Question
Add additional information to the student class below such as name, address, major, and contact information along with all the getters and setters (methods) needed
Add additional information to the student class below such as name, address, major, and contact information along with all the getters and setters (methods) needed to access all data. Submit your code along with a sample run of your program.
Create the code and sample run files.
// ShowStudent.java
// client to test the Student class
class ShowStudent
{
public static void main (String args[])
{
Student pupil = new Student(); // We need a new student named pupil
pupil.setIDnumber(234); // We are setting pupil\\\'s ID number to 234
pupil.setPoints(47); // We are setting pupil\\\'s points to 47
pupil.setHours(15); // We are setting pupil\\\'s hours to 15
pupil.showIDnumber(); // We are showing pupil\\\'s ID number
pupil.showPoints(); // We are showing pupil\\\'s points
pupil.showHours(); // We are showing pupil\\\'s hourse
System.out.println("The grade point average is " +
pupil.getGradePoint()); // we are calculating pupil\\\'s GPA
}
}
class Student // this is the student class
{
// the private data members
private int idNumber;
private int hours;
private int points;
// Constructor creates a new student
Student()
{
idNumber = 9999; // a max ID
points = 12; // starting out assuming 12 points
hours = 3; // starting out assuming 3 hours
}
// end of constructor
// the public get and set methods fro pulil specific information
public void setIDnumber(int number) // pass in a number
{
idNumber = number; // assign the number
}
public int getIDnumber() // return a student ID
{
return idNumber;
}
public void setHours(int number) // pass in a number
{
hours = number; // assign the number to hours
}
public int getHours() // return the student\\\'s hours
{
return hours;
}
public void setPoints(int number) // pass in a number
{
points = number; // assign number to points
}
public int getPoints() // return the points
{
return points;
}
// methods to display the fields
public void showIDnumber()
{
System.out.println("ID Number is: " + idNumber);
}
public void showHours()
{
System.out.println("Credit Hours: " + hours);
}
public void showPoints()
{
System.out.println("Points Earned: " + points);
}
public double getGradePoint() // calculate the GPA with internal data
{
return (points * 1.0 / hours);
// simple integer division will truncate the decimal places
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
ShowStudentjava class Student This is a class of students Member of personal data Private private ID int Private hours Private custom point More infor...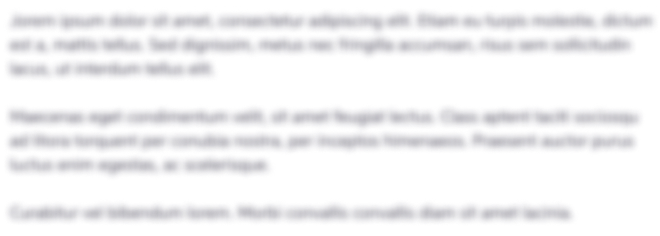
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started