Question
An array that can grow and shrink (as needed) at run time is generally called a dynamic array. You are to write a class named
An array that can grow and shrink (as needed) at run time is generally called a dynamic array. You are to write a class named DynArray which can hold double values that adheres to the following:
The physical size of the array at all times is a nonnegative power of two (smallest of which is 1).
The physical size is never more than 2 times the number of elements which occupy it (with exception to when it is at size 1).
Mandatory Instance variables:
private double[] array; private int size; private int nextIndex;
Mandatory Instance methods:
public DynArray() // constructor
// set array to a new array of double, of size one
// set size to one,
// and set nextIndex to zero.
public int arraySize() // accessor
// return the value of size.
public int elements() // accessor
// return the value of nextIndex.
public double at(int index) // accessor
// if 0 <= index < nextIndex
// return the value of array[index].
// else
// return Double.NaN.
private void grow()
// make array a reference to an array that is twice as large
// and contains the same values for indicies 0 through
// nextIndex - 1, and adjust size appropriately.
private void shrink()
// make array a reference to an array that is half as large
// and contains the same values for indicies 0 through
// nextIndex - 1, and adjust size appropriately.
public void insertAt(int index, double value) // mutator
// if 0 <= index <= nextIndex
// move the nessecary values over one so that value can
// be incerted at the location index in the array, inserts
// incerts value at the location index, and adjust nextIndex
// appropriately.
// Note a grow() may be neccessary before or after.
// else
// do nothing.
public void insert(double value) // mutator
// insert value at location nextIndex.
public double removeAt(int index) // mutator
// if 0 <= index < nextIndex
// move the nessecary values over one as to eliminate
// the value at the location index in the array, adjust
// nextIndex appropriately, and return the value that was
// at the location index .
// Note: a shrink() may be neccessary before or after.
// else
// return Double.NaN.
public double remove() // mutator
// return the removal of the value at location nextIndex-1.
public void printArray() //accessor
// prints the values of all occupied locations of the array to
// the screen
Your Class must also work with the following Driver Class DynArrayDriver: public class DynArrayDriver
{
public static void main(String[] args)
{
DynArray myArray = new DynArray();
System.out.println("size = " + myArray.arraySize()); System.out.println("elements = " + myArray.elements()); System.out.println(" ");
int pot = 1;
for (int v = 0; v < 10; ++v)
{
myArray.insert(pot);
System.out.println("myArray.at(" + v + ") = " + myArray.at(v)); System.out.println("size = " + myArray.arraySize()); System.out.println("elements = " + myArray.elements() + " "); pot *= 2;
}
System.out.println("myArray.at(10) = " + myArray.at(10)); System.out.println(" ");
for (int v = 0; v < 10; ++v)
{
double value = myArray.remove(); System.out.println("value = " + value); System.out.println("size = " + myArray.arraySize());
System.out.println("elements = " + myArray.elements() + " ");
}
double value = myArray.remove();
System.out.println("value = " + value); System.out.println("size = " + myArray.arraySize()); System.out.println("elements = " + myArray.elements()); System.out.println(" ");
for (int i = 0; i < 5; ++i)
{
myArray.insertAt(i, 3 * i);
System.out.println("myArray.at(" + i + ") = " + myArray.at(i)); System.out.println("size = " + myArray.arraySize()); System.out.println("elements = " + myArray.elements() + " ");
}
myArray.printArray();
System.out.println();
value = myArray.removeAt(2); System.out.println("value = " + value); System.out.println("size = " + myArray.arraySize());
System.out.println("elements = " + myArray.elements() + " "); myArray.printArray();
System.out.println();
value = myArray.removeAt(4); System.out.println("value = " + value); System.out.println("size = " + myArray.arraySize());
System.out.println("elements = " + myArray.elements() + " ");
}
}
|
And produce the following output (or something equivalent):
myArray.at(10) = NaN value = 512.0
size = 16
elements = 9
value = 256.0
size = 16
elements = 8
value = 128.0
size = 8
elements = 7
value = 64.0
size = 8
elements = 6
value = 32.0
size = 8
elements = 5
value = 16.0
size = 8
elements = 4
value = 8.0
size = 4
elements = 3
value = 4.0
size = 4
elements = 2
value = 2.0
size = 2
elements = 1
value = 1.0
size = 1
elements = 0
value = NaN size = 1
elements = 0
myArray.at(0) = 0.0
size = 1
elements = 1
myArray.at(1) = 3.0
size = 2
elements = 2
myArray.at(2) = 6.0
size = 4
elements = 3
myArray.at(3) = 9.0
size = 4
elements = 4
myArray.at(4) = 12.0
size = 8
elements = 5
array.at(0) = 0.0
array.at(1) = 3.0
array.at(2) = 6.0
array.at(3) = 9.0
array.at(4) = 12.0
value = 6.0
size = 8
elements = 4
array.at(0) = 0.0
array.at(1) = 3.0
array.at(2) = 9.0
array.at(3) = 12.0
value = NaN size = 8
elements = 4
Step by Step Solution
There are 3 Steps involved in it
Step: 1
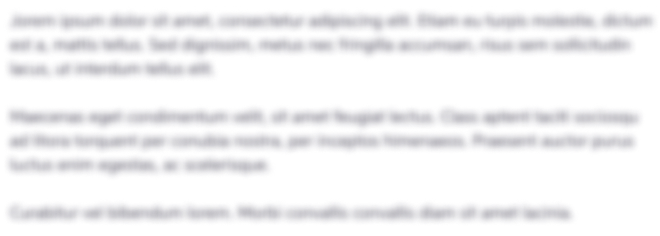
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started