Question
After reading and understanding the Gradient class, start implementing the following classes: 1) Source coed of the Gardient class package app; import grading.*; import java.util.*;
After reading and understanding the Gradient class, start implementing the following classes:
1) Source coed of the Gardient class
package app;
import grading.*;
import java.util.*;
/**
* An application for calculating the numeric grade for
* a course from the grades on individual assignments.
*
* This version assumes that the course is structured as follows:
*
* 6 programming assignments (PAs) accounting for 40% of the course grade
* after the lowest grade is dropped.
*
* 5 homework assignments (HWs) accounting for 10% of the course grade.
*
* 1 mid-term exam (Midterm) accounting for 20% of the course grade.
*
* 1 final exam (Final) accounting for 30% of the course grade.
*
* @version 1.0
* @author Sagacious Media
*
*/
public class Gradient
{
/**
* The entry point for the application.
*
* @param args The 13 grades (ordered as above). Missing grades can be entered as "NA".
*/
public static void main(String[] args)
{
Filter paFilter;
Grade courseGrade, hwGrade, paGrade;
GradingStrategy courseStrategy, hwStrategy, paStrategy;
List
Map
// Early exit
if ((args == null) || (args.length != 13))
{
System.err.println("You must enter all 13 grades. (Use NA for missing.)");
System.exit(1);
}
// Create the filter and strategy for PAs
paFilter = new DropFilter(true, false);
paStrategy = new TotalStrategy();
// Create the strategy for HWs
hwStrategy = new TotalStrategy();
// Create the weights and strategy for the course grade
courseWeights = new HashMap
courseWeights.put("PAs", 0.4);
courseWeights.put("HWs", 0.1);
courseWeights.put("Midterm", 0.2);
courseWeights.put("Final", 0.3);
courseStrategy = new WeightedTotalStrategy(courseWeights);
try
{
// Put the PA grades in a List
pas = new ArrayList
for (int i=0; i<6; i++)
{
pas.add(parseGrade("PA"+(i+1), args[i]));
}
// Calculate the PA grade (after filtering)
paGrade = paStrategy.calculate("PAs", paFilter.apply(pas));
// Put the HW grades in a List
hws = new ArrayList
for (int i=0; i<5; i++)
{
hws.add(parseGrade("HW"+(i+1), args[i+6]));
}
// Calculate the HW grade
hwGrade = hwStrategy.calculate("HWs", hws);
// Put all of the grades in a List
grades = new ArrayList
grades.add(paGrade);
grades.add(hwGrade);
grades.add(parseGrade("Midterm", args[11]));
grades.add(parseGrade("Final", args[12]));
// Calculate the final grade
courseGrade = courseStrategy.calculate("Course Grade", grades);
// Display the final grade
System.out.println(courseGrade.toString());
}
catch (SizeException se)
{
System.out.println("You entered too few valid grades.");
}
catch (IllegalArgumentException iae)
{
// Should never get here since all keys should be valid
}
}
/**
* Construct a Grade object from a key and a String representation of
* its value. If the String representation of the value is null or not a valid
* double then the resulting Grade will have a missing value.
*
* @param key The key for the Grade
* @param value The String representation of the value
* @return The corresponding Grade object
* @throws IllegalArgumentException if the key is invalid
*/
private static Grade parseGrade(String key, String value) throws IllegalArgumentException
{
Grade result;
try
{
Double v;
if (value == null) v = null;
else v = new Double(Double.parseDouble(value));
result = new Grade(key, v);
}
catch (NumberFormatException nfe)
{
result = new Grade(key, null);
}
return result;
}
}
2) Implement the Grade class
the Grade class must satisfy the following specifications.
1. Grade objects must be immutable.
2. If a constructor is passed a key that is null or empty (i.e., "") then the constructor must throw an IllegalArgumentException.
3. The Grade(String key) constructor must construct a Grade object with a value attribute of 0.0.
4. The compareTo(Grade other) method must return the result of comparing this.value and other.value accounting for missing (i.e., null) values appropriately.
4.1. If this.value is null and other.value is non-null then it must return -1.
4.2. If this.value is null and other.value is null then it must return 0.
4.3. If this.value is non-null and other.value is null then it must retun 1.
4.4. If both this.value and other.value are non-null then it must return the result of calling compareTo() on this.value and passing it other.value (though it need not be implemented this way).
5. The toString() method must return a String representation of the Grade object.
5.1. If the value attribute is not null then the String must contain the key attribute, followed by the String literal ":", followed by a single space, followed by the value attribute (in a field of width 5 with 1 digit to the right of the decimal point).
5.2. If the value attribute is null then the String must contain the key attribute, followed by by the String literal ":", followed by a single space, followed by the String literal " NA" (which is also a field of width 5).
Note that, while null key attributes are invalid (i.e., every Grade object must have a non-null, non-empty key attribute), null value attributes are valid (and are used to indicate that the Grade is missing).
3) Implement the Missing class
the Missing class must satisfy the following specifications.
1. The default double value of a missing value must be 0.0.
2. The method doubleValue(Double number) must return the double value of the Double parameter unless it is null, in which case it must return the default double value of a missing value.
3. The method doubleValue(Double number, double missingValue) must return the double value of the Double parameter unless it is null, in which case it must return missingValue.
4) Implement the SizeException class
the SizeException class must satisfy the following specifications.
1. The SizeException class must be a checked exception.
5) Implement the GradingStrategy interface
Calculate(key: string, grades : java.until.List
6) Implement the TotalStrategy class
the TotalStrategy class must satisfy the following specifications.
1. public methods must not have any side effects. That is, they must not change the parameters that they are passed in any way (e.g., the List that is passed to the calculate() method must not be changed in any way) and they must not change attributes that are not owned (i.e., attributes that are aliases) in any way.
2. The calculate() method must calculate the total of the List of Grade objects it is passed.
2.1. You may assume that the calculate() method is passed a List that does not contain any null elements.
2.2. If the List is null then it must throw a SizeException.
2.3. If the List is empty then it must throw a SizeException.
2.4. Otherwise, it must return a Grade object with the given key and a value equal to the total of the Grade objects in the List.
2.4.1. If the value of a particular Grade is missing (i.e., null) then a value of 0.0 must be used. Note: The Missing class has a method that can be used to accomplish this.
7) Implement the WeightedTotalStrategy
the WeightedTotalStrategy class must satisfy the following specifications.
1. public methods must not have any side effects. That is, they must not change the parameters that they are passed in any way (e.g., the List that is passed to the calculate() method must not be changed in any way) and they must not change attributes that are not owned (i.e., attributes that are aliases) in any way (e.g., the Map that is passed to the constructor must not be changed in any way).
2. The calculate() method must calculate the weighted total of the List of Grade objects it is passed.
2.1. You may assume that the calculate() method is passed a List that does not contain any null elements.
2.2. If the List is null then it must throw a SizeException.
2.3. If the List is empty then it must throw a SizeException.
2.4. Otherwise, it must return a Grade object with the given key and a value equal to the weighted total of the Grade objects in the List.
2.4.1. The weight for each element must be obtained from the Map using the key for that element.
2.4.1.1. If the weights Map is null than a weight of 1.0 must be used.
2.4.1.2. If the weight for a particular Grade is unspecified (i.e., null) then a weight of 1.0 must be used. Note: The Missing class has a method that can be used to accomplish this.
2.4.1.3. If the weight for a particular Grade is less than 0.0 then a weight of 0.0 must be used.
2.4.2. If the value of a particular Grade is missing (i.e., null) then a value of 0.0 must be used. Note: The Missing class has a method that can be used to accomplish this.
3. The default constructor must (directly or indirectly) initialize the weights Map to null.
8) Implement the Filter interface.
+apply(grades : java.until.List
9) Implement the DropFilter class.
the DropFilter class must satisfy the following specifications.
1. public methods must not have any side effects. That is, they must not change the parameters that they are passed in any way (e.g., the List that is passed to the apply() method must not be changed in any way) and they must not change attributes that are not owned (i.e., attributes that are aliases) in any way.
2. You may assume that the apply() method is passed a List that does not contain any null elements.
3. The default constructor must construct a DropFilter object that drops the lowest and highest element.
4. The apply() method must construct a new List that is a subset of the List it is passed.
4.1. If the apply() method is passed a List that has an inappropriate size then it must throw a SizeException.
4.1.1. If the apply() method is passed a null List then it must throw a SizeException.
4.1.2. If the apply() method is passed a List that contains fewer elements than are to be dropped then it must throw a SizeException.
4.1.3. If the apply() method is passed a List that contains the same number of elements as are to be dropped then it must throw a SizeException.
4.2. If the apply() method is passed a list that has an appropriate size then it must return a new List.
4.2.1. The elements of the new List must be aliases for (not copies of) the Grade objects in the List it is passed.
4.2.2. Because each Grade object in the List has a key that can be used to identify it, the new List need not be in the same order as the List it is passed.
4.2.3. The elements in (and size) of the returned List must be based on the values of the parameters that were passed to the constructor when the object was constructed.
4.2.3.1. If shouldDropLowest was true then it must drop exactly one of the elements with the lowest value in the original List.
4.2.3.2. If shouldDropHighest was true then it must drop exactly one of the elements with the highest value in the original List.
4.2.3.3. When dropping the highest and lowest, two elements must always be dropped, even if the highest and lowest have the same value.
4.2.3.4. When determining the highest and/or lowest values it must account for missing (i.e., null) values as in the compareTo() method of the Grade class (i.e., missing values have smaller magnitude than non-missing values and one missing value has the same magnitude as another missing value).
Step by Step Solution
There are 3 Steps involved in it
Step: 1
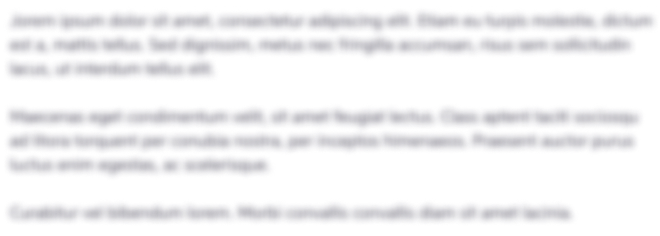
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started