Question
AES program in Java, appreciate any help /** * Implement all of the methods in this class. * In this version, although all values are
AES program in Java, appreciate any help
/**
* Implement all of the methods in this class.
* In this version, although all values are bytes, arrays of ints are used instead of bytes.
* Also, the mixColumns step will be easier to detect whether you need to modulo reduce the polynomials with int
* values (i.e., if a result requires more than 8 bits, modulo reduce with the appropriate XOR).
*/
public class AESOperations {
/**
* Computes the byte substitution step of an AES round.
*
* @param state The current state matrix. This method modifies this matrix by replacing each byte
* with the corresponding output from the AES s-box.
*/
public static void byteSubstitution(int[][] state) {
// HINT 1: I'd recommend simply hardcoding the S-box as a 1 dimensional array of bytes as a field within this class.
// If you use this hint, then you'd convert the 2D S-box to a simple array, by starting with the first row, followed by the 2nd, etc.
// Just Google AES S-box to find it, such as on the wikipedia page. The input byte is then simply used as an index into this array.
//
// HINT 2: You'll probably find the S-box elements specified in hexadecimal. You don't need to convert from hexadecimal to decimal
// in using HINT 1. If you weren't already aware, in Java you can specify integer values with hex, by using 0x. For example, 0x2a
// is how you'd specify the decimal value 42 in hexadecimal.
//
// HINT 3: Reminder, when you initialize an array in Java, you can do so by specifying a list of elements within { }.
// For example, consider the following:
// byte[] someBytes = { 0x2a, 0x33, 0x41 };
// In this example, someBytes is initialized to an array of length 3, with the specific values specified in hexadecimal.
// If you follow HINT 1, you'd have something like this as a field outside this method for the entire S-box.
}
/**
* Computes the shift rows step of an AES round.
*
* @param state The current state matrix. This method modifies this matrix to contain the result of the shift rows step.
*/
public static void shiftRows(int[][] state) {
}
/**
* Computes the mix columns step of an AES round.
*
* @param state The current state matrix. This method modifies this matrix to contain the result of the mix columns step.
*/
public static void mixColumns(int[][] state) {
// HINT 1: See the notes or textbook for the matrix that you need to multiply by state.
// HINT 2: This step is similar to a matrix multiplication, because, well it is. However, the
// byte values actually represent polynomials with coefficients computed mod 2, and with the result mod
// AES's prime polynomial. You might start by implementing a normal matrix multiplication (you'll likely
// need a temporary 2D array for the result, but before this method finishes make sure you copy the result back into state).
// After you implement a normal matrix multiplication, you'll need to change it according to hints 3, 4, and 5.
// HINT 3: Since the elements you're adding are not actually integer values, but rather the integer values
// are encoding the coefficients of a polynomial (mod 2), then addition should actually be done with en XOR.
// Java's XOR operator is ^ so if you followed hint 2, then wherever you are adding, you actually want to XOR.
// HINT 4: For the same reason as in HINT 2, multiplication is not actually a simple multiplication.
// HINT 4a: The one matrix has nothing but 1, 2, and 3 values. If you multiply any value by 1, the result is the value (this is
// no different than if the numbers were integers).
// HINT 4b: If you need to multiply by 2, well the 2 is actually the polynomial: X. You can multiply by 2 in one of
// two ways. You can either left shift 1 position. For example, if you what to compute state[i][j] left shifted
// one position, you would do this: state[i][j] << 1
// You can actually also just multiply by 2 (multiplying by 2 is equivalent to shifting the bit values one place to the left).
// HINT 4c: Wherever you are multiplying by 3, you definitely cannot actually multiply by 3. You will get the wrong answer.
// The value 3 represents the polynomial: X + 1 (since 3 in binary is 11). If you have to compute 3 * state[i][j], then
// this really means (X + 1) * state[i][j], which is equivalent to X * state[i][j] + 1 * state[i][j], equivalent to
// X * state[i][j] + state[i][j]. But from hint 4b, the multiplication by X is a left shift, and from hint 3, the addition
// should be an XOR.
// HINT 5: If any results are polynomials with degree 8 or higher, then you need to modulo reduce by AES's prime polynomial.
// In general computing f(x) mod p(x) may involve multiple rounds of shifting and XOR. However, the multiplications you
// did earlier, represented by 2 and 3 (i.e., X and X + 1) will produce polynomials with degree no greater than 8.
// Why? Well, since each element of the state is a byte, then with 8 bits, each position representing a power of X, the
// left most bit is the X to the power 7 term. If that bit is a 1 and if you multiply by X or by X + 1, then you will end
// up with an X to the power 8 term (but the exponent won't be any higher than that). So, at most a single XOR will be
// needed (and no shifting). So, whenever you shift left, you'll need to first detect whether the left most bit is a 1, and
// if so, after shifting, you'll need to XOR with the value that represents AES's prime polynomial.
// CAUTION: You'll need to detect whether or not the left shift will produce an X to the 8 term before left-shifting.
// Since the state matrix has byte values, the left most bit is lost by the left shift (you only have 8 bits),
// so you need to know what it was before the left shift.
}
/**
* Returns a 2D array from the key.
* @param key The key
* @return 2D array with the key, 4 rows and 4 columns
*/
public static int[][] generateKeyMatrixFromKey(int[] key) {
// HINT: the bytes of the key or filled into the key matrix down the columns (not across rows).
}
/**
* Computes the expanded key matrix for AES.
* @param keyMatrix The first 4 columns, i.e., the initial key matrix.
* @return Returns a new matrix that corresponds to the expanded key matrix of AES.
*/
public static int[][] keyExpansion(int[][] keyMatrix) {
// HINT: The expanded key matrix has 4 rows and 44 columns, so start by constructing a 2D array for the result that
// is of the correct dimensions. Then initialize the first 4 columns with the keyMatrix. Then iterate over the columns
// computing the rest per the AES key expansion rules. Finally return the new 2D array.
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
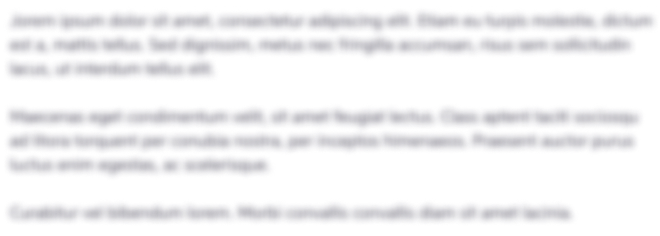
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started