All this is written in Java
This is a Two-part question please follow the instructions and make sure the program follows the rubric as well.
Please make sure that when you answer you have all the new programs separate ex. ElectionTesterV5, ElectionTesterV6, ElectionTesterV7, ElectionTesterV8 Do not worry about the PMR in the Rubric.
Part 1:
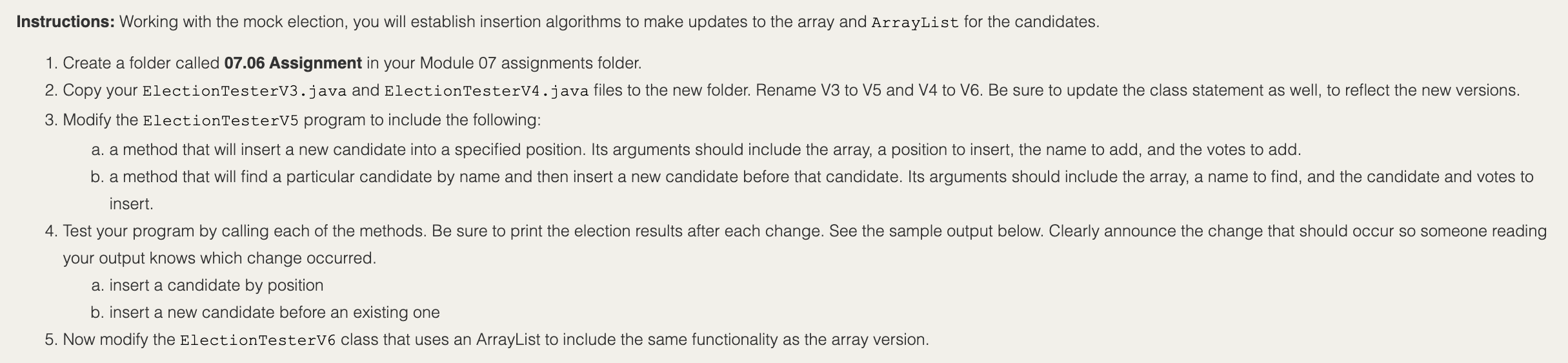
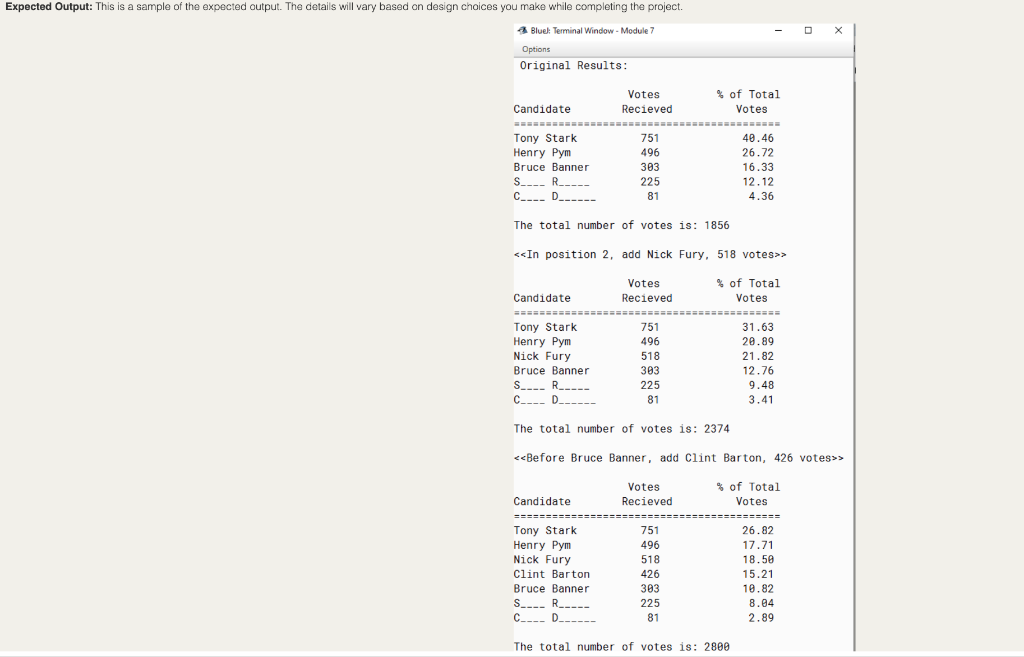
Part 2:
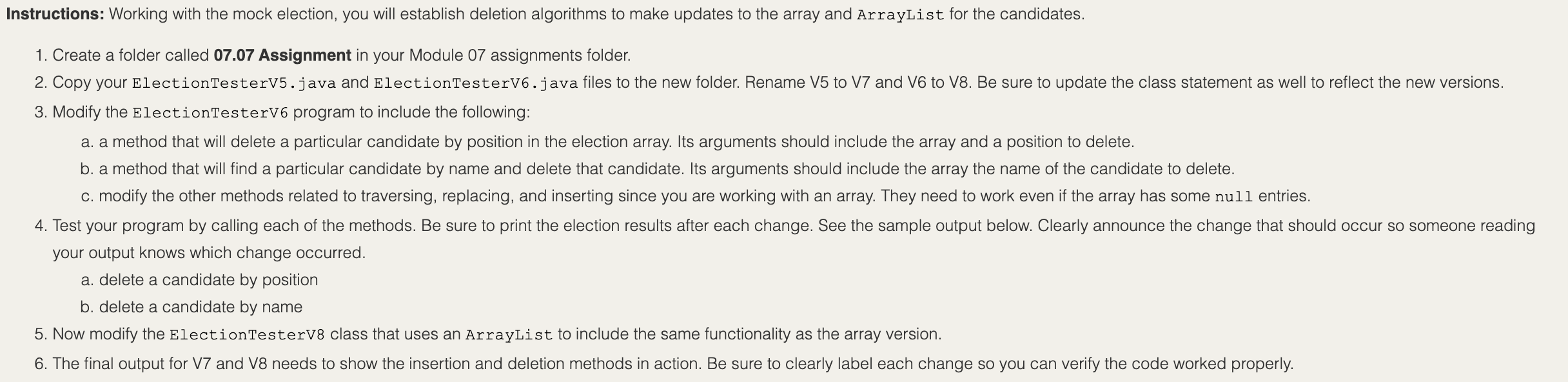
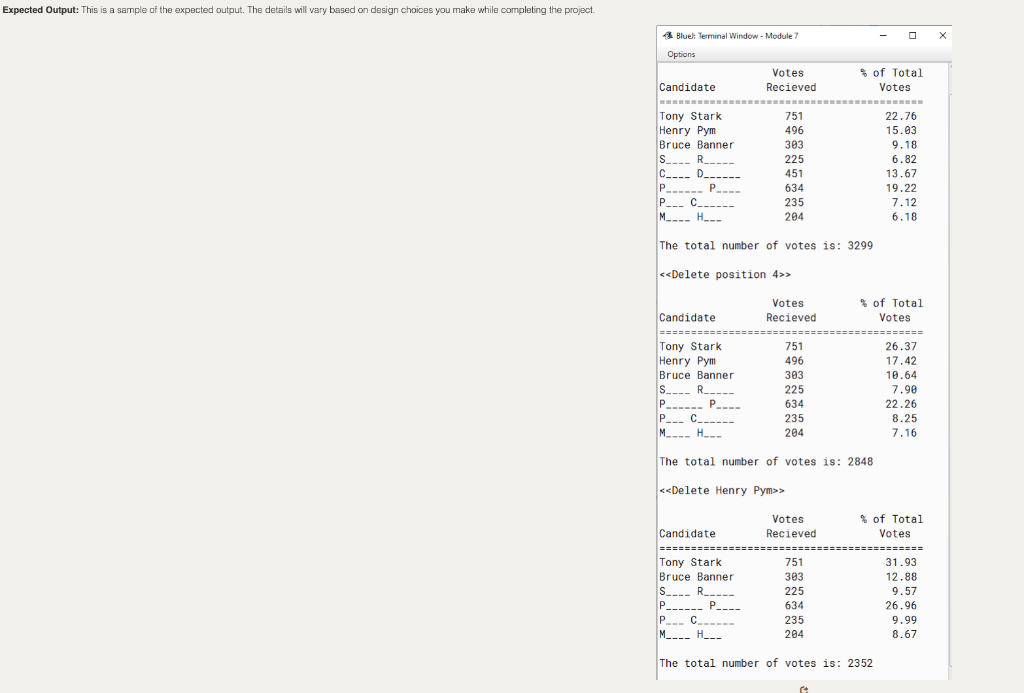
Rubric
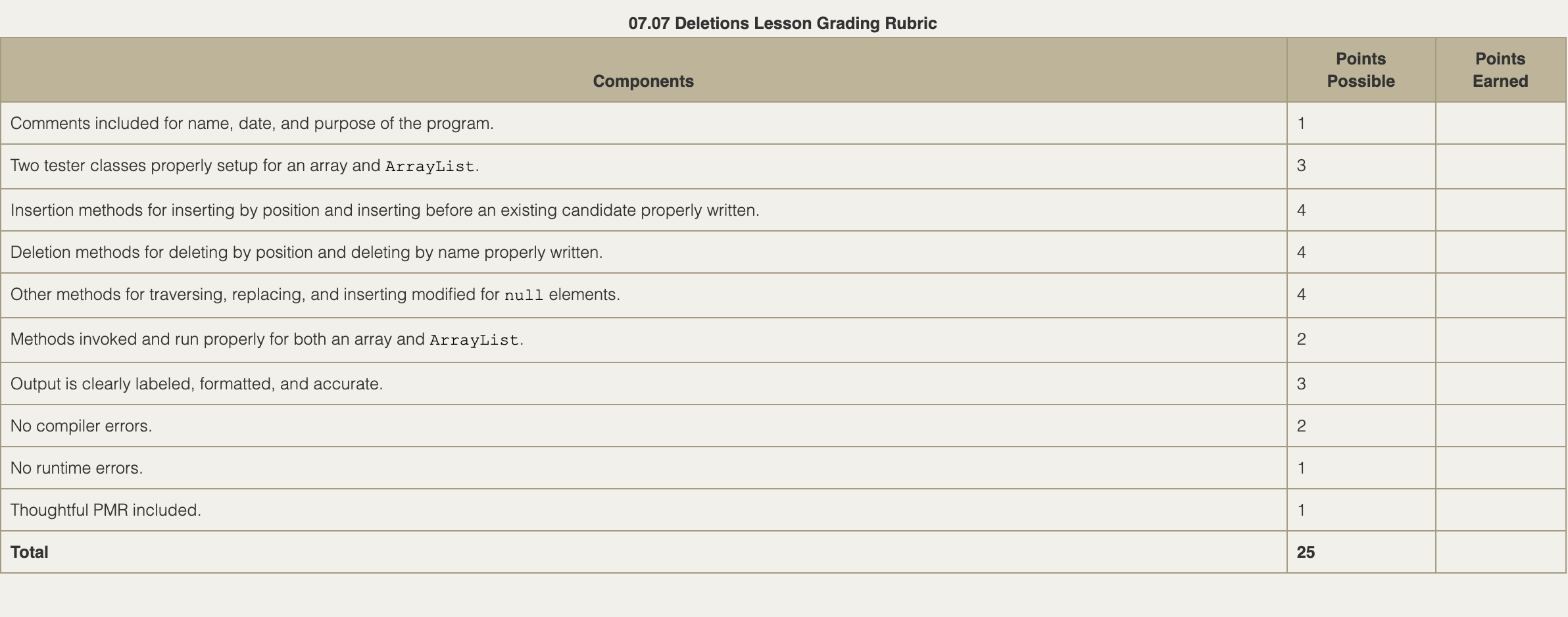
ElectionTesterV3:
public class ElectionTesterV3{
public static void printCandidates(Candidate[] arr){ for(Candidate c: arr){ System.out.println(c); } }
public static int countTotalVotes(Candidate[] arr){ int totalVotes = 0; for(Candidate c:arr){ totalVotes += c.getVotes(); } return totalVotes; }
public static void printTable(Candidate[] arr){ System.out.println(); System.out.format("%-20s%-20s%-20s","candidate","Votes Recieved","% of total votes"); System.out.println(" ===========================================================");
int totalVotes = countTotalVotes(arr); for(Candidate c: arr){ System.out.format("%-25s%-20s%-20.2f",c.getName(),c.getVotes(),(double)c.getVotes()*100/totalVotes); System.out.println();
}
System.out.println(" The total number of votes is: "+totalVotes); }
// method to change name public static void changeName(Candidate[] arr, String name, String newName){ // traverse through index and if the name found set its name to new name by setter of candidate for(int i=0;i // method to change votes, same as above public static void changeVotes(Candidate[] arr, String name, int newVote){ for(int i=0;i // teseter for this public static void main(String [] args){
// make arr of 5 and assign values Candidate arr[] = new Candidate[5];
arr[0] = new Candidate("Tony Stark",751); arr[1] = new Candidate("Henry Pym",496); arr[2] = new Candidate("Bruce Banner",303); arr[3] = new Candidate("S____ R____",225); arr[4] = new Candidate("C____ D_____",81);
// print original result System.out.println(" Original results: "); printTable(arr);
// change name and print results System.out.println(">"); changeName(arr,"Bruce Banner","Stan Lee");
printTable(arr);
// change votes and print results System.out.println(">"); changeVotes(arr,"Henry Pym",284);
printTable(arr);
// change name and vote together and print results System.out.println(">"); changeNameAndVote(arr,"Tony Stark","David John",643);
printTable(arr);
} }
ElectionTesterV4
import java.util.*;
public class ElectionTesterV4{
public static void printCandidates(ArrayList list){ for(Candidate c: list){ System.out.println(c); } }
public static int countTotalVotes(ArrayList list){ int totalVotes = 0; for(Candidate c:list){ totalVotes += c.getVotes(); } return totalVotes; }
public static void printTable(ArrayList list){ System.out.println(); System.out.format("%-20s%-20s%-20s","candidate","Votes Recieved","% of total votes"); System.out.println(" ===========================================================");
int totalVotes = countTotalVotes(list); for(Candidate c: list){ System.out.format("%-25s%-20s%-20.2f",c.getName(),c.getVotes(),(double)c.getVotes()*100/totalVotes); System.out.println();
}
System.out.println(" The total number of votes is: "+totalVotes); }
public static void changeName(ArrayList list, String name, String newName){ for(int i=0;i public static void changeVotes(ArrayList list, String name, int newVote){ for(int i=0;i public static void changeNameAndVote(ArrayList list, String name, String newName, int newVote){ for(int i=0;i public static void main(String [] args){
ArrayList list = new ArrayList(5);
list.add(new Candidate("Tony Stark",751)); list.add(new Candidate("Henry Pym",496)); list.add(new Candidate("Bruce Banner",303)); list.add(new Candidate("S____ R____",225)); list.add(new Candidate("C____ D_____",81));
System.out.println(" Original results: "); printTable(list);
System.out.println(">"); changeName(list,"Bruce Banner","Stan Lee");
printTable(list);
System.out.println(">"); changeVotes(list,"Henry Pym",284);
printTable(list);
System.out.println(">"); changeNameAndVote(list,"Tony Stark","David John",643);
printTable(list);
} }
Instructions: Working with the mock election, you will establish insertion algorithms to make updates to the array and ArrayList for the candidates. 1. Create a folder called 07.06 Assignment in your Module 07 assignments folder. 2. Copy your ElectionTesterv3.java and ElectionTester14.java files to the new folder. Rename V3 to V5 and V4 to V6. Be sure to update the class statement as well, to reflect the new versions. 3. Modify the ElectionTesterv5 program to include the following: a. a method that will insert a new candidate into a specified position. Its arguments should include the array, a position to insert, the name to add, and the votes to add. b. a method that will find a particular candidate by name and then insert a new candidate before that candidate. Its arguments should include the array, a name to find, and the candidate and votes to insert. 4. Test your program by calling each of the methods. Be sure to print the election results after each change. See the sample output below. Clearly announce the change that should occur so someone reading your output knows which change occurred. a. insert a candidate by position b. insert a new candidate before an existing one 5. Now modify the ElectionTesterv6 class that uses an ArrayList to include the same functionality as the array version. Expected Output: This is a sample of the expected output. The details will vary based on design choices you make while completing the project. Bluel: Terminal Window - Module 7 Options Original Results: Votes Recieved % of Total Votes Candidate Tony Stark Henry Pym Bruce Banner S_--- R---- C ---D----- 751 496 303 225 81 40.46 26.72 16.33 12.12 4.36 The total number of votes is: 1856 > Votes Recieved % of Total Votes Candidate Tony Stark Henry Pym Nick Fury Bruce Banner S-R---- C__-_D 751 496 518 303 225 81 31.63 20.89 21.82 12.76 9.48 3.41 The total number of votes is: 2374 > Candidate Votes Recieved % of Total Votes Tony Stark Henry Pym Nick Fury Clint Barton Bruce Banner S.--- R. C____D__ 751 496 518 426 303 225 81 26.82 17.71 18.50 15.21 10.82 8.04 2.89 The total number of votes is: 2800 Instructions: Working with the mock election, you will establish deletion algorithms to make updates to the array and ArrayList for the candidates. 1. Create a folder called 07.07 Assignment in your Module 07 assignments folder. 2. Copy your ElectionTesterv5.java and ElectionTesterv6.java files to the new folder. Rename V5 to 17 and V6 to 18. Be sure to update the class statement as well to reflect the new versions. 3. Modify the ElectionTestervo program to include the following: a. a method that will delete a particular candidate by position in the election array. Its arguments should include the array and a position to delete. b. a method that will find a particular candidate by name and delete that candidate. Its arguments should include the array the name of the candidate to delete. c. modify the other methods related to traversing, replacing, and inserting since you are working with an array. They need to work even if the array has some null entries. 4. Test your program by calling each of the methods. Be sure to print the election results after each change. See the sample output below. Clearly announce the change that should occur so someone reading your output knows which change occurred. a. delete a candidate by position b. delete a candidate by name 5. Now modify the ElectionTesterv8 class that uses an ArrayList to include the same functionality as the array version. 6. The final output for V7 and V8 needs to show the insertion and deletion methods in action. Be sure to clearly label each change so you can verify the code worked properly. Expected Output: This is a sample of the expected output. The details will vary based on design choices you make while completing the project Bluel Terminal Window - Module 7 - Options Votes Recieved % of Total Votes Candidate Tony Stark Henry Pym Bruce Banner S_R R_. C---- D P ---P PC----- M ---H-- 751 496 303 225 451 634 235 204 22.76 15.03 9.18 6.82 13.67 19.22 7.12 6.18 The total number of votes is: 3299 : > Votes Recieved Candidate Tony Stark Henry Pym Bruce Banner S--- R P.---.P P___ C M ---H 751 496 303 225 634 235 204 % of Total Votes ====== 26.37 17.42 10.64 7.90 22.26 8.25 7.16 The total number of votes is: 2848 > Votes Recieved % of Total Votes Candidate Tony Stark Bruce Banner S--- R P------ P---- P__C MH- 751 303 225 634 235 204 31.93 12.88 9.57 26.96 9.99 8.67 The total number of votes is: 2352 07.07 Deletions Lesson Grading Rubric Points Possible Points Earned Components Comments included for name, date, and purpose of the program. 1 Two tester classes properly setup for an array and ArrayList. 3 Insertion methods for inserting by position and inserting before an existing candidate properly written. 4. Deletion methods for deleting by position and deleting by name properly written. 4 Other methods for traversing, replacing, and inserting modified for null elements. 4. Methods invoked and run properly for both an array and ArrayList. 2 Output is clearly labeled, formatted, and accurate. 3 No compiler errors. 2 No runtime errors. 1 Thoughtful PMR included. 1 Total 25