Question
Am unable to print out results, any ideas why? public class TaxiHomework { public static void main(String[] args) { int[] cabID = {231, 155, 619,
Am unable to print out results, any ideas why?
public class TaxiHomework
{
public static void main(String[] args)
{
int[] cabID = {231, 155, 619, 127, 333};
TaxiCab[] fleet;
double[] collection;
int cntr;
String name;
int fares;
String choice;
name = "none yet";
fares = 0;
collection = new double[0];
fleet = new TaxiCab[cabID.length];
for(cntr = 0; cntr < cabID.length; ++cntr)
{
choice = MyUtilityClass.inputString("Would you like to Deploy "
+ "Cab #: "+ cabID[cntr] +" " + "Enter Yes or No");
if(choice.equalsIgnoreCase("Yes"))
{
name = MyUtilityClass.inputString("Enter Name of Driver:\t");
fares = MyUtilityClass.inputInteger("Enter in the Amount of Fares Taken:\t");
fleet[cntr] = new TaxiCab(name, true, fares);
}
else
{
fleet[cntr] = new TaxiCab(name, false, 0);
}
}
System.out.println(fleet);
}
}
class TaxiCab
{
private String driverName; //name of driver
private int acceptedFare; //amount of accepted fares
private double fareAmount[]; //array of fares made by driver
private double totalCollected; //total money collected
private boolean deploy; //cab was deployed or not
//
// TaxiCab
//
// default constructor initialize taxi cab attributes
//
// Input: none
// Return: none
//
public TaxiCab()
{
driverName = "none yet";
acceptedFare = 0;
totalCollected = 0.0;
fareAmount = new double[acceptedFare];
deploy = false;
int cntr;
for(cntr = 0; cntr < fareAmount.length; ++cntr)
{
fareAmount[cntr] = 0.0;
}
}
//
// TaxiCab
// overloaded constructor for taxi cab class
//
// Input: acceptedFare number of fares accepted
// driverName name of driver
// Return: none
public TaxiCab(String driverName, boolean deploy, int acceptedFare)
{
this.acceptedFare = acceptedFare;
this.driverName = driverName;
totalCollected = 0.0;
this.deploy = deploy;
int cntr;
if(acceptedFare >= 0)
{
fareAmount = new double[acceptedFare];
//initialize array of accepted fares
for(cntr = 0; cntr < fareAmount.length; ++cntr)
{
fareAmount[cntr] = MyUtilityClass.inputDouble("Enter in Amount Made on Fare "
+ cntr + ":" + "\t");
}
}
}//end TaxiCab
//
// setDriverName
//
// the purpose of this method is to modify the name of the driver
//
// Input: driverName name of driver
// Return: none
//
public void setDriverName(String driverName)
{
this.driverName = driverName;
}//end setDriverName
//
// setAcceptedFare
//
// the purpose of this method is to modify the amount of accepted fares
//
// Input: acceptedFare amount of accepted fares by driver
// Return: none
//
public void setAcceptedFare(int acceptedFare)
{
//validate the amount of accepted fares
if(acceptedFare >= 0)
{
this.acceptedFare = acceptedFare;
}
else
{
System.out.println("Invalid entry of "+ acceptedFare);
}
}//end setAcceptedFare
//
// setFareAmount
//
// the purpose of this method is to modify a list of fares made by driver
//
// Input: double[] acceptedFare list of accepted fare amounts
// Return: none
//
public void setFareAmount(double[] fareAmount)
{
int cntr;
this.fareAmount = new double[fareAmount.length];
for(cntr = 0; cntr < fareAmount.length; ++cntr)
{
this.fareAmount[cntr] = fareAmount[cntr];
}
}//end setFareAmount
//
// setOneFareAmount
//
// the purpose of this method is to modify one fare collected
//
// Input: num index number of fare
// fare amount made by driver for one fare
// Return: none
//
public void setOneFareAmount(int num, double fare)
{
//validate index for
if(num >= 0 && num < fareAmount.length)
{
fareAmount[num] = fare;
}
else
{
System.out.println("Invalid index of "+ num);
}
}//end setOneFareAmount
public void setDeploy(boolean deploy)
{
this.deploy = deploy;
}
//
// setTotalCollected
//
// the purpose of this method is to modify the total money collected
// by the driver
// Input: totalCollected total money collected by driver
// Return: none
//
public void setTotalCollected(double totalCollected)
{
//validate money made by driver
if(totalCollected >= 0.0)
{
this.totalCollected = totalCollected;
}
else
{
System.out.println("Invalid entry of "+ totalCollected);
}
}//end setTotalCollected
//
// getDriverName
//
// the purpose of this method is to return a copy of driver name
//
// Input: none
// Return: driverName name of driver
//
public String getDriverName()
{
return(driverName);
}//end getDriverName
//
// getAcceptedFare
//
// the purpose of this method is to return a copy of the accepted fare amounts
//
// Input: none
// Return: acceptedFare amount of accepted fares by driver
//
public int getAcceptedFare()
{
return(acceptedFare);
}//end getAcceptedFare
//
// getFareAmount
//
// the purpose of this method is to return a copy of the list of fares collected
//
// Input: none
// Return: tmpArray temporary copy of array list
//
public double[] getFareAmount()
{
int cntr;
double[] tmp; //temporary array
tmp = new double[fareAmount.length];
for(cntr = 0; cntr < fareAmount.length; ++cntr)
{
tmp[cntr] = fareAmount[cntr];
}
return(tmp);
}
//
// getOneFareAmount
//
// the purpose of this method is to return one fare made by driver
//
// Input: index the list index number
// Return: retVal return value of data stored in list
//
public double getOneFareAmount(int index)
{
double retVal;
retVal = -1.0;
//validate index used to get fare amount
if(index >= 0 && index < fareAmount.length)
{
retVal = fareAmount[index];
}
else
{
System.out.println("Invalid entry of "+ index);
}
return(retVal);
}//end getOneFareAmount
//
// getTotalCollected
//
// the purpose of this method is to return a copy of total money collected
//
// Input: none
// Return: totalCollected total money collected by driver
//
public double getTotalCollected()
{
return(totalCollected);
}//end getTotalCollected
public boolean getDeploy()
{
return(deploy);
}
//
// toString
//
// the purpose of this method is to print out all data for taxi cab
//
// Input: none
// Return: retStr return output for taxicab
//
public String toString()
{
String retStr;
StringBuffer buff;
retStr = "none yet.";
buff = new StringBuffer();
buff.append("Drivers Name:\t" + driverName + " ");
int cntr;
for(cntr = 0; cntr < fareAmount.length; ++cntr)
{
buff.append("Fare Number " + cntr + "\t" + fareAmount[cntr] + " ");
}
buff.append("Total Amount Collected:\t$" + totalCollected + " ");
retStr = buff.toString();
return(retStr);
}// end toString
//
// handleTotalCost
//
// the purpose of this method is to calculate the total cost from
// the array of items
//
// Input: aFare list of taken fares from driver
// Return: none
//
public void handleTotalCost(double[] aFare)
{
int cntr;
for(cntr = 0; cntr < aFare.length; ++cntr)
{
totalCollected += aFare[cntr];
}
}// end handleTotalCost
//public double totalCollected(TaxiCab[] fleet, boolean depolyed)
{
double total;
total = 0.0;
// for(TaxiCab c : fleet)
{
// if(c)
{
}
}
}
//
// copyTaxiCab
//
// the purpose of this method is to create a copy of the taxi cab class attributes
//
// Input: tCab the cab to copy from
// Return: none
//
public void copyTaxiCab(TaxiCab tCab)
{
driverName = tCab.getDriverName();
acceptedFare = tCab.getAcceptedFare();
fareAmount = tCab.getFareAmount();
totalCollected = tCab.getTotalCollected();
}//end copyTaxiCab
}
import java.util.Scanner;
public class MyUtilityClass
{
//
// inputString
// the purpose of this method is to input
// a string from the keyboard
// Input: prompt message for dialog box
// Return: inputStr string entered at kbd
//
public static String inputString(String prompt)
{
Scanner input = new Scanner(System.in);
String inputStr;
// prompt user to enter in string
System.out.print(prompt);
// read in value from keyboard
inputStr = input.nextLine();
// return input string
return(inputStr);
} // end inputString
//
// inputInteger
// the purpose of this method is to input
// an integer from the keyboard
// Input: prompt message for dialog box
// Return: num integer entered at kbd
//
public static int inputInteger(String prompt)
{
Scanner input = new Scanner(System.in);
int num;
String cleanUpStr;
num = 0;
cleanUpStr = "none yet";
System.out.print(prompt);
num = input.nextInt();
return(num);
} // end inputInteger
//
// inputDouble
// the purpose of this method is to input
// a double from the keyboard
// Input: prompt message for dialog box
// Return: num double entered at kbd
//
public static double inputDouble(String prompt)
{
Scanner input = new Scanner(System.in);
double num;
String cleanUpStr;
num = 0.0;
cleanUpStr = "none yet";
System.out.print(prompt);
num = input.nextDouble();
return(num);
} // end inputDouble
//
// genRandom
//
// The purpose of this method is to generate a random number
// between the minimum and maximum values specified inclusively
//
// Input: min the minimum value in the range of numbers
// max the maximum value in the range of numbers
//
// Return: num the random number in the given range
//
public static int genRandom(int min, int max)
{
double rNum; // the original random number generated
double range; // the range of random numbers allowed
int num;
rNum = 0.0;
range = 0.0;
num = 0;
// generate a random number 0.0 <= rNum < 1.0
rNum = Math.random( );
// determine the range
range = (double)max - (double)min + 1.0;
// scale the number between min and max inclusively
rNum = rNum * range;
rNum = rNum + (double)min;
// convert back to integer value
num = (int)rNum;
// return the integer
return(num);
}// end genRandom
//
// fillArray
// the purpose of this method is to fill
// an array with integers entered from kbd
// Input: array array of integers
// Return: none
//
public static void fillArray(int[] array)
{
int cntr;
for(cntr = 0; cntr < array.length; ++cntr)
{
array[cntr] = inputInteger("Please enter a integer");
}
} // end fillArray
//
// fillRandomArray
//
// The purpose of this method is to fill an array of integers with
// random values between the minimum and maximum values inclusively.
//
// Input: array the array of integers
// minVal the minimum random value
// maxVal the maximum random value
// Return: none
//
public static void fillRandomArray(int[] array, int minVal, int maxVal)
{
int cntr;
// check to make sure that minValue is less than or equal to maxVal
if (minVal <= maxVal)
{
for (cntr = 0; cntr < array.length;++cntr)
{
array[cntr] = genRandom(minVal, maxVal);
}// end for each random value
}
else
{
System.out.println("Array cannot be filled because " + minVal +
" is not less than " + maxVal);
}
}// end fillRandomArray
//
// printArray
// the purpose of this method is to print out the values stored in the array.
// The values should be printed showing the index in one column and the
// corresponding values in the next column. A title should be included at
// the top of each column.
//
// Input: array the array of integers
// Return: none
//
public static void printArray(int[] array)
{
int cntr;
System.out.println("Index\t\tValue");
for (cntr=0;cntr < array.length;++cntr)
{
System.out.println(cntr + "\t\t" + array[cntr]);
}// end for each element of the array
}// end printArray
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
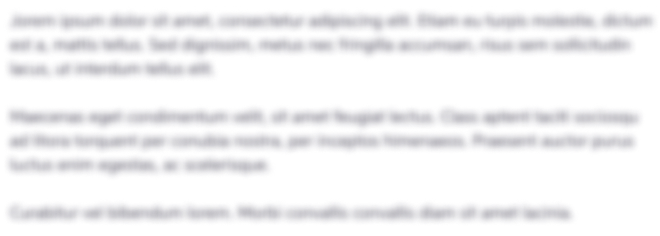
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started