Question
Algorithms: BFS/Python implementation-- Need help fixing code for bfs babyfaces vs heels program below . My code below runs but it's output is not 100%
Algorithms: BFS/Python implementation-- Need help fixing code for bfs "babyfaces vs heels" program below.
My code below runs but it's output is not 100% correct, and I need help correcting it. It MUST be able to run on flip terminal using python OR python3.
Program Requirements: Suppose there are two types of professional wrestlers: Babyfaces (good guys) and Heels (bad guys). Between any pair of professional wrestlers, there may or may not be a rivalry. Suppose we have n wrestlers and we have a list of r pairs of rivalries.
Input: Input is read in from a file specified in the command line at run time. The file contains the number of wrestlers, n, followed by their names, the number of rivalries r and rivalries listed in pairs. Note: The file only contains one list of rivalries
Output: Results are outputted to the terminal.
---Yes, if possible followed by a list of the Babyface wrestlers and a list of the Heels.
---No, if impossible.
EXAMPLE INPUT:
6 Bear Maxxx Killer Knight Duke Samson 5 Bear Samson Killer Bear Samson Duke Killer Duke Maxxx Knight
// OUTPUT REQ'D Yes Possible Babyfaces: Bear Maxx Duke //currently, my code has Maxx in the "Heels" category. Heels: Killer Knight Samson
***************************** CODE **********************************
import sys
import os
from pprint import pprint
def designate_wrestlers(wrestlers):
def rival_type(type):
if type == 'babyface':
return 'heel'
return 'babyface'
def mark_rivals(node):
good_guys = wrestlers[node]['type']
bad_guys = rival_type(good_guys)
for r in wrestlers[node]['rivals']:
# derive the rival type for this node.
current_type = wrestlers[r]['type']
if current_type is not None and current_type != bad_guys:
print("NO! Wrestlers have conflicting alignments. Sides cannot be allocated to these wrestlers!")
sys.exit()
else:
wrestlers[r]['type'] = bad_guys
# starting node = babyface
# populate the unvisited nodes list with the neighbors
node_list = [x for x in list(wrestlers.keys()) if wrestlers[x]['state'] != 'visited']
while len(node_list):
n = node_list[0]
wrestlers[n]['type'] = 'babyface'
wrestlers[n]['state'] = 'visited'
unvisited_nodes = list(wrestlers[n]['rivals'])
mark_rivals(n)
while unvisited_nodes:
u = unvisited_nodes.pop(0) # take the first item in the list to use BFS
wrestlers[u]['state'] = 'visited'
# add any unchecked neighbors to unvisited, remove dupes
for r in wrestlers[u]['rivals']:
if wrestlers[r]['state'] != 'visited':
unvisited_nodes.append(r)
unvisited = list(set(unvisited_nodes))
# mark all of their neighbors
mark_rivals(u)
node_list = [x for x in list(wrestlers.keys()) if wrestlers[x]['state'] != 'visited']
def print_allegiances(wrestlers):
print(" ")
print("YES! We've designated the rivalries between these wrestlers:")
print("\tBabyfaces: " + str(' '.join([x for x in list(wrestlers.keys()) if wrestlers[x]['type'] == 'babyface'])))
print("\tHeels: " + str(' '.join([x for x in list(wrestlers.keys()) if wrestlers[x]['type'] == 'heel'])))
print(" ")
def main():
# get and read filename
try:
file_name = sys.argv[1]
except IndexError:
print("You must specify a valid filepath for the designate wrestlers script.")
sys.exit()
assert os.path.exists(file_name), "File not found. Please specify a valid file path."
with open(file_name, "r") as w_file:
lines = w_file.readlines()[1:] # skip first line
# loop through the wrestlers in the file and add them to the list of wrestlers.
lines = [x.strip() for x in lines if not x.startswith("// ")]
# parse through the lines until we hit a line where its an integer representing the rivalries
wrestlers = {}
l = lines.pop(0)
wrestlers_parsed = False
while not wrestlers_parsed:
try:
l = int(l)
except ValueError:
wrestlers[l] = {
'rivals': [],
'type': None,
'state': 'unchecked'
}
l = lines.pop(0)
else:
wrestlers_parsed = True
# parse through the rivalries and populate those into the wrestlers list
r = lines.pop(0)
while lines:
r = lines.pop(0)
if len(r.split(" ")) != 2:
break
w1, w2 = r.split(" ")
if w2 not in wrestlers[w1]['rivals']:
wrestlers[w1]['rivals'].append(w2)
if w1 not in wrestlers[w2]['rivals']:
wrestlers[w2]['rivals'].append(w1)
# invoke the designate_wrestlers algo
# pprint(wrestlers)
designate_wrestlers(wrestlers)
print_allegiances(wrestlers)
if __name__ == '__main__':
main()
Step by Step Solution
There are 3 Steps involved in it
Step: 1
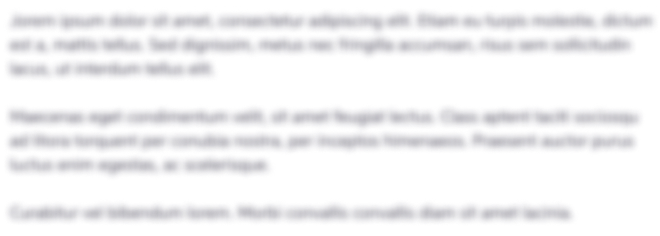
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started