Question
/** * An app for a mobile device * * Modifications: * CT: Added validation to setPrice * * * @author * @version 2018.04.30 *
/** * An app for a mobile device * * Modifications: * CT: Added validation to setPrice * * * @author * @version 2018.04.30 *
public class App { private String name; private String author; private double price; private int rating; // valid ratings are 1-4 or 0 meaning not rated /** * Create an app with the given name, author, price, and rating * @param appName the app name * @param appAuthor the app author * @param appPrice the price of the app (0 if the app is free) * @param appRating the app's rating */ public App(String appName, String appAuthor, double appPrice, int appRating) { name = appName; author = appAuthor; price = appPrice; // TODO: ----------------- 1 ------------------- // TODO: Replace the statement below with a call to setRating that has the same effect rating = appRating; // TODO: ----------------- 1b ------------------- // TODO: Answer this question in a comment right below (no code for this one) // TODO: (Tip: Update setRating based on TODO #3 below before answering.) // Question: Why do you think we want to replace the assignment statement with a call to setRating? // Your Answer: } /** * Create an app with the given name, author, and price that is not rated * @param appName the app name * @param appAuthor the app author * @param appPrice the price of the app (0 if the app is free) */ public App(String appName, String appAuthor, double appPrice) { name = appName; author = appAuthor; price = appPrice; rating = 0; }
/** * @return the app name */ public String getName() { return name; } /** * @return the app author */ public String getAuthor() { return author; } /** * @return the app price */ public double getPrice() { return price; } /** * Set the price of this app to the value given * @param newPrice new price for this app */ public void setPrice(double newPrice) { if (newPrice >= 0) { price = newPrice; } else { System.out.println("Error: Price must be greater than or equal to 0"); } } /** * @return true if this app is free, false otherwise */ public boolean isFree() { // TODO: ----------------- 2 ------------------- // TODO: Implement this method so that it behaves as specified. // TODO: (If an app's price is 0, that means it is free.) return false; } /** * @return the app rating */ public int getRating() { return rating; } /** * Set the rating of this app to the value given * @param newRating new rating for this app */ public void setRating(int newRating) { // TODO: ----------------- 3 ------------------- // TODO: Add validation: The new rating must be in the range 1 - 4 (inclusive) // TODO: If it's not, print an error message stating the valid range and don't update the rating rating = newRating; } /** * Reset the rating of this app to not rated */ public void resetRating() { rating = 0; } /** * Increase the rating of this app by 1 */ public void increaseRating() { // TODO: ----------------- 4 ------------------- // TODO: Update so that the rating is not allowed to go higher than 4. // TODO: I.e., if the rating is already at 4, calling this method has no effect. // TODO: Do not print anything in this method. rating = rating + 1; }
/** * Decrease the rating of this app by 1 */ public void decreaseRating() { // TODO: ----------------- 5 ------------------- // TODO: Update so that the rating is not allowed to go lower than 1. // TODO: If the rating is already at 1, calling this method has no effect. // TODO: If the rating is 0, calling this method has no effect. // TODO: Do not print anything in this method. rating = rating - 1; }
/** * Print information on this app */ public void printAppInfo() { System.out.println("---------------------------------"); System.out.println("App: " + name); System.out.println("Author: " + author); System.out.println("Price: $" + price); System.out.println("Rating: " + rating); // TODO: ----------------- 6 ------------------- // TODO: Update the two statements above: // TODO: If an app is free it should print "Price: FREE" instead of "Price: $0" // TODO: If an app is not rated yet (rating 0), it should print "Rating: (not rated)" // TOCO: instead of "Rating: 0" System.out.println("---------------------------------"); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
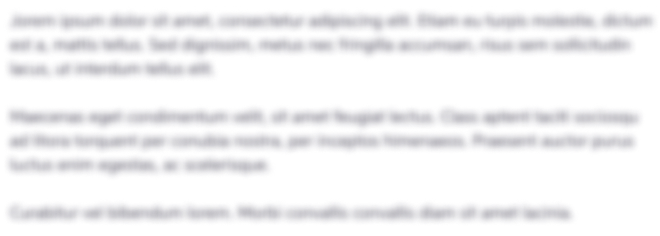
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started