Question
AJAX, JSON, and Table Rows Add Rows to a Table Introduction In this assignment you will use AJAX to read in an external JSON data
Add Rows to a Table
Introduction
In this assignment you will use AJAX to read in an external JSON data file. You will convert this external JSON data file into a JavaScript object and then display the contents in a web page as new rows in an existing table.
Student Information from JSON file
Loading student data...
Name | Favorite Hobby | Favorite Color |
---|
Add this CSS to your "css/styles.css" file:
body { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/tri.svg') top center no-repeat; background-size: cover; color: #555; font-family: Arial,Helvetica,sans-serif; } h1, h2, h3 { font-family: Arial, Helvetica, sans-serif; } h2 { border-bottom: 0.02em solid #3f87a6; color: #3f87a6; } .loading { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/ajax-loader_trans.gif') center left no-repeat; color: #94aace; font-size: smaller; font-style: italic; padding-left: 20px; } .loadingHidden { display: none; } main { width: 100%; min-height: 60vh; max-width: 40em; background: white; box-shadow: 0 0 2em #aaa; padding: 2em 5% 3em 5%; margin: 0 ; display: flex-container; } section { margin-bottom: 2em; } .student-info p { background: transparent url('https://chelan.highline.edu/~tpollard/assets/images/user_grayscale.png') center left no-repeat; padding-left: 20px; } table { border-collapse: collapse; min-width: 90%; } tbody tr:nth-child(odd) { background-color: #eff7fb; } td, th { text-align: left; padding-left: .3em; padding-right: .3em; } tr { border-bottom: 1px solid #ccc; } @media (min-width: 45em) { main { width: 80%; padding: 2em 5% 6em 5%; margin: 4em ; } }
Like the previous assignments, you should use "window.onload" to wait until the HTML page is loaded before any of your other JavaScript is run: Waiting for the Page to Load & Event Listeners
At the beginning of your "js/scripts.js" file you should do something like this:
// Wait until the page loads. window.onload= function(){ // Read JSON data and display to page. processData(); };
The processData function calls theajaxLoadFilefunction. A copy of theajaxLoadFilefunction to use is listed at the bottom of the assignment. You can use this function as it is. You don't need to edit it at all.
We pass in 2 arguments to theajaxLoadFilefunction:
the path to the file "data/students.json",
and the callback function to run after the file was successfully read from the server.
The callback function then converts the JSON string into a JavaScript object, and then passes that object to the displayData function:
// Read the student JSON string, convert it to a JavaScript object. function processData() { ajaxLoadFile('data/students.json', function(response) { // Convert JSON string into JavaScript Object. let jsResponse = JSON.parse(response); // Display the results displayData(jsResponse) ; }); }
This displayData function needs to do these things:
Create a DOM fragment.
Loop through the "students" object.
Read thefirst name,last name,favorite hobby,andfavorite colorfrom each object.
Create a table row "tr" element.
Create a table data "td" element for each column in the row.
Add the student's name to the first column of the row usingcreateTextNode,template literals, andappendChild. The first column should contain both the student's first and last name in this format - "lastname, firstname" - then append the td to the tr element.
Add the student's hobby in the second column, then append it to the tr element.
Add the student's favorite color in the third column, then append it to the tr element.
Add the tr to a DOM fragment
Finally, after you've built your DOM fragment append it the "#student-table tbody" element.
Use querySelector to read the "p.loading" paragraph.
Change the class attribute of that paragraph from 'loading' to 'loadingHidden' using 'classList.replace': Setting CSS Styles Using JavaScript
// Display the student data. function displayData(students) { // Add the student data to the DOM. // Hide the loading message. }
Add the following JavaScript function to the end of your "js/scripts.js" file. You will use this function to read the JSON file from the server. Take some time to read through this code to understand how it works: Anatomy of an AJAX Request. You should be able to just use this function. You don't need to edit it at all!
/** * Description: Loads a file from the web server. Runs a callback function if sucessfull. * Parameters: filename The filename to load. * callback The callback function to run after the file has been loaded. */ function ajaxLoadFile(filename, callback) { // Create new request. let xobj = new XMLHttpRequest(); // Add datetime parameter to the URL to prevent browser caching. let bustCache = '?datetime=' + new Date().getTime(); xobj.open('GET', filename + bustCache, true); xobj.onreadystatechange = function() { // 4 == DONE, 200 == request was fulfilled. if (xobj.readyState == 4 && xobj.status == "200") { // File was loaded so run callback function. callback(xobj.responseText); } } xobj.send(null); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
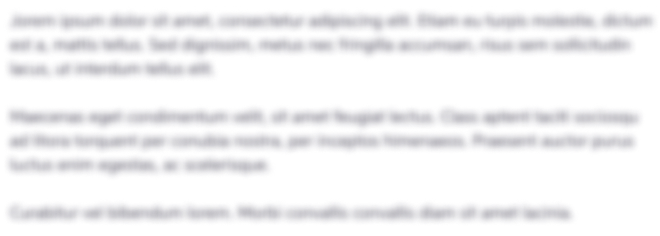
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started