Question
Add documentation comments (also referred to as javadoc comments) to all of the methods of your Policy class. (see code at end of question from
Add documentation comments (also referred to as "javadoc" comments) to all of the methods of your Policy class. (see code at end of question from previous work)
Modify the Demo class to read information about a set of Insurance Policies from a text file (named PolicyInformation.txt) instead of asking the user for input. The text file contains information in the following order:
Policy Number
Provider Name
Policyholders First Name
Policyholders Last Name
Policyholders Age
Policyholders Smoking Status (will be smoker or non-smoker)
Policyholders Height (in inches)
Policyholders Weight (in pounds)
PolicyInformation.txt data
3450 State Farm Alice Jones 20 smoker 65 110 3455 Aetna Bob Lee 54 non-smoker 72 200 2450 Met Life Chester Williams 40 smoker 71 300 3670 Global Cindy Smith 55 non-smoker 62 140 1490 Reliable Jenna Lewis 30 smoker 60 105 3477 State Farm Craig Duncan 23 smoker 66 215
The program should create Policy objects for each of the policies contained in the file, and should be able to handle a file of any size.
The Policy objects should be stored in a structure that can automatically adjust to accommodate the number of objects being stored in it.
The demo program should display the same information as the output in Project 1 for each of the Policy objects by iterating over the structure that the Policy objects are being stored in.
The demo program should display the number of Policyholders that are smokers and the number of Policyholders that are non-smokers.
Sample Output
Policy Number: 3450
Provider Name: State Farm
Policyholder's First Name: Alice
Policyholder's Last Name: Jones
Policyholder's Age: 20
Policyholder's Smoking Status (smoker/non-smoker): smoker
Policyholder's Height: 65.0 inches
Policyholder's Weight: 110.0 pounds
Policyholder's BMI: 18.30
Policy Price: $700.00
Policy Number: 3455
Provider Name: Aetna
Policyholder's First Name: Bob
Policyholder's Last Name: Lee
Policyholder's Age: 54
Policyholder's Smoking Status (smoker/non-smoker): non-smoker
Policyholder's Height: 72.0 inches
Policyholder's Weight: 200.0 pounds
Policyholder's BMI: 27.12
Policy Price: $675.00
Policy Number: 2450
Provider Name: Met Life
Policyholder's First Name: Chester
Policyholder's Last Name: Williams
Policyholder's Age: 40
Policyholder's Smoking Status (smoker/non-smoker): smoker
Policyholder's Height: 71.0 inches
Policyholder's Weight: 300.0 pounds
Policyholder's BMI: 41.84
Policy Price: $836.74
Policy Number: 3670
Provider Name: Global
Policyholder's First Name: Cindy
Policyholder's Last Name: Smith
Policyholder's Age: 55
Policyholder's Smoking Status (smoker/non-smoker): non-smoker
Policyholder's Height: 62.0 inches
Policyholder's Weight: 140.0 pounds
Policyholder's BMI: 25.60
Policy Price: $675.00
Policy Number: 1490
Provider Name: Reliable
Policyholder's First Name: Jenna
Policyholder's Last Name: Lewis
Policyholder's Age: 30
Policyholder's Smoking Status (smoker/non-smoker): smoker
Policyholder's Height: 60.0 inches
Policyholder's Weight: 105.0 pounds
Policyholder's BMI: 20.50
Policy Price: $700.00
Policy Number: 3477
Provider Name: State Farm
Policyholder's First Name: Craig
Policyholder's Last Name: Duncan
Policyholder's Age: 23
Policyholder's Smoking Status (smoker/non-smoker): smoker
Policyholder's Height: 66.0 inches
Policyholder's Weight: 215.0 pounds
Policyholder's BMI: 34.70
Policy Price: $700.00
The number of policies with a smoker is: 4
The number of policies with a non-smoker is: 2
-
** PREVIOUS CODE TO USE **
Policy.java
public class Policy { //fields private String policyNumber; private String providerName; private String firstName; private String lastName; private int age; private String smokingStatus; private double height; private double weight;
//constructors public Policy() { policyNumber = ""; providerName = ""; firstName = ""; lastName = ""; age = 0; smokingStatus = ""; height = 0; weight = 0; } public Policy(String pNumber, String pName, String fName, String lName,int a, String sStatus, double h, double w) { policyNumber = pNumber; providerName = pName; firstName = fName; lastName = lName; age = a; smokingStatus = sStatus; height = h; weight = w; } //setters//
public void setPolicyNumber(String pNumber) { policyNumber = pNumber; } public void setProviderName(String pName) { providerName = pName; } public void setFirstName(String fName) { firstName = fName; } public void setLastName(String lName) { lastName = lName; } public void setAge(int a) { age = a; } public void setSmokingStatus(String sStatus) { smokingStatus = sStatus; } public void setHeight(double h) { height = h; } public void setWeight(double w) { weight = w; }
//getters// public String getPolicyNumber() { return policyNumber; } public String getProviderName() { return providerName; } public String getFirstName() { return firstName; } public String getLastName() { return lastName; } public int getAge() { return age; } public String getSmokingStatus() { return smokingStatus; } public double getHeight() { return height; } public double getWeight() { return weight; } //Calculates the Policyholder's BMI public double getBMI() { final double CONVFACTOR = 703; return (weight * CONVFACTOR) / (height * height); } //Calculates the Policy's price public double getPrice() { final double BASE_PRICE = 600; final double ADDITIONAL_FEE_AGE = 75; final double ADDITIONAL_FEE_SMOKING = 100; final double ADDITIONAL_FEE_PER_BMI = 20; final int AGE_THRESHOLD = 50; final int BMI_THRESHOLD = 35; double price = BASE_PRICE; if(age > AGE_THRESHOLD) //over 50 years price += ADDITIONAL_FEE_AGE; //75 if(smokingStatus.equalsIgnoreCase("smoker")) price += ADDITIONAL_FEE_SMOKING; //100 //call the getBMI method if(getBMI() > BMI_THRESHOLD) //BMI over 35 price += ((getBMI() - BMI_THRESHOLD) * ADDITIONAL_FEE_PER_BMI); //additional BMI fee - 20 return price; } //Not included in the instructions but can be added... /*Displays information about the Policy public void displayInformation() { System.out.println("Policy Number: " + policyNumber); System.out.println("Provider Name: " + providerName); System.out.println("Policyholder's First Name: " + firstName); System.out.println("Policyholder's Last Name: " + lastName); System.out.println("Policyholder's Age: " + age); System.out.println("Policyholder's Smoking Status (Y/N): " + smokingStatus); System.out.println("Policyholder's Height: " + height + " inches"); System.out.println("Policyholder's Weight: " + weight + " pounds"); System.out.printf("Policyholder's BMI: %.2f ", getBMI()); System.out.printf("Policy Price: $%.2f ", getPrice()); } */ }
PolicyDemo.java
import java.util.Scanner;
public class PolicyDemo { public static void main(String[] args) { //declare variables String policyNumber; String providerName; String firstName; String lastName; int age; String smokingStatus; double height; double weight; Scanner keyboard = new Scanner(System.in); //prompt the user to enter information about the Policy System.out.print("Please enter the Policy Number: "); policyNumber = keyboard.nextLine(); System.out.print("Please enter the Provider Name: "); providerName = keyboard.nextLine(); System.out.print("Please enter the Policyholder's First Name: "); firstName = keyboard.nextLine(); System.out.print("Please enter the Policyholder's Last Name: "); lastName = keyboard.nextLine(); System.out.print("Please enter the Policyholder's Age: "); age = keyboard.nextInt(); keyboard.nextLine(); System.out.print("Please enter the Policyholder's Smoking Status (smoker/non-smoker): "); smokingStatus = keyboard.nextLine(); System.out.print("Please enter the Policyholder's Height (in inches): "); height = keyboard.nextDouble(); System.out.print("Please enter the Policyholder's Weight (in pounds): "); weight = keyboard.nextDouble(); //create a Policy object Policy policy = new Policy(policyNumber, providerName, firstName, lastName, age, smokingStatus, height, weight); //put a blank line before the output System.out.println(); //display information about the Policy System.out.println("Policy Number: " + policy.getPolicyNumber()); System.out.println("Provider Name: " + policy.getProviderName()); System.out.println("Policyholder's First Name: " + policy.getFirstName()); System.out.println("Policyholder's Last Name: " + policy.getLastName()); System.out.println("Policyholder's Age: " + policy.getAge()); System.out.println("Policyholder's Smoking Status: " + policy.getSmokingStatus()); System.out.println("Policyholder's Height: " + policy.getHeight() + " inches"); System.out.println("Policyholder's Weight: " + policy.getWeight() + " pounds"); System.out.printf("Policyholder's BMI: %.2f ", policy.getBMI()); System.out.printf("Policy Price: $%.2f ", policy.getPrice()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
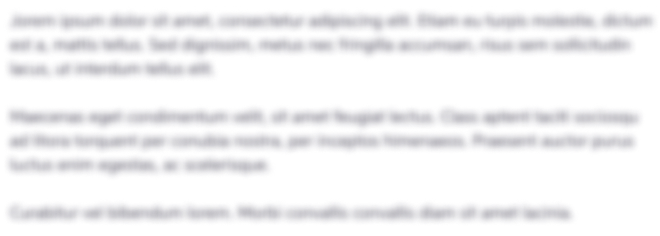
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started