Question
Allowed Functions All functions and knowledge that we've covered so far in lectures and Stepik readings are allowed to use. You may find the following
Allowed Functions
All functions and knowledge that we've covered so far in lectures and Stepik readings are allowed to use. You may find the following functions particularly useful in this PA:
-
list functions: append()
-
string functions: strip(), split()
-
other built-in functions or techniques: len(), range(), min(), in keyword, string/list slicing ([start:stop:step])
Please do NOT use functions that we have not discussed in this class.
Part 2.1: Required Questions
dining_hall_menus.py
Create a new file called dining_hall_menus.py, where you will implement the function:
def dining_hall_menus(ovt_menu, pines_menu)
The function will take in 2 lists, ovt_menu and pines_menu and returns the difference between the two menus offered that day. We define the "difference" between two menus as a list of unique items that one menu has but the other menu doesn't. For example, the function call dining_hall_menus(["pizza", "spaghetti", "salad", "fries"], ["burgers", "sushi", "fries", "pizza"]) would return ["spaghetti", "salad", "burgers", "sushi"].
Note the following:
-
Items in the menus must match the exact capitalization to be considered as the same. For example, the function call dining_hall_menus(["pizza"], ["Pizza"]) would return ["pizza", "Pizza"].
-
ovt_menu and pines_menu may have duplicates within the list, but will be treated as one item. For example, the function call dining_hall_menus(["pizza", "salad", "salad"], ["pizza"]) would return the list ["salad"].
-
Empty lists are valid arguments, passing in a single empty list and a non-empty list should return a list of the unique items in the non-empty list. For example, the function call dining_hall_menus([], ["fries", "fries"]) would return ["fries"]. Passing in two empty lists would return an empty list.
-
Order of the items in the returned list does not matter.
count_exact_match.py
Create a new file calledcount_exact_match.py. In this file, you would need to implement the following function:
def count_exact_match(text, query)
This function takes in two strings text and query, and returns the number of exact matches between them. An exact match is the case where the same character appears at exactly the same index of both strings. For example, count_exact_match("severussnape", "alanrickman") returns 2, because there are two exact matches -- in both strings, character 'r' appears at index 4, and character 'a' appears at index 9.
Note the following:
-
text and query may have different lengths
-
In the case where a string is an empty string "" , the returning value should be 0
-
The function is case-sensitive, so 'r' and 'R' are not considered as the same character
validate_email.py
Create a new file called validate_email.py. In this file, you would need to implement the following function:
def validate_email(email_address,email_suffix)
This function takes in two strings email_address and email_suffix and returns True if the email address is valid and False otherwise. We define email_address as valid if the substring of what lies right of "@" exactly matches email_suffix. You should check the validity of the email address with the following rules:
-
email_address should contain exactly one "@". For example, for email_suffix = "gail.com", email_address = "hello@world@gail.com" is not valid, but email_address="helloworld@gail.com" is.
-
Other than leading and trailing spaces, email_address should not contain any space. For example, for email_suffix = "gail.com", email_address = " hello world@gail.com " is not valid because of the spaces between "hello" and "world" but email_address = " helloworld@gail.com " is valid.
-
The substring between "@" (not including "@") and the first trailing space (not including the space) or the end of the string if there is no trailing space should match the email_suffix. For example, for email_address = " helloworld@gail.com " , it is valid if email_suffix = "gail.com", but not valid if email_suffix = "ucsd.edu".
-
email_suffix can be any string, which means it may contains leading/trailing spaces itself. For example, email_address = " hello@ . " and email_suffix = " ." should return False because of the leading space in the suffix.
Part 2.2: Optional Extra Credit Questions
In this part, we are giving you a chance to earn up to 5 extra points for this PA. The EC part is totally optional, and we will not penalize you if you decide not to attempt it.
Create a new file calledcompute_string_distance_ec.py. In this file, you would need to implement the following two functions:
-
def compute_string_distance(text,query) This function takes in two strings of equal length and returns the distance between the two strings. Distance here is defined as the number of characters at the corresponding indices that are different (known as the hamming distance). You may assume the input strings have the same length.
In the example below, the string "Hello" and "Hewwo" has a distance of 2 because the characters at position 2 and 3 are different as indicated by the red line, so the function would return 2 for the function call compute_string_distance("hello","hewwo").
-
def min_distance_substring(text,query) This function takes in two strings text and query where len(text) >= len(query) and returns the starting position of the first substring of text with same length as query that has the smallest distance between the substring and the query.
-
Note: the compute_string_distance function you just defined might be useful and you may call the function here.
-
Consider the example below with text = "hello" and query = "l". There are 5 substrings with length 1 in the text ("h","e","l","l","o"). When comparing the distance between the substrings and the query, there are two string substrings with distance 0 at index 2 and 3. In this case your function should return 2 as that's the first occurrence of the substring with the smallest distance.
Part 3: Style
Coding style is an important part of ensuring readability and maintainability of your code. Starting from this assignment on, we will grade your coding style in all submitted code files (including the optional extra credit file) according to the style guidelines. You can keep these guidelines in mind as you write your code or go back and fix your code at the end. For now, we will mainly be grading the following:
-
Use of descriptive variable names: The names of your variables should describe the data they hold. Your variable names should be words (or abbreviations), not single letters.
-
e.g. a --> index_of_apple; letter1 and letter2 --> lower_case_letter and upper_case_letter
-
Exception: If it is a loop index like i, j, k, one char is OK and sometimes preferred.
-
-
Inline Comments: If there is a length of code that is left unexplained, take the time to type a non-redundant line summarizing this length of code (e.g. #initialize an int is redundant, vs. #set initial length to 10 inches is not). It will let others who look at your code understand what's going on without having to spend time understanding your logic first. But don't be too descriptive, as too many comments reduce readability.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
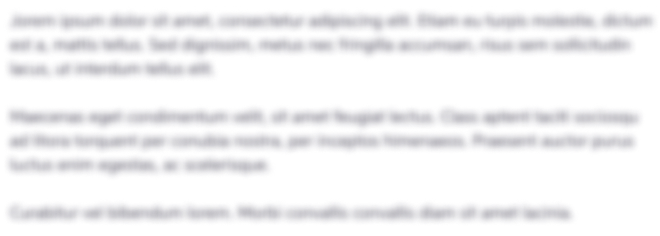
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started