;
private int weight; //weight rounded up, in lbs.
public Chicken(String type, int weight) {
super();
this.type = type;
this.weight = weight;
}
/**
* @return the type
*/
public String getType() {
return type;
}
/**
* @param type the type to set
*/
public void setType(String type) {
this.type = type;
}
/**
* @return the weight
*/
public int getWeight() {
return weight;
}
/**
* @param weight the weight to set
*/
public void setWeight(int weight) {
this.weight = weight;
}
}
-----
import java.util.ArrayList;
public class Thanksgiving {
public static void main(String[] args) {
ArrayList
turkeys.add(new Chicken("Butterball", 20));
turkeys.add(new Chicken("Jenni-O", 12));
turkeys.add(new Chicken("Foster Farms", 24));
turkeys.add(new Chicken("Honeysuckle", 17));
turkeys.add(new Chicken("Trader Joes", 32));
turkeys.add(new Chicken("Norbest", 10));
turkeys.add(new Chicken("Vons O Organics", 38));
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
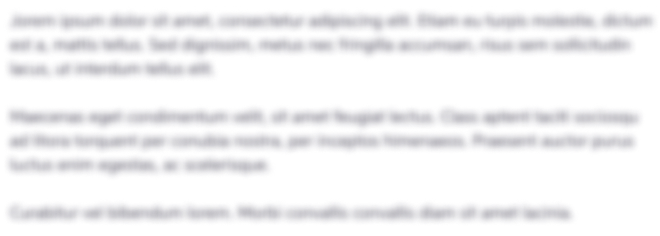
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started