Question
Add a static merge() method to the class OrderedArrayApp so that you can merge two ordered source arrays into an ordered destination array. The method
- Add a static merge() method to the class OrderedArrayApp so that you can merge two ordered source arrays into an ordered destination array. The method should have the method heading like
public static OrderedArray merge (OrderedArray src1, OrderedArray src2)
Write code in main() of OrderedArrayApp.java that inserts some random numbers into the two source arrays, calls merge(), and displays the contents of the resulting destination array. The source arrays may hold different numbers of data items. In your algorithm, you will need to compare the elements of the source arrays, picking the smaller one to copy to the destination array. Youll also need to handle the situation when one source array exhausts its contents before the other.
For example, assume two sources arrays are:
srcArray1: 12 28 30
srcArray2: 2 4 11 19 25 50 89
Then, the destination array should be:
desArray: 2 4 11 12 19 25 28 30 50 89
- Add a method called median() to the ArrayIns class in the insertSort.java program. This method should return the median value in the array. (Recall that in a group of numbers half are larger than the median and half are smaller.) Do it the easy way.
- Another simple sort is the odd-even sort. The idea is to repeatedly make two passes through the array. On the first pass you look at all the pairs of items, a[j] and a[j+1], where j is odd (j = 1, 3, 5, ). If their key values are out of order, you swap them. On the second pass you do the same for all the even values (j = 2, 4, 6, ). You do these two passes repeatedly until the array is sorted. Replace the bubbleSort() method in bubbleSort.java with an oddEvenSort() method. Make sure it works for varying amounts of data. Youll need to figure out how many times to do the two passes. Use big O notation to give your answers.
The odd-even sort is actually useful in a multiprocessing environment, where a separate processor can operate on each odd pair simultaneously and then on each even pair. Because the odd pairs are independent of each other, each pair can be checkedand swapped, if necessaryby a different processor. This makes for a very fast sort.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
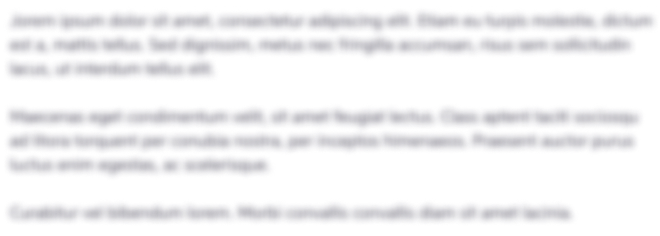
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started