Answered step by step
Verified Expert Solution
Question
1 Approved Answer
All your code should be in a package called calculator. Your tests should be in the default package. This ensures that your test can see
All your code should be in a package called "calculator". Your tests should be in the default package. This ensures that your test can see your classes and interfaces in the same way as any other client.
The Calculator interface
Write an interface Calculator that represents a single calculator. This interface should contain the following:
A method input that takes a single character as its only argument. This method should return a Calculator object as a result of processing the input.
A method getResult that does not take any arguments and returns the current "result" of the calculator ie the message that we would normally see on the screen as a String object.
The SimpleCalculator implementation
A simple calculator takes straightforward inputs. Due to limited processing power it cannot work with whole numbers longer than bits. This calculator has the following characteristics:
A correct basic sequence of inputs is the first operand, followed by the operator, followed by the second operand, followed by eg Note that each operand may have multiple digits.
A valid sequence can contain a sequence of operands and operators eg etc.
The result at any point should show either what was entered thus far, or the result. For example, for the sequence of inputs the result after each input should be in that order. For the sequence of inputs the result after each input should be in that order. Before entering any inputs, the result should be the blank string.
The only valid operand characters are and the only valid operators are and
The input C will clear the calculator inputs. The result after clearing should be the blank string.
The calculator does not "infer" any missing inputs. For example, although etc. is valid input on a normal calculator, this calculator will report that as an error.
The calculator does allow inputting multiple times. In this case it will return the same result. For example the result after and is the same: This is not what a normal calculator will do
The calculator does not allow inputting negative numbers, although it can handle negative results.
If an operand overflows, it should throw an IllegalArgumentException and the operand's value before the input that caused it to overflow should be retained.
It throws an IllegalArgumentException for all invalid inputs and sequences. However it throws a RuntimeException if a valid input causes an operation to overflow. If the operand does not overflow but the result of the arithmetic does, then the result reported should be For example, a b should result in if ab will overflow.
The input method is not expected to change the calling object.
Implement this in a class called SimpleCalculator Write tests to thoroughly test your class.
The SmartCalculator implementation
A smart calculator accepts inputs like a normal calculator. This calculator is backward compatible with the simple calculator ie it can handle everything the simple calculator can Due to limited processing power it too cannot work with whole numbers longer than bits. However this calculator can also handle the following "smart" inputs:
Input multiple times: produces as before. However and are also valid input sequences, and produce and respectively.
Skipping the second operand: the input produces The input produces and so on The state at the end of each is the result of the computation thus far.
Two consecutive operators: ignores the first operator, and produces as the result.
Begin with operator: ignores the and produces as the result. Note that this only applies to the operator as it has a mathematical meaning when it comes before an operand. All other operators before operands are invalid.
Like SimpleCalculator it does not allow negative inputs although it can handle negative numbers, and it uses IllegalArgumentException to report all invalid inputs and sequences. However it throws a RuntimeException if a valid input causes an operand to overflow. If the operand does not overflow but the result of the arithmetic does, then the result reported should be For example, a b should result in if ab will overflow.
The input method is not expected to change the calling object.
Implement this in a class called SmartCalculator.Write tests to thoroughly test your class.
Tests
Write tests for your implementations. You may be able to abstract some tests that are common to both implementations. Read the test notes on the course web page to help you design tests effectively.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
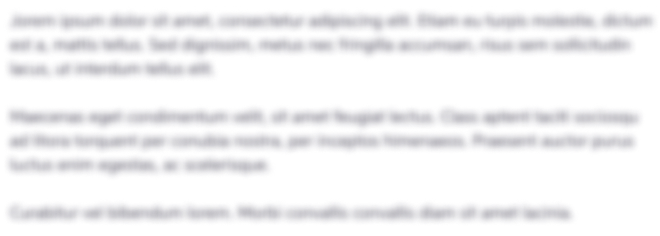
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started