Question
ALL IN C++ Question: 1. Download the input file, employees.dat, from my public directory. While you are in your own cs... 1. Download the input
ALL IN C++
Question: 1. Download the input file, employees.dat, from my public directory. While you are in your own cs...
1. Download the input file, employees.dat, from my public directory. While you are in your own cs111
directory, use the copy command (cp).
[smith001@empress cs111] cp /cs/slott/cs111/employees.dat . Copying into the current directory
Assume this input file contains an unknown number of lines/employees. Each line contains the information about an employee - first name, last name, gender, hourly rate, employee id and age. Assume the number of lines/employees never exceeds 100.
Ada Agusta F 10.00 19569 28
Issac Asimov M 18.25 63948 58
:
:
2. Create a struct called employee.
3. In the main, create 2 arrays of the struct employee type called mAr and fAr. mAr will have all the male
employees and fAr will have all the female employees.
use const for the size(100) for each array.
Make a function called readData that will fill mAr and fAr from the input file. This function should return the number of male employees and also the number of female employees to the caller. Assume you dont know how many employees you have (use the while loop). Declare the input file and open and close it within this function.
hint:
??? readData( ??? )
{
mi = 0; //the index to the male array
fi = 0; //the index to the female array
declare the input file open the input file if it doesnt exist, display an error message and terminate the program if it exists, read each employee from the file and store it into the appropriate array depending on the gender. Keep counting how many male and female employees you have.
employee temp; //make an employee called temp //fill temp with the first employee fin >> temp.fName; fin >> temp.lName;
fin >> temp.gender;
:
while(fin && there is still room for both female and male arrays)
{
if(temp.gender == F) fAr[???] = temp; //copy all the fields from temp to the female array
:
read in the next employee into temp }
close the input file.
}
5. Call readData() in the main and display the numbers of male and female employees. Run your program now and make sure you get 11 for males and 5 for females.
Sample Run There are 11 male and 5 female employees.
Make a function called printEmployee. One employee (not the whole array) will be passed to this function as a parameter and display all the information about that employee on the screen in the following format. There should be only one parameter.
Sample Run
Ada Agusta F 10.00 19569 28
7. Call printEmployee with the first employee in the female array in the main. Run your program and make sure you will see the correct information on the screen.
Expected output
Ada Agusta F 10.00 19569 28
8. Call printEmployee with the first employee in the male array in the main. Run your program and make sure you will see the correct information on the screen. Expected output Issac Asimov M 18.25 63948 58
9. Make a function called printAllEmp. Either the male or female array will be passed to this function. This function will print all the employees in the array to the screen in the following format. Call the printEmployee function(#6) to print each employee. this function should not have a cout.
Dont go through the entire array. Pass the number of employees returned from readData() to this function and check only the slots being occupied
for(int i = 0; i < ????; i++)
printEmployee(........);
Ada Agusta F 10.00 19569 28
Emmylou Harris F 33.50 72647 38
:
:
Call printAllEmp with the male array in the main. Make sure you see all the 11 male employees on the screen.
Call printAllEmp with the female array in the main. Make sure you see all the 5 female employees on the screen.
12. Make a function called outfileArray. To this function, you will pass either the male or female array. It will output the last and first names of all the employees from the array to the output file the user specifies in the following format. Declare, Open and close the output file within this function.
Sample Run Enter the output file name: male.dat
male.dat Asimov, Issac Bogart, Humphry
:
:
13. Test outfileArray with the male array. Make sure your output file has all the male employees.
14. Test outfileArray with the female array. Make sure your output file has all the female employees.
15. Make a function called findOldest. To this function, you will pass either the male or female array. This function will find the oldest employee in the array and return the whole struct to the caller. Assume there is only one employee with that oldest age.
Hints: employee findOldest(.....)
{ look for the oldest
return ?????; //return the whole struct }
16. Call findOldest with the male array in the main and display only the first name of the oldest employee (use cout in the main).
Sample Run
Albert
Added Wed (Nov 16)
17. Make a function called giveRaise. A raise percentage is passed to this function as one of the parameters and give the raise to everybody in the array. You will be passing either the male or female array to this function.
18. Call giveRaise in the main with the female array and 5.5 (5.5% raise) and then call printAllEmp to make sure all the female employees received a 5.5 raise.
int main()
{
giveRaise(5.5, ?????????????); }
Expected Output Ada Agusta F 10.55 19569 28
Emmylou Harris F 35.34 72647 38
:
:
19. Call giveRaise in the main with the male array and 5.0 (5.0% raise) and then call printAllEmp to make sure all the male employees received a 5.0 raise.
Expected Output
Issac Asimov M 19.16 63948 58
:
:
20. Make a function called giveRaiseToOneEmployee. One employee (do not pass an entire array) and a raise percentage will be passed to this function. It will give the raise to the employee. Hint:
21. Call giveRaiseToOneEmployee in the main passing the second employee in the female array and 2.0 (2.0% raise). Then call printEmployee with the same employee.
int main()
{
giveRaiseToOneEmployee(2.0, ??????);
}
Expected Output Emmylou Harris F 36.05 72647 38
22. Call giveRaiseToOneEmployee in the main passing the first employee in the male array and 50.0 (50.0% raise). Then call printEmployee with the same employee.
Expected Output Issac Asimov M 28.74 63948 58
23. Make a function called combineArray that will combine the male and female arrays into one array.
a) Create a new array called allEmp with 200 slots in the main. Use SIZE * 2 = 200. (We could have 100 males and 100 females, so the combined array should have 200 slots.)
?????? allEmp[SIZE*2]; b) The combineArray function should copy all the males from the male array into the allEmp array first
then copy all the females from the female array into the allEmp array.
24. Call printAllEmp with the allEmp array and make sure you have all the males then females.
25. Make a void fucntion called findEmp that will find the employee with a particular id.
Your program should then output to the screen the first name and last name of that employee. If the employee id doesn't exist, an appropriate message
should be given (See Sample Run below). Just ask the user to enter an employee id once, and you dont have to keep asking using a loop. Employee ids are unique, so don't keep looking after you found the employee. You should use a boolean variable. Initialize it to false and set it to true when you find the employee.
void findEmp(???????????) {
Ask the user to enter an employee id.
bool found = false; //set it to true as soon as the id is found e.g. key = 2000 Found Dont stay in the for loop and check the rest
for(???????????????????) {
}
//Dont forget to say There is no employee with ID ....
} 26. Call findEmp () with the allEmp array twice in the main.
To this function, youcould pass any Employee array (allEmp, male or female), so give your parameter for the array a generic name.
Inside this function, you will ask the user to enter an employee id.
Sample Run
Enter the employee id: 48482 Humphry Bogart Enter the employee id: 11111 There is no employee with ID 11111.
First call to findEmp()
Second call to findEmp()
This is the last function. You might want to wait to make it until I go over c-strings in lecture on Monday.
27. Make a function called sortEmployees that will sort an Employee array by first name in alphabetical order (ascending order) using the selection sort
You can compare C++ strings using relational perators (>, <, ==, etc).
string name1, name2; cout << Name 1: ; cin >> name1; cout << Name 2: ; cin >> name2;
if(name1 < name2) //name2 is bigger than name1aname2 comes after name1 in a dictionary cout << name1 << shows up before << name2 << in the dictionary. << endl;
else if(name2 < name1) //name1 is bigger than name2aname1 comes after name2 in a dictionary cout << name2 << shows up before << name1 << in the dictionary. << endl;
else // name1 == name2 cout << The two names are the same. << endl;
Sample Run 1: Name 1: Agusta Name 2: Einstein Agusta shows up before Einstein in the dictionary. From if
Sample Run 2: Name 1: Einstein Name 2: Agusta Agusta shows up before Einstein in the dictionary. From else if
Sample Run 3: Name 1: Einstein Name 2: Einstein The two names are the same. From else
Recall you can do an aggregate operation to copy a whole struct to another. You do not need to copy data field by field.
To this function, you could pass any Employee array (allEmp, male or female), so give your parameter for the
array a generic name.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
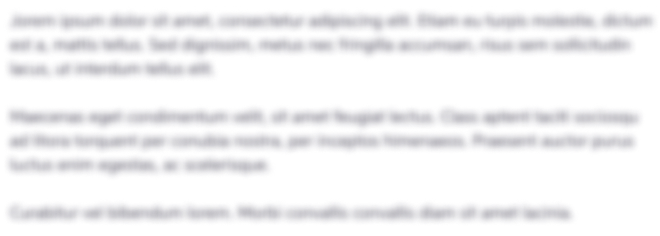
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started