Question
Aims Develop a Python program to solve a problem Follow good program development and coding style practices Use functions and files to allow more complex
Aims
Develop a Python program to solve a problem
Follow good program development and coding style practices
Use functions and files to allow more complex solutions
Instructions
Write a Python program to solve the following problem. Your solution should include a readme.md file (which includes details of what your program does, how to install it, how to run it and how its efficiency could be improved) and your Python program in a file named top_n.py, and be submitted as a single .tgz file named pt2.tgz. You should ensure your solution works using the Python 3 interpreter on turing.
Problem
Thanks to your donations.py program, Save the Northern Hairy-nosed Wombat (SNHW) now has an idea of which sponsors have donated how much to their cause. The SNHW CEO now wants you to develop a program called SponsorFind which allows staff to quickly access the information for a particular sponsor, and/or list the top n sponsors based on total donations.
The data are stored in a file named sponsors.txt, with each line containing a name of a sponsor, followed by a comma (,), followed by their total amount donated. The name must not be an empty string, and the total amount donated must be a positive integer or a positive floating point number with at most two decimal places. Any line that does not meet this format exactly should be seen as an error. Further, each sponsor should only be listed in the file once. More than one line corresponding to the same sponsor should also be seen as an error.
The line below shows a valid line in sponsors.txt:
John Smith,105.5
Your task is to read in the data from sponsors.txt and, if there are no errors reading the data, ask the user what they would like to do. The choices users have consist of searching for sponsors using a search string, asking for a list of top n sponsors, based on total donations, and exiting. The user should be taken to the main menu again and again, until they choose to exit. If a user enters a wrong input, they should be advised that they entered invalid data and taken back to the main menu.
If a user is searching for a sponsor, they are prompted for a search string, which must be non-empty. If they are asking for a list of top n sponsors, they are prompted for the number n of sponsors that they want to list, where n should be a non-negative integer. For a valid n, your program should output the top n sponsors and their total donation amount in descending order. If n is an integer greater than the total number of sponsors, the program should output all the sponsors.
To extract the data from sponsors.txt, your program should have a function named read_sponsor_data that takes a single parameter that is the name of the file to read the data from. The function should return a dictionary, where each key is a sponsor name, and the corresponding value is the total donations for that sponsor, if the data read from sponsors.txt is valid. If there are any errors reading data from the file, this function should raise an exception, which your main program should then handle by printing a message for the user and terminating (see Example Interactions).
You should also have a function named top_n_sponsors that takes as parameters a dictionary of sponsors/total donations pairs returned by read_sponsor_data, and a number n. This function should return a list of n tuples, each containing a single sponsor name and their total donations, sorted by the total donations in descending order.
Note:
Your solution must include the two functions specified above, and can include other functions if you would like.
All total donation amounts that you output to the console should be formatted to two decimal places.
The example interactions below give you an idea of how your program should respond to unexpected input, but the onus is on you to predict what other unexpected inputs and errors your program may encounter, and respond to them in an appropriate way. E.g. what happens if the sponsors.txt file does not exist or is unreadable? What about if there are two sponsors who have donated the same amount? Does your program handle cases like that in a way that is useful and informative to the user?
Once you finish the assignment, you should reflect on it and consider if another way of solving this problem may be more efficient in some cases. You should make a recommendation for improving efficiency in the readme.md file.
Example Interactions
Given the following contents in sponsors.txt:
John Smith,230.5 Sally Jones,380 Mary Smith,104.55 David McDougal,165.7 Sally Matthews,184.5 Peter Evans,300.25
here is an example of interactions:
Welcome to SponsorFind! 1. Search for a sponsor. 2. List top n sponsors. 3. Exit What would you like to do? (Enter 1, 2 or 3): 1 Please enter a search string: Smith Search returned 2 results: John Smith has donated $230.50 Mary Smith has donated $104.55 What would you like to do? (Enter 1, 2 or 3): 1 Please enter a search string: Search string cannot be empty. Please enter a search string: Rodriguez Search returned no results. What would you like to do? (Enter 1, 2 or 3): 2 How many sponsors would you like to list? 3 The top 3 sponsors and their donations are: 1. Sally Jones ($380.00) 2. Peter Evans ($300.25) 3. John Smith ($230.50) What would you like to do? (Enter 1, 2 or 3): 2 How many sponsors would you like to list? Hello Invalid value. Non-negative integer required. How many sponsors would you like to list? 7 The top 6 sponsors and their donations are: 1. Sally Jones ($380.00) 2. Peter Evans ($300.25) 3. John Smith ($230.50) 4. Sally Matthews ($184.50) 5. David McDougal ($165.70) 6. Mary Smith ($104.55) What would you like to do? (Enter 1, 2 or 3): 2 How many sponsors would you like to list? -3 Invalid value. Please enter a non-negative integer. How many sponsors would you like to list? 0 The top 0 sponsors and their donations are: What would you like to do? (enter 1, 2 or 3): 3 Goodbye!
*****************************************************************
Given any of the following contents of sponsors.txt:
,300.0
or:
John Smith 100
or:
Samantha Tilley,200.004
or:
Peter Matthews,100 Peter Matthews,100
an interaction with the program should look like the following:
Error reading data. Each line of sponsors.txt should contain a sponsor name, followed by a comma, followed by a total amount donated, and no sponsor should appear more than once in the file.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
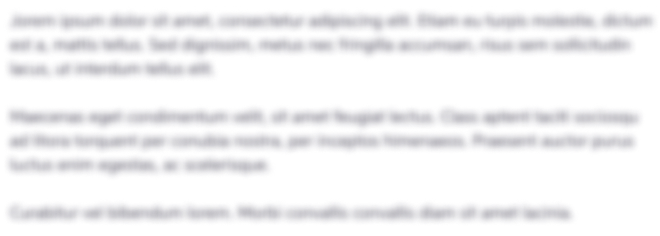
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started