Question
Add functions push(int n, Deque &dq) and pop(Deque &dq). Functions push(int n, Deque &dq) and pop(Deque &dq) should call functions of the Deque class to
Add functions push(int n, Deque &dq) and pop(Deque &dq).
Functions push(int n, Deque &dq) and pop(Deque &dq) should call functions of the Deque class to simulate a stack's push and pop functions.
The Deque class should not be touched. I will use the original unmodified Deque class when testing your code.
Demonstrate to convert a base 10 number to base 2. Ask the user to enter a base 10 number, output the equivalent base 2 number. The main() function would create a Deque object and pass it by reference when calling the new
push(int n, Deque &dq) and pop(Deque &dq) functions simulating stack operations.
keep it simple and to the instructions and code provided please! and thank you!
// C++ implementation of De-queue using circular
// array
#include
using namespace std;
// Maximum size of array or Dequeue
#define MAX 100
// A structure to represent a Deque
class Deque
{
int arr[MAX];
int front;
int rear;
int size;
public :
Deque(int size)
{
front = -1;
rear = 0;
this->size = size;
}
// Operations on Deque:
void insertfront(int key);
void insertrear(int key);
void deletefront();
void deleterear();
bool isFull();
bool isEmpty();
int getFront();
int getRear();
};
// Checks whether Deque is full or not.
bool Deque::isFull()
{
return ((front == 0 && rear == size-1)||
front == rear+1);
}
// Checks whether Deque is empty or not.
bool Deque::isEmpty ()
{
return (front == -1);
}
// Inserts an element at front
void Deque::insertfront(int key)
{
// check whether Deque if full or not
if (isFull())
{
cout << "Overflow " << endl;
return;
}
// If queue is initially empty
if (front == -1)
{
front = 0;
rear = 0;
}
// front is at first position of queue
else if (front == 0)
front = size - 1 ;
else // decrement front end by '1'
front = front-1;
// insert current element into Deque
arr[front] = key ;
}
// function to inset element at rear end
// of Deque.
void Deque ::insertrear(int key)
{
if (isFull())
{
cout << " Overflow " << endl;
return;
}
// If queue is initially empty
if (front == -1)
{
front = 0;
rear = 0;
}
// rear is at last position of queue
else if (rear == size-1)
rear = 0;
// increment rear end by '1'
else
rear = rear+1;
// insert current element into Deque
arr[rear] = key ;
}
// Deletes element at front end of Deque
void Deque ::deletefront()
{
// check whether Deque is empty or not
if (isEmpty())
{
cout << "Queue Underflow " << endl;
return ;
}
// Deque has only one element
if (front == rear)
{
front = -1;
rear = -1;
}
else
// back to initial position
if (front == size -1)
front = 0;
else // increment front by '1' to remove current
// front value from Deque
front = front+1;
}
// Delete element at rear end of Deque
void Deque::deleterear()
{
if (isEmpty())
{
cout << " Underflow " << endl ;
return ;
}
// Deque has only one element
if (front == rear)
{
front = -1;
rear = -1;
}
else if (rear == 0)
rear = size-1;
else
rear = rear-1;
}
// Returns front element of Deque
int Deque::getFront()
{
// check whether Deque is empty or not
if (isEmpty())
{
cout << " Underflow " << endl;
return -1 ;
}
return arr[front];
}
// function return rear element of Deque
int Deque::getRear()
{
// check whether Deque is empty or not
if(isEmpty() || rear < 0)
{
cout << " Underflow " << endl;
return -1 ;
}
return arr[rear];
}
// Driver program to test above function
int main()
{
Deque dq(5);
cout << "Insert element at rear end : 5 ";
dq.insertrear(5);
cout << "insert element at rear end : 10 ";
dq.insertrear(10);
cout << "get rear element " << " "
<< dq.getRear() << endl;
dq.deleterear();
cout << "After delete rear element new rear"
<< " become " << dq.getRear() << endl;
cout << "inserting element at front end ";
dq.insertfront(15);
cout << "get front element " << " "
<< dq.getFront() << endl;
dq.deletefront();
cout << "After delete front element new "
<< "front become " << dq.getFront() << endl;
return 0;
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
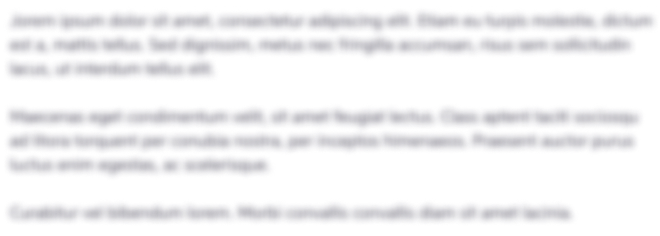
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started