Question
//ALL USING JAVA package questin1; import java.util.ArrayList; /** * Remove Oatmeal from the ArrayList. * Add the name of your favorite breakfast food to the
//ALL USING JAVA package questin1; import java.util.ArrayList; /** * Remove Oatmeal from the ArrayList. * Add the name of your favorite breakfast food to the ArrayList. * Add Cornflakes to the ArrayList. * Display all of the items in the ArrayList. * Print a message if the ArrayList contains Special K. Print a different message if it does not contain "Special K". */ public class Question_1_Cereals_ArrayList { public static void main(String[] args) { new Question_1_Cereals_ArrayList().cereals(); } ArrayList cereals = new ArrayList(); public void cereals() { // Don't modify these three lines cereals.add("Special K"); cereals.add("Captain Crunch"); cereals.add("Oatmeal"); // TODO Remove Oatmeal from the ArrayList. // TODO Add the name of your favorite breakfast cereal (or favorite breakfast food) to the ArrayList. // TODO Add Cornflakes to the ArrayList. // TODO Print all of the items in the ArrayList, one per line // TODO Print the exact message "Special K is in the ArrayList" if the ArrayList contains Special K. // TODO Print a different message if it does not contain "Special K". // TODO (optional) non-programming question: what does Captain Crunch have to do with computer hacking? } }
------------------------------------------------------------------------------------
package question2; import input.InputUtils; import java.lang.reflect.Array; import java.util.ArrayList; import java.util.Random; import static input.InputUtils.positiveIntInput; /** Write a program to roll a set of dice. Generate a random number between 1 and 6 for each dice to be rolled, and save the values in an ArrayList. Display the total of all the dice rolled. In some games, rolling the same number on all dice has a special meaning. In your program, check if all die have the same value, and print a message if all dice show the same value. note: your methods should work for more or less than 2 dice. So diceTotal() and allSameValue() should also work with 0, 1, 3, 4, 100 dice values in the ArrayList. */ public class Question_2_Dice_Rolls_ArrayList { public final String SAME_VALUES = "All the dice have the same value!"; Random rnd = new Random(); // Use this Random number generator in your code. public static void main(String[] args) { Question_2_Dice_Rolls_ArrayList dice = new Question_2_Dice_Rolls_ArrayList(); dice.rollDice(); } public void rollDice() { // How many dice to roll? int numberOfDice = positiveIntInput("How many dice would you like to roll?"); // A do loop is similar to a while loop, but the condition is // checked at the end of one loop iteration. // So the set of dice are always rolled at least one time. do { // Roll the dice ArrayList diceValues = roll(numberOfDice); // Print the dice values rolled System.out.println("The dice have the values: " + diceValues); System.out.println("The total of all dice: " + diceTotal(diceValues)); if (allSameValue(diceValues)) { System.out.println(SAME_VALUES); } } while (userWantsToContinue()); } public boolean userWantsToContinue() { // TODO Ask the user if they want to roll again. Return true if so, return false if not // TODO Use the yesNoInput method in InputUtils. return false; // Replace with your code } public ArrayList roll(int numberOfDice) { // TODO Roll the given number of dice. Store the values in an ArrayList and return it. // Use the global Random rnd to generate random numbers return null; // Replace with your code } public int diceTotal(ArrayList diceValues) { // TODO add up all of the values in the ArrayList and return this total. // TODO this should still work for any number of dice in the diceValues ArrayList. // TODO if the diceValues ArrayList is empty or null, return 0 (zero) return 0; // Replace with your code. } public boolean allSameValue(ArrayList diceValues) { // TODO return true if all of the values in the diceValues ArrayList are the same. // TODO return false for an empty or null ArrayList. // TODO this method should work for 1 dice, 2 dice, 3 dice, 100 dice... return false; // Replace with your code } }
---------------------------------------------------------
package question3; /** * You work for a recycling company. Youd like to collect some statistics on how much each of the houses on a certain street is recycling. Each house has to use crates for their recycling. Your program will count the number of crates set out by each house. This street is a little unusual since it only has 8 houses, and the city planner must have been a computer programmer, since the house numbers are 0, 1, 2, 3, 4, 5, 6, and 7. (Hint the house numbers are the same as array element indexes.) Write a program that asks for the number of recycling crates set out by each house. You should store, and process this data, in an array. DON'T use an ArrayList or LinkedList! Analyse your data and determine: How many recycling crates, in total, from all the houses on the street? What is the largest number of crates set out by any house? What is the smallest number of crates set out by any house? Which house had the most recycling? Display that house number. */ public class Question_3_Recycling_Truck_Arrays { public static void main(String[] args) { new Question_3_Recycling_Truck_Arrays().recycling(); } public void recycling() { int numberOfHouses = 8; int[] cratesPerHouse = getRecyclingPerHouse(numberOfHouses); int totalCrates = calculateTotal(cratesPerHouse); int maxCrates = calculateMax(cratesPerHouse); int minCrates = calculateMin(cratesPerHouse); int houseWithMostRecycling = calculateHouseWithMostRecycling(cratesPerHouse); System.out.println("Total crates from all houses = " + totalCrates); System.out.println("Max crates at any house = " + maxCrates); System.out.println("Min crates at any house = " + minCrates); System.out.println("House with most recycling = " + houseWithMostRecycling); } // Ask user for number of crates for each house. Store in array and return this array. public int[] getRecyclingPerHouse(int houses) { // TODO ask user for input. return null; // Replace with your code } //Add up all of the numbers in the array and return that public int calculateTotal(int[] cratesPerHouse) { // TODO calculate and return the total. return 0; // Replace with your code } //Which is the largest number in the array? public int calculateMax(int[] cratesPerHouse) { // TODO identify the largest number in the array. return 0; // Replace with your code } //Which is the smallest number in the array? public int calculateMin(int[] cratesPerHouse) { // TODO identify the smallest number return 0; // Replace with your code } //Use the array to figure out which house number - or array element number - has the most recycling public int calculateHouseWithMostRecycling(int[] cratesPerHouse) { // TODO which house had the most recycling? If more than one house // had the max number, return either house number. return 0; // Replace with your code } }
----------------------------------------------------------------------------------------------
package question4; import java.util.HashMap; import static input.InputUtils.*; /** * Extend this program to: * Ask user for a month, and snowfall amount, and add this data to HashMap * Check if month is already in HashMap before adding data. If so, warn user that they will overwrite data, and ask for confirmation before writing. * For month names, assume that all month names will be the full name, with the * initial letter capitalized, and will always be spelled the same. You may add input validation if you like. * * Identify the month with the most snowfall * Add up all of the snowfall amounts and display the total
* When finding the month with the max amount of snow; what if there are 2 or more months with the same max? (e.g. January:5, February:5; March:2}. Return any of the month names that have this joint max value.
*/ public class Question_4_Snowfall_HashMap { // Global HashMap, your methods will read and modify this. HashMap snowfall = new HashMap(); public static void main(String[] args) { new Question_4_Snowfall_HashMap().snow(); } public void snow() { // Add some example data snowfall.put("January", 3.7); snowfall.put("February", 10.2); snowfall.put("March", 6.5); snowfall.put("April", 0.1); snowfall.put("May", 0.0); getNewMonthData(); String monthWithMostSnow = maxSnow(); System.out.println("The month with most snow was " + monthWithMostSnow); double totalSnow = totalSnow(); System.out.println("The total snow was " + totalSnow); System.out.println("All of the data: "); // TODO answer this question: is the data printed in order? Is that ok? for (String month : snowfall.keySet()) { System.out.println("Month: " + month + ", Snowfall (inches): " + snowfall.get(month)); } } // You don't need to modify this method. public void getNewMonthData() { String month = stringInput("Enter month name"); double snow = doubleInput("Enter amount of snow"); addToHashMap(month, snow); } public void addToHashMap(String month, double snow) { // TODO finish this method // Check if data is in HashMap if (monthInHashMap(month)) { // If so, confirm if user wants to overwrite it if (yesNoInput("Do you want to overwrite your data?")) { // TODO what should happen here? } else { // TODO what should happen here? } } else { // TODO what should happen here? } } public boolean monthInHashMap(String month) { //TODO check if month is already a key in the in HashMap. return false; // replace with your code } public double totalSnow() { // TODO add up the snow in the HashMap, and return the total. return 0; // replace with your code } public String maxSnow() { // TODO figure out the month with the most snow, and return that month's name. return null; // replace with your code } }
==============================================================
package qeustion5; import static input.InputUtils.*; /** * You are a runner, and you are in training for a race. You'd like to keep track of all of your times for your training runs. You only like to run around lakes. Here's some example data, Cedar, 45.15 Cedar, 43.32 Harriet, 49.34 Harriet, 44.43 Harriet, 46.22 Como, 32.11 Como, 28.14 Write a program that enables you to enter the names of lakes and times, and store it all of this data in data structure(s). Your data structure should save EVERY time entered for each lake. Don't store it in individual variables. Your program should still work if you started running around another lake too (e.g. Lake of the Isles, or Lake Phalen). Your program should be able to analyze the data that you have stored. You should be able to print your fastest time for each lake you ran around. So, for the data above, your program will calculate something like Cedar, 43.32 Harriet, 44.43 Como, 28.14 You should also be able to calculate the average time. So, for the same data as above, your program will calculate something like this (you may truncate, or round numbers to 2 decimal places) Cedar, 44.23 Harriet, 46.66 Como, 30.12 Your program should be case-insensitive. So "Como" is the same lake as "como" or "COMO". Print all of the the data - Lake Name, Average, and Fastest Time, in a table. Your program should use the generic types of data structures. You should use methods to organize your program. Hint: you may need to combine more than one type of data structure. */ public class Question_5_Lake_Running { // TODO create a global data structure to store all of your lakes and times here. // You methods will read and write to this data structure. public static void main(String[] args) { new Question_5_Lake_Running().running(); } public void running() { while (moreLakes()) { String name = stringInput("Enter lake name"); double time = doubleInput("Enter time for running lake " + name); addLake(name, time); } printReportForAllLakes(); } public void printReportForAllLakes() { // TODO read data from your data structure, and print the average and fastest time for each lake. /* Your output should look something like this, with the average, followed by the fastest. Lake Average Fastest Cedar 44.23 43.32 Harriet 46.66 44.43 Como 30.12 28.12 */ // Use the fastestTimeForLake method, and averageTimeForLake method to help. } public double fastestTimeForLake(String lakeName) { // TODO read data from your data structure, and find the fastest time for the lake of this name. // Your lake name should not be case sensitive. "Como" is the same as "como". // If the lake is not in your data structure, return -1 to indicate it was not found. return 0; // replace with your code } public double averageTimeForLake(String lakeName) { // TODO read data from your data structure, and find the average time for the lake of this name. // Your lake name should not be case sensitive. "Como" is the same as "como". // If the lake is not in your data structure, return -1 to indicate it was not found. return 0; // replace with your code } public void addLake(String name, double time) { // TODO Add the data for this lake to your data structure // Your lake name should not be case sensitive. "Como" is the same as "como". } // You don't need to modify this method. private boolean moreLakes() { return yesNoInput("More lake data to add?"); } }
=================================================================
package question6; import java.util.ArrayList; import static com.google.common.collect.Lists.newArrayList; import static input.InputUtils.*; /** * A first prototype of a program that plays a simplified version of the children's card game Go Fish against you. This version is based from the rules given at https://en.wikipedia.org/wiki/Go_Fish "Seven cards are dealt from a standard 52-card deck to each player. The remaining cards are spread out in a disorderly pile referred to as the "pool". The player whose turn it is to play asks another player for his or her cards of a particular face value. For example Alice may ask, "Bob, do you have any threes?" Alice must have at least one card of the rank she requested. Bob must hand over all cards of that rank if possible. If he has none, Bob tells Alice to "go fish" and Alice draws a card from the pool and places it in her own hand. Then it is the next player's turn unless the card Alice drew is the card she asked for, in which case she shows it to the other players, and she gets another turn. When any player at any time has all four cards of one face value, it forms a book, and the cards must be placed face up in front of that player. Play proceeds to the left. When all sets of cards have been laid down in books, the game ends. The player with the most books wins." Your tasks: finish the incomplete methods. Run and test the program. Also run the unit tests. You might want to add some extra System.out.println() statements to update the player on the status of the game. Optional extra challenge: Write your own test to check the behavior of your selectComputerCardValue method. */ public class Question_6_Go_Fish { static ArrayList pool; // Uses a Google Guava utility method to create an ArrayList from a set of values. static final ArrayList cardValues = newArrayList( "A", "2", "3", "4", "5", "6", "7", "8", "9", "10", "J", "Q", "K" ); // List of player's cards, list of computer's cards static ArrayList playerHand, computerHand; // List of values that the player and computer have books of. For example, // if the player has made a book of 6s and a book of Qs, then playerBooks = [ "6", "Q" ] static ArrayList playerBooks, computerBooks; // Players' names final static String HUMAN = "Human"; final static String COMPUTER = "Computer"; public static void main(String[] args) { // Create a deck of cards, ArrayList deck = createDeck(); // Deal cards to two players playerHand = dealHand(deck); computerHand = dealHand(deck); // Empty lists for storing books made playerBooks = new ArrayList<>(); computerBooks = new ArrayList<>(); // Set up the pool createPool(deck); // Play game - players take turns to play while (!gameOver()) { playerTurn(); computerTurn(); } // Game is over, identify the winner and display message. System.out.println("The winner is " + identifyWinner()); printGameStats(); } /** Methods to set up game */ public static ArrayList createDeck() { // TODO create an array with 52 strings, each representing a card value in a standard deck. // Your strings can be "A" "2", "3", "4" ... since suits don't matter in this game. // Use the static cardValues ArrayList. // Shuffle the deck. return null; } public static void createPool(ArrayList deck) { // TODO copy all cards from deck to pool // This method should modify the global pool variable. It does not need to return anything. } public static ArrayList dealHand(ArrayList deck) { // TODO create a new ArrayList to represent a hand of cards. // Deal from the *start* of the ArrayList. // TODO how should you handle with running out of cards to deal? // deal seven cards from the deck - remove from deck, add to the hand. return null; } /** Game play methods */ public static void playerTurn() { // human plays turn. // Ask computer for a card. // If computer does not have this card, human goes fishing // If computer does have card, take all cards of this values from computer // and add to hand. Player gets another go. // TODO add some messages for the user so they know what's happening. while (true) { // Show the player their current cards displayHand(playerHand); // What card does the human want to request from the computer? String cardRequested = cardValueInput(); if (handHasCard(computerHand, cardRequested)) { transfer(cardRequested, computerHand, playerHand); makeBooks(playerHand, playerBooks); } else { goFish(playerHand); makeBooks(playerHand, playerBooks); return; // end turn } } } public static void computerTurn() { // Similar to playerTurn, but calls a different method for computer to select their card. // TODO add some messages for the user so they know it's the computer's turn, and which card the computer requests etc.. while (true) { // Select card value to request from player String cardRequested = selectComputerCardValue(); // Request this card from human player if (handHasCard(playerHand, cardRequested)) { transfer(cardRequested, playerHand, computerHand); makeBooks(computerHand, computerBooks); } else { goFish(computerHand); makeBooks(computerHand, computerBooks); return; // end turn } } } /** Helper methods to break down game logic into discreet steps */ public static String selectComputerCardValue() { // TODO Select a valid card value from computerHand. A basic computer // strategy could be to select a card at random, or to request the value of the first card in their hand. // You will need to write a test for this method. return null; } public static void goFish(ArrayList hand) { //TODO remove card from pool and add to this hand. // The cards should be shuffled. // Take the FIRST card from the pool and add it to the end of the hand. //TODO test that the pool is not empty. If pool is empty, don't modify hand or pool. //This method does not need to return anything. // Pass-by-reference means that any modifications made to hand are the same changes made to hand in the calling method. } public static boolean handHasCard(ArrayList hand, String cardRequested) { // TODO Check if any cards of this value are present in the hand. Return true if so. return false; } public static void transfer(String card, ArrayList fromHand, ArrayList toHand) { // TODO transfer all cards of the given value from one hand to the other. // example: if card = "5" then remove all "5" from fromHand and add them to toHand. // example: card = "5" , fromHand = [ 5, 6, 7, 2, 5 ], toHand = [ 1, 2 ] // After transfer, fromHand = [ 6, 7, 2 ], toHand = [ 1, 2, 5, 5 ] } public static void makeBooks(ArrayList hand, ArrayList books) { // TODO make books for this hand. Remove cards from the hand, and add one entry to the books list. // example: hand has 4 "6" values in, so a book of 6s. Remove all "6" from hand, and add one "6" to books. // example: hand starts as ["6","6","6","2","6","7"], books starts as ["4", "Q"] // After transfer, hand = ["2","7"] and books = ["4","Q","6"] // Your method should work if there is more than one book in the hand // Example: ["6","6","A","6","2","A","6","A","7","A"] and books is ["4", "Q"] // Hand should become ["2","7"] and books = ["4","Q","6", "A"] } public static boolean gameOver() { // TODO test if the pool is empty. return false; } public static String identifyWinner() { // TODO return "Computer" if computer wins, return "Human" if human player wins. Use the global static player name Strings. // Count the number of books each player has made, the player with the most books is the winner. return null; } /** Input and output methods */ public static void displayHand(ArrayList hand) { // TODO Display all of the cards in this hand. } /** Ask player for a card. Checks to see if the value is a valid value for a card, in the range A, 2, 3 .... J , Q , K * Also checks to see if the player does already have a card of that value in their hand. Players can only request * cards that they already have one of that value in their hand. */ public static String cardValueInput() { while (true) { String card = stringInput("Enter the card value to request").toUpperCase(); // Is this a valid value A - K ? if (!cardValues.contains(card)) { System.out.println("Enter a valid card value."); continue; } // If this a value that the human player has in their hand? No cheating! if (!handHasCard(playerHand, card)){ System.out.println("You don't have a " + card + " in your hand. Try again."); continue; } return card; } } public static void printGameStats() { // TODO Display if human or computer wins by examining computerBooks and playerBooks. - who has the most books? // Display list of books each player has, and the total number of books. } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
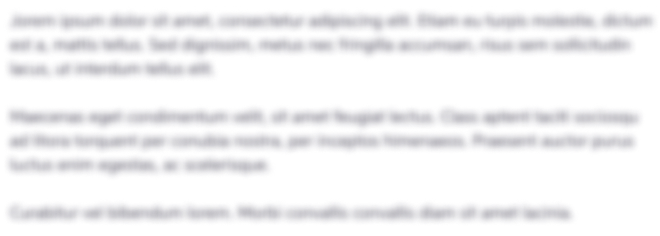
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started