Question
Analyze and tell me how this code works. //FlasCard class to store the Question and Answer public class FlashCard { //Declare data members private String
Analyze and tell me how this code works.
//FlasCard class to store the Question and Answer
public class FlashCard
{
//Declare data members
private String qust;
private String ans;
// constructor of FlashCard()
public FlashCard()
{
qust = "";
ans = "";
}
// getNextQuestion() to retrieve the next question
public String getNextQuestion()
{
return qust;
}
// setNextQuestion() to set the next question
public void setNextQuestion(String qust)
{
this.qust = qust;
}
// getNextAnswer() to find the answer of the current question
public String getNextAnswer()
{
return ans;
}
// setNextAnswer() to set the answer
public void setNextAnswer(String ans)
{
this.ans = ans;
}
}
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import javax.swing.*;
import javax.swing.border.Border;
import javax.swing.border.EtchedBorder;
import javax.swing.border.SoftBevelBorder;
import java.io.*;
import java.util.*;
public class FlashCardGUI extends JFrame implements
ActionListener
{
//declare the required varibles
JButton response;
//To display the answer
JLabel answerDisplay;
//To hold the FlashCard object
ArrayList
//To set the unique questions to be displayed
ArrayList
//To hold the components
JPanel card, FlashCardGame;
//To set the count of questions answered
int count = 0;
//to set the flag values
boolean flag = false;
//To hold the index of the object
int index = 0;
//To the respective values to the respective component
String welcome = "Welcome to Travis's Flash Card app!";
String start = "START THE GAME";
String nextQuestion = "Next Question";
String answer = "Click for Answer";
// constructor
public FlashCardGUI()
{
//main panel to display FlashCardGame
FlashCardGame = new JPanel(new BorderLayout());
// set border for the FlashCardGame panel
FlashCardGame.setBorder(BorderFactory.createCompoundBorder(BorderFactory.createMatteBorder(20, 20, 20,
20, Color.CYAN),BorderFactory.createRaisedSoftBevelBorder()));
//To create the QuestionList objects for the ArrayList
QuestionList = new ArrayList
//To initialize the Boolean array list
bool = new ArrayList
//Intitial the JPanel to hold the components
card = new JPanel(new BorderLayout());
//initialize the JButton
response = new JButton(start);
//set the Font
response.setFont(new Font("Courier New", Font.BOLD, 20));
//set the Border to the button
response.setBorder(BorderFactory.createSoftBevelBorder( SoftBevelBorder.RAISED,
Color.DARK_GRAY, Color.BLUE));
//intitialize the Label
answerDisplay = new JLabel(welcome, SwingConstants.CENTER);
//set the font for the label
answerDisplay.setFont(new Font ("Courier New", Font.BOLD + Font.ITALIC, 16));
//add the label and button to the JPanel
card.add(answerDisplay, BorderLayout.CENTER);
card.add(response, BorderLayout.SOUTH);
//add card to the FlashCardGame
FlashCardGame.add(card, BorderLayout.CENTER);
//add the FlashCardGame to the current container
add(FlashCardGame);
//Action listener to the responseButton
response.addActionListener(this);
//load the FlashCard objects from the text file
readData();
}
// getIndex() this method checks for the uniqueness of the question and then
// retrieves the index which has not been questioned
public int getIndex()
{
//declare the required varibles
int random = 0;
boolean f = false;
//loop until the boolean Values are true
while (!f)
{
//generate the random number
random = (int)(Math.random()*bool.size());
//check whether the values at the index random is
//false or not
//If false, return index
if(bool.get(random)==false)
{
f = true;
return random;
}
}
//if not found return -1;
return -1;
}
//To load the data from the file into FlashCard and add the FlashCard
//Object to the ArrayList
public void readData()
{
//define the required varibles
File file = null;
Scanner input = null;
String question, answer;
FlashCard obj = null;
//place the code inside inside the try..catch block
try
{
//intitialize the file object and scanner object
file = new File("FlashQuestions.txt");
input = new Scanner(file);
//loop until all the values are read from the text file
while (input.hasNextLine())
{
question = input.nextLine();
answer = input.nextLine();
obj = new FlashCard();
obj.setNextQuestion(question);
obj.setNextAnswer(answer);
//Add the FlashCard object to the arraylist
QuestionList.add(obj);
//To set the boolean to false
bool.add(false);
}
//close the Scanner object
input.close();
}
//catch the exception if any
catch(Exception exp)
{
exp.printStackTrace();
}
}
//JButton action event which performs the display the questions
//and answer according to the button click as specified in the question
public void actionPerformed(ActionEvent event)
{
//if the button is clicked
if (event.getSource() == response)
{
//check whethe rthe count is equal to the
//FlashCard array list size
if(count < QuestionList.size())
{
//if lesser,check for the flag values
if(!flag)
{
//if the flag is true then the display the
//question by getting the random value
index = getIndex();
//change the text of te button
response.setText(answer);
bool.set(index, true);
//display the question to the user
answerDisplay.setText(QuestionList.get(index).getNextQuestion());
}
//if the flag is false
else
{
//reset the text of the bottom to other value
response.setText(nextQuestion);
//display the answer
answerDisplay.setText(QuestionList.get(index).getNextAnswer());
//incremenet the count
count++;
}
//toggle the flag value
flag = !flag;
}
//One if all the questions are displayed,
//then to again repeat or go through the questions
else
{
int repeat = JOptionPane.showConfirmDialog(null, "Would you like to try again?",
"Confirmation", JOptionPane.YES_NO_OPTION);
//if they wanna go through the questions again
if(repeat == JOptionPane.YES_OPTION)
{
response.setText(start);
answerDisplay.setText(welcome);
count = 0;
QuestionList = new ArrayList
bool = new ArrayList
readData();
flag = false;
}
//else disable the button
else
{
answerDisplay.setText("Goodbye!");
response.setEnabled(false);
}
}
}
}
public static void main(String args[])
{
// create an object for the FlashCardGUI
FlashCardGUI game = new FlashCardGUI();
// set the title,
game.setTitle("Flash Card Game");
//set the size and visibility close operations
// for the JFrame
game.setSize(1000, 500);
game.setResizable(false);
game.setVisible(true);
//set the close operations for the JFrame
game.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
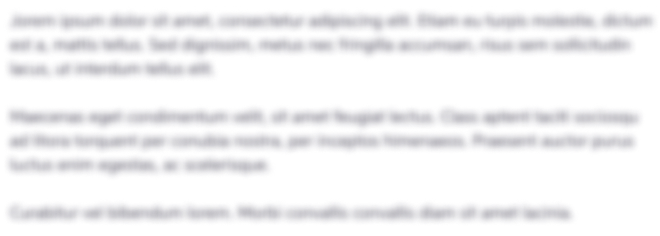
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started