Question
Add printRang method to BST.java that, given a low key value, and high key value, print all records in a sorted order whose values fall
Add printRang method to BST.java that, given a low key value, and high key value, print all records in a sorted order whose values fall between the two given keys. (Both low key and high key do not have to be a key on the list).
BST.java
import java.lang.Comparable;
/** Binary Search Tree implementation for Dictionary ADT */ class BST
/** Constructor */ BST() { root = null; nodecount = 0; }
/** Reinitialize tree */ public void clear() { root = null; nodecount = 0; }
/** Insert a record into the tree. @param k Key value of the record. @param e The record to insert. */ public void insert(Key k, E e) { root = inserthelp(root, k, e); nodecount++; }
// Return root
public BSTNode getRoot() { return root; }
/** Remove a record from the tree. @param k Key value of record to remove. @return The record removed, null if there is none. */ public E remove(Key k) { E temp = findhelp(root, k); // First find it if (temp != null) { root = removehelp(root, k); // Now remove it nodecount--; } return temp; }
/** Remove and return the root node from the dictionary. @return The record removed, null if tree is empty. */ public E removeAny() { if (root == null) return null; E temp = root.element(); root = removehelp(root, root.key()); nodecount--; return temp; }
/** @return Record with key value k, null if none exist. @param k The key value to find. */ public E find(Key k) { return findhelp(root, k); }
/** @return The number of records in the dictionary. */ public int size() { return nodecount; } private E findhelp(BSTNode
private BSTNode
private BSTNode
private StringBuffer out;
public String toString() { out = new StringBuffer(400); printhelp(root); return out.toString(); } private void printVisit(E it) { out.append(it + " "); }
}
BSTNode.java
class BSTNode
/** Constructors */ public BSTNode() {left = right = null; } public BSTNode(Key k, E val) { left = right = null; key = k; element = val; } public BSTNode(Key k, E val, BSTNode
/** Get and set the key value */ public Key key() { return key; } public void setKey(Key k) { key = k; }
/** Get and set the element value */ public E element() { return element; } public void setElement(E v) { element = v; }
/** Get and set the left child */ public BSTNode
/** Get and set the right child */ public BSTNode
/** @return True if a leaf node, false otherwise */ public boolean isLeaf() { return (left == null) && (right == null); } }
BinNode.java
/** ADT for binary tree nodes */ public interface BinNode
/** @return The left child */ public BinNode
/** @return The right child */ public BinNode
/** @return True if a leaf node, false otherwise */ public boolean isLeaf(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
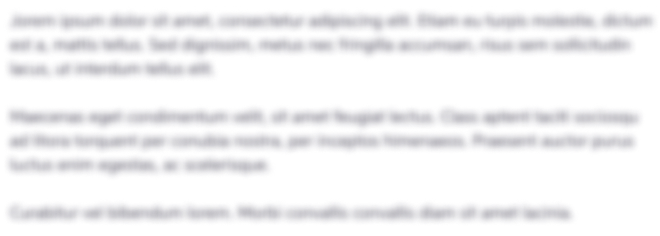
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started