Question
ADVANCED OBJECT ORIENTED PROGRAMMING ASSIGNMENT 4 Simple Java Pokemon-like game in which a player attempts to capture creatures. This will involve: Designing and implementing a
ADVANCED OBJECT ORIENTED PROGRAMMING ASSIGNMENT 4
Simple Java Pokemon-like game in which a player attempts to capture creatures. This will involve:
Designing and implementing a Player class that keeps track of player-related information.
Designing and implementing a Creature class that handles the players attempts to capture each creature (a new Creature object will be constructed for each encounter).
Modifying a given partially completed CreatureCapture application to call these methods while running the game.
In addition, you will be producing a simple design document in advance of the final implementation, listing the methods your Player and Creature support classes will implement.
You will also create some simple Junit tests for your Player class.
The Player object--- You are to design and implement a Player class that keeps track of player-related information, and provides methods that allows the CreatureCapture application to access and manipulate that information during the course of a game.The methods and constructors for this class will be based on the following game rules:
The player has an energy level. The level is 100 at the start of the game, and is printed out each round. Each action taken by the player decreases that energy. When it reaches 0, the game is over: You ran out of energy! Game over!
The player can use different weapons to try to capture creatures. Each turn, the application will prompt for which to use: You can f) Fire a freeze ray w) Cast a web b) Throw a force bubble What is your choice: Each has a different cost to their energy level:
Web Cast: Energy cost of 10.
Force Bubble: Energy cost of 20.
Freeze Ray: The player is prompted for how much energy to use: What is your choice: f How much energy do you want to use: 12 Actions that require more energy that the player currently has are not allowed:Your energy level is currently 18
You can f) Fire a freeze ray w) Cast a web b) Throw a force bubble What is your choice: b You don't have enough energy! Throwing a force bubble has cost 20. Finally, when a creature is captured, its energy is added to the players. For example, if the creatures energy were 22:
What is your choice: w The creature is caught in your web! You have gained 22 energy! Your energy level is currently 50 The Creature objects --- You are to design and implement a Creature class that keeps track of creature-related information, and provides methods that allows the CreatureCapture application to access and manipulate that information during the course of a game (specifically, when the player uses weapons).
The methods and constructors for this class will be based on the following game rules: Each Creature object has a speed and a strength level.
These are set to random values between 1 and 25 when the creature is constructed. -Each player weapon has a different effect: o Casting a web captures the creature if its speed is less than 5.
The creature is caught in your web! Otherwise, it has no effect on the creature: The creature is too fast for your web! o Throwing a force bubble captures the creature if its strength is less than 10.
The creature is trapped in the bubble! Otherwise, it has no effect on the creature: The creature breaks free of the bubble! o Firing a freeze ray decreases both the speed and the strength of the creature by the (amount of energy used)/2.
For example, if the creature has speed 23 and strength 17, and the player fires a freeze ray with energy 12, the creature speed is decreased to 17 and the strength decreased to 11. The creature appears slower and weaker! If this decreases either speed or strength to less than 0, the creature is captured: You have frozen the creature! When a creature is captured, the player is rewarded with energy equivalent to the original speed + the original strength of the creature.
The CreatureCapture Application--- You have been provided with a partial CreatureCapture application. It contains an outer loop that runs as long as the player still has energy, and an inner loop that runs for each creature until it has been captured (or the player runs out of energy).It also contains a great deal of empty space, along with documentation about what should go into that section of code, and what should be printed. This is where you will add calls to the methods in your Player and Creature classes.
Design Requirements --- I am not specifying the methods and constructors for your Player and Creature classes. These are the main design decisions for this assignment!
Separating the Logic and the User Interface All of the logic for the game must be handled within your Player and Creature classes.
The Player class is solely responsible for keeping track of the player energy level, the effect of using the different weapons on the energy level, and whether or not there is enough energy left to use a weapon.The Creature class is solely responsible for keeping track of the creature speed and strength, the effect of a freeze ray on those levels, and whether the creature has been captured when the player uses a weapon. On the other hand, the CreatureCapture application class is responsible for all of the I/O. In other words, the Player and Creature classes are not to print anything.Neither are they to return any messages to be printed by the application methods in the Player and Creature classes should only return numeric or Boolean values (as a justification, your company plans to market the applications in other languages).
Static Constants -There are a very large number of numeric values in this game, including the initial player energy, the costs of firing a web or a force bubble, and the thresholds at which they capture the creature. In order to make these easier to modify in future versions of the game, you are to store them as static constants.Note that some of these (particularly the costs of firing a web or a force bubble) are also used in messages printed in the application. You might consider making them public to allow the application to access them.
Encapsulation- Your support classes must be properly encapsulated.That means all member variables must be private. The only exception would be any static constants the application might use.
Documentation - As with any program, your source code should be well documented! For this assignment, this includes the following: For the Player and Creature classes, make sure that you put a header at the top of each method and constructor that briefly explains what it does (particularly in terms of the member variables). For the application, make sure that you explain how they work, particularly in terms of manipulating the Player and Creature objects.
Note that this documentation is to be in JavaDoc format. That is, I should be able to run your classes through the JavaDoc tool and see your documentation as an API.
Creating JUnit Tests
You are also to create JUnit tests for at least one of your support classes, either Player or Creature (whichever you would find easier to do).
You do not have to create a complete set of tests for that class, but should create at least one for each of the following:
An inspector method in that class.
A modifier method in that class.
Note that you will also need to create a toString() method for that class in order to test its modifiers.
The Design Document
The initial stage of most object-oriented projects is the creation of a design document that lets developers of a class and its users agree on the methods and constructors the classes will provide. This will be the first stage of this assignment. It is worth 10 of the possible 50 overall points of the assignment.
Specifically, you will turn in a document describing each constructor and method your Player and Creature support classes will provide. To keep things simple, these descriptions will be similar to the JavaDoc documentation you will turn in later (in fact, if you do the design correctly you can just reuse it in your final documentation).
Include the following for each method:
An abstract description of what it does.
What each input parameter represents.
What the return value represents.
Any exceptions it might throw.
This can be turned in as a Word document, a text document, or even an html file created using JavaDoc
package creaturecapture; import java.util.*; public class CreatureCapture { public static void main(String[] args) { Player player; Creature creature; Scanner scanner = new Scanner(System.in); System.out.println("Welcome to creature capture!"); while (/* player still has energy */) { System.out.println(" A new creature has appeared!"); while(/* creature not captured and player still has energy */) { // Tell the player how much energy they have left. System.out.println("You can f) Fire a freeze ray w) Cast a web b) Throw a force bubble"); System.out.print("What is your choice: "); String reply = scanner.nextLine(); switch (reply) { case "f": System.out.print("How much energy do you want to use: "); int amount = Integer.parseInt(scanner.nextLine())
break; case "w": break; case "b;
break; } } } System.out.println("You ran out of energy! Game over!"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
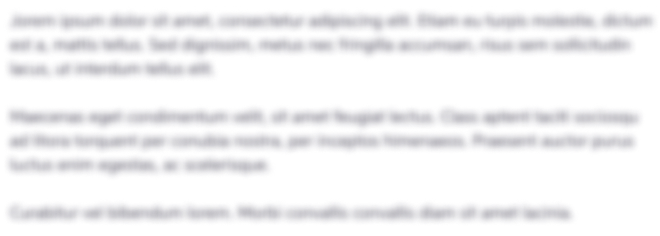
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started