Question
ADD method T Minimum () which return smallest item/node value LinkedListLibrary using System; namespace LinkedListLibrary { // class to represent one node in a list
ADD method T Minimum
LinkedListLibrary using System;
namespace LinkedListLibrary { // class to represent one node in a list class ListNode
public List() : this("list") { }
public void Count() { int counter = 0; ListNode
public void Search(T insertItem) { ListNode
public void InsertAtFront(T insertItem) { if (IsEmpty()) { firstNode = lastNode = new ListNode
public void InsertAtBack(T insertItem) { if (IsEmpty()) { firstNode = lastNode = new ListNode
public T RemoveFromFront() { if (IsEmpty()) { throw new EmptyListException(name); }
T removeItem = firstNode.Data; if (firstNode == lastNode) { firstNode = lastNode = null; } else { firstNode = firstNode.Next; }
return removeItem; }
public T RemoveFromBack() { if (IsEmpty()) { throw new EmptyListException(name); }
T removeItem = lastNode.Data; if (firstNode == lastNode) { firstNode = lastNode = null; } else { ListNode
while (current.Next != lastNode) { current = current.Next; }
lastNode = current; current.Next = null; }
return removeItem; }
public bool IsEmpty() { return firstNode == null; }
public void Display() { if (IsEmpty()) { Console.WriteLine($"Empty {name}"); } else { Console.Write($"The {name} is: ");
ListNode
while (current != null) { Console.Write($"{current.Data} "); current = current.Next; }
Console.WriteLine(" "); } } } public class EmptyListException : Exception { public EmptyListException() : base("The list is empty") { }
public EmptyListException(string name) : base($"The {name} is empty") { }
public EmptyListException(string exception, Exception inner) : base(exception, inner) { } } }
LinkedListTest
// Fig. 19.5: ListTest.cs // Testing class List. using System; using LinkedListLibrary;
// class to test List class functionality class ListTest { static void Main() { var list = new List
list.InsertAtFront(34.56); list.Display(); list.InsertAtFront(56.43); list.Display(); list.InsertAtBack(743.3321); list.Display(); list.InsertAtBack(534.21); list.Display(); list.Search(32.1); list.Count(); list.Avg();
// remove data from list and display after each removal try { object removedObject = list.RemoveFromFront(); Console.WriteLine($"{removedObject} removed"); list.Display();
removedObject = list.RemoveFromFront(); Console.WriteLine($"{removedObject} removed"); list.Display();
removedObject = list.RemoveFromBack(); Console.WriteLine($"{removedObject} removed"); list.Display();
removedObject = list.RemoveFromBack(); Console.WriteLine($"{removedObject} removed"); list.Display(); } catch (EmptyListException emptyListException) { Console.Error.WriteLine($" {emptyListException}"); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
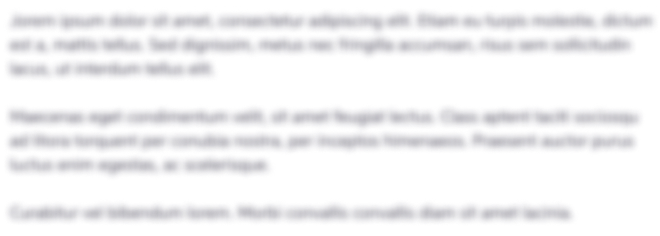
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started