Question
Although the rank of a card will be stored as an integer, we will sometimes need a string representation of the rank. For example, when
Although the rank of a card will be stored as an integer, we will sometimes need a string representation of the rank. For example, when printing a Queen (which has a rank of 12), we will use the string "Q" instead of the integer 12. This is why we have provided you with the RANK_STRINGS array, which is a class constant that contains string representations of all of the card ranks.
As the comments that weve included above the array indicate, if a card has a rank of r, the corresponding rank string can be obtained using the expression RANK_STRINGS[r]. For example, 12 is the numeric rank of a Queen card, so RANK_STRINGS[12] gives us the string "Q".
A) Your first task is to add a static method called rankNumFor that goes in the reverse direction taking a rank string as its only parameter and returning the corresponding integer rank. In other words, the method should find and return the index of the specified rank string in the RANK_STRINGS array. For example:
rankNumFor("Q") should return 12, because "Q" has an index of 12 in the RANK_STRINGS array.
rankNumFor("A") should return 1, because "A" has an index of 1 in the RANK_STRINGS array.
rankNumFor("10") should return 10, because "10" has an index of 10 in the RANK_STRINGS array.
If the value of the parameter is null or if it doesnt appear in the RANK_STRINGS array, the method should return -1. For example, rankNumFor("B") should return -1.
Important notes:
Because the smallest possible rank is 1, we have put an empty string in position 0 of the RANK_STRINGS array. When processing the array, you should ignore position 0.
Your method should work even if we change the contents of the RANK_STRINGS array. In other words, you should write code that processes any array of String objects that is assigned to the class constant RANK_STRINGS. The only assumption you should make about the array is that element 0 will be an empty string.
This method (unlike most of the others you will write for this problem) is static, because it doesnt need to access the fields of a Card object. Rather, it gets all of the information that it needs from its parameter and from the RANK_STRINGS array.
B) Add another static method called isValidSuit that takes a single-character representation of a cards suit and returns true if that suit is valid (i.e., if it is one of the values in the SUITS array), and false otherwise. For example:
isValidSuit('D') should return true, because 'D' appears in the SUITS array
isValidSuit('B') should return false, because 'B' does not appear in the SUITS array.
Important: Your method should work even if we change the contents of the SUITS array. In other words, you should write code that processes any array of char values that is assigned to the class constant SUITS, and you should not make any assumptions about the chars found in that array.
Here again, this method is static, because it doesnt need to access the fields of a Card object. Rather, it gets all of the information that it needs from its parameter and the SUITS array.
Testing your static methods Before you proceed with the remaining steps, we highly recommend adding a main method to your Card class that makes test calls to the two static methods that you just implemented. Take whatever steps are needed to ensure that they work correctly.
CODE:
public class Card {
/* constants for the ranks of non-numeric cards */
public static final int ACE = 1;
public static final int JACK = 11;
public static final int QUEEN = 12;
public static final int KING = 13;
/* other constants for the ranks */
public static final int FIRST_RANK = 1;
public static final int LAST_RANK = 13;
/*
* class-constant array containing the string representations
* of all of the card ranks.
* The string for the numeric rank r is given by RANK_STRINGS[r].
*/
public static final String[] RANK_STRINGS = {
null, "A", "2", "3", "4", "5", "6",
"7", "8", "9", "10", "J", "Q", "K"
};
/*
* class-constant array containing the char representations
* of all of the possible suits.
*/
public static final char[] SUITS = {'C', 'D', 'H', 'S'};
/* Put the rest of the class definition below. */
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
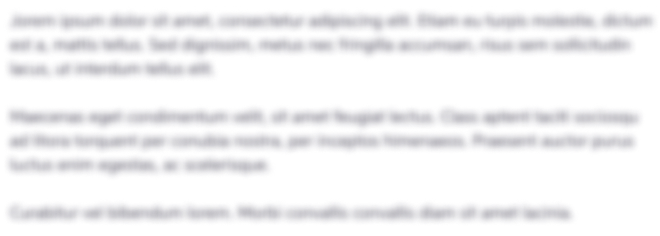
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started