Add delete at a position to the code and display it to the code: class listnodes { int data; listnodes link; listnodes() { data =
Add delete at a position to the code and display it to the code:
class listnodes { int data; listnodes link;
listnodes() { data = 0; link = null; }
listnodes(int d, listnodes l) { data = d; link = l; } } class singlelinkedlist { public listnodes insertnode(int data, listnodes head) {
listnodes newnode = new listnodes(data, null);
newnode.link = head;
head = newnode; return head; }
public listnodes insertAtPosition(listnodes head, int data, int position) { listnodes newnode = new listnodes(data, null); listnodes previous = head; int count = 1; while (count <= position - 1) { previous = previous.link; count++; }
listnodes current = previous.link; newnode.link = current; previous.link = newnode; return head; }
public listnodes deletefirst(listnodes head) { listnodes pre = head; head = head.link; pre.link = null; return pre; }
public void display(listnodes head) { listnodes current = head; while (current.link != null) { System.out.print(current.data + "-->"); current = current.link; } System.out.print(current.data); }
} public class LinkedList {
public static void main(String[] args) { listnodes head=new listnodes(10,null); singlelinkedlist sl=new singlelinkedlist(); sl.display(head);
System.out.println(" Insert at front"); listnodes newhead=sl.insertnode(20, head); sl.display(newhead);
System.out.println(" Insert at a position"); sl.insertAtPosition(newhead, 40, 1); sl.display(newhead);
System.out.println(" Delete first"); listnodes newhead2=sl.deletefirst(newhead); sl.display(newhead2);
} }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
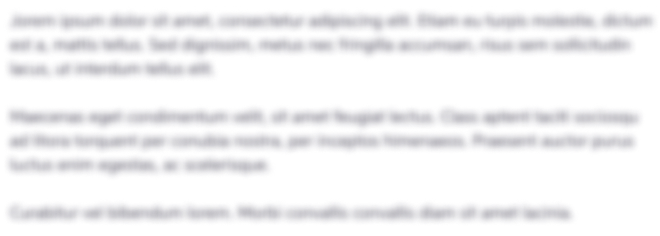
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started