Android Studios:
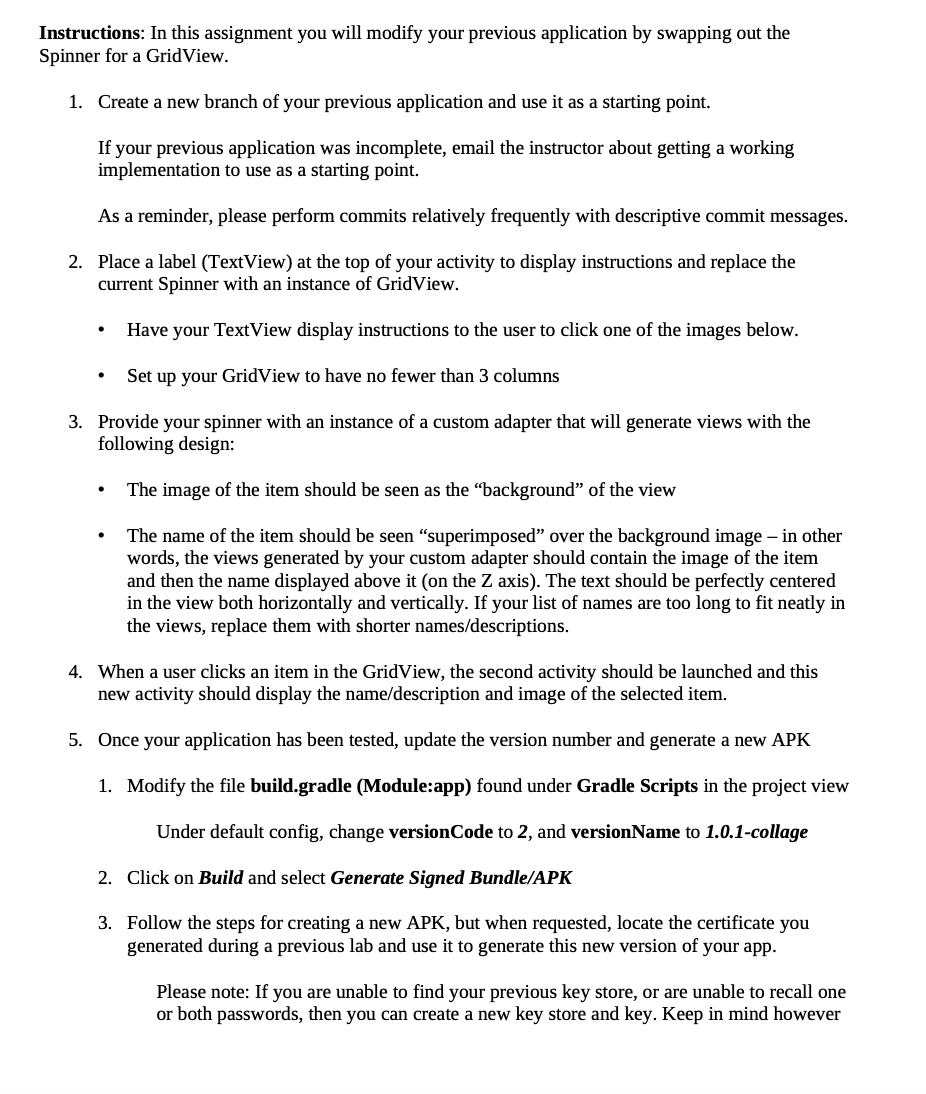
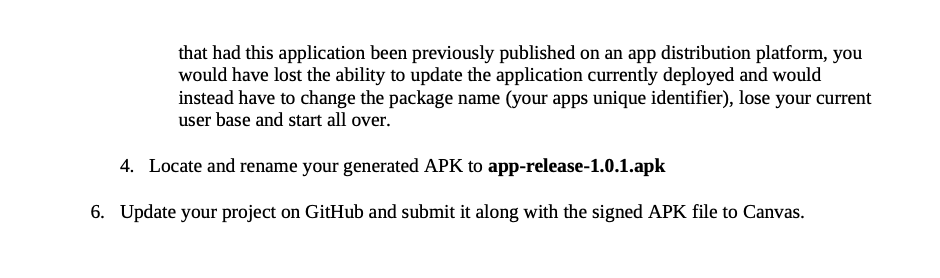
My code:
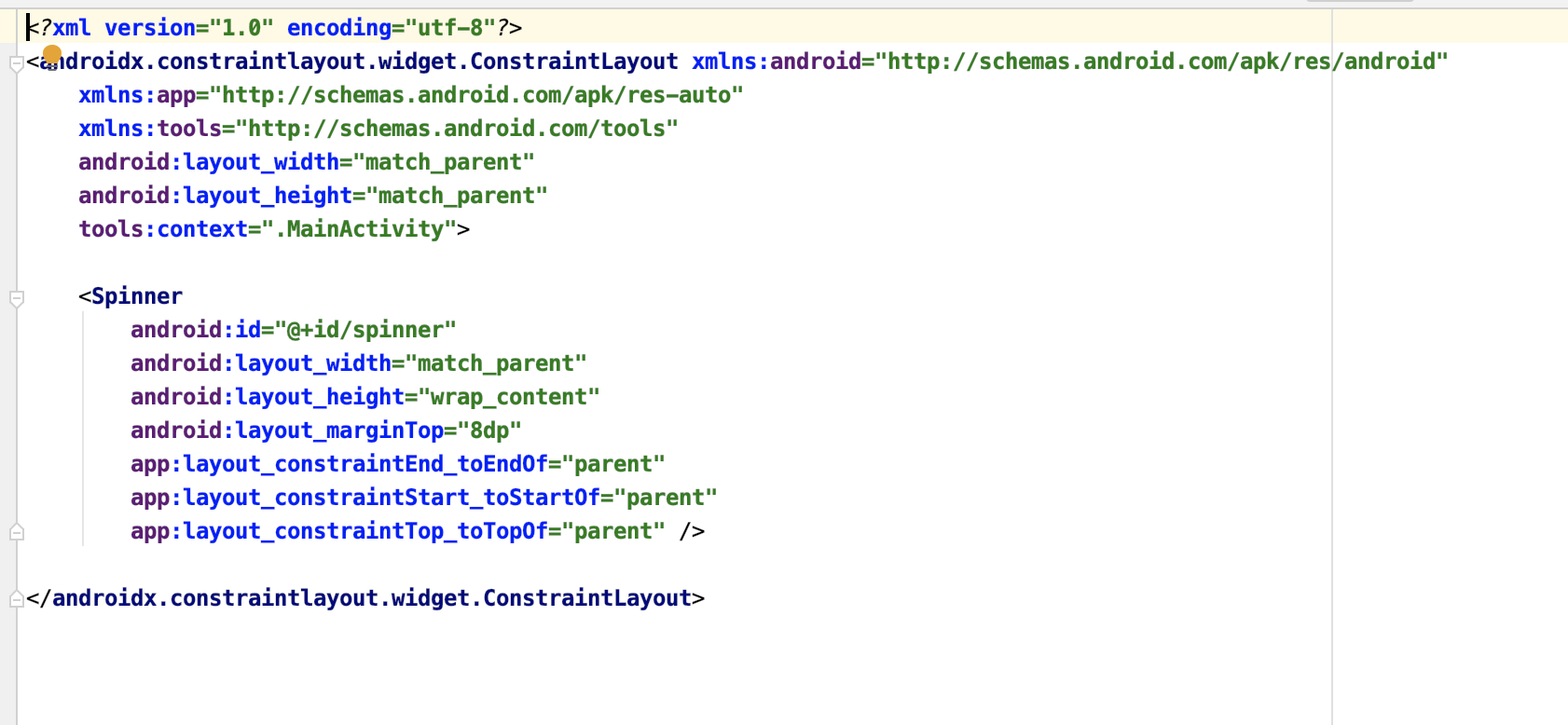
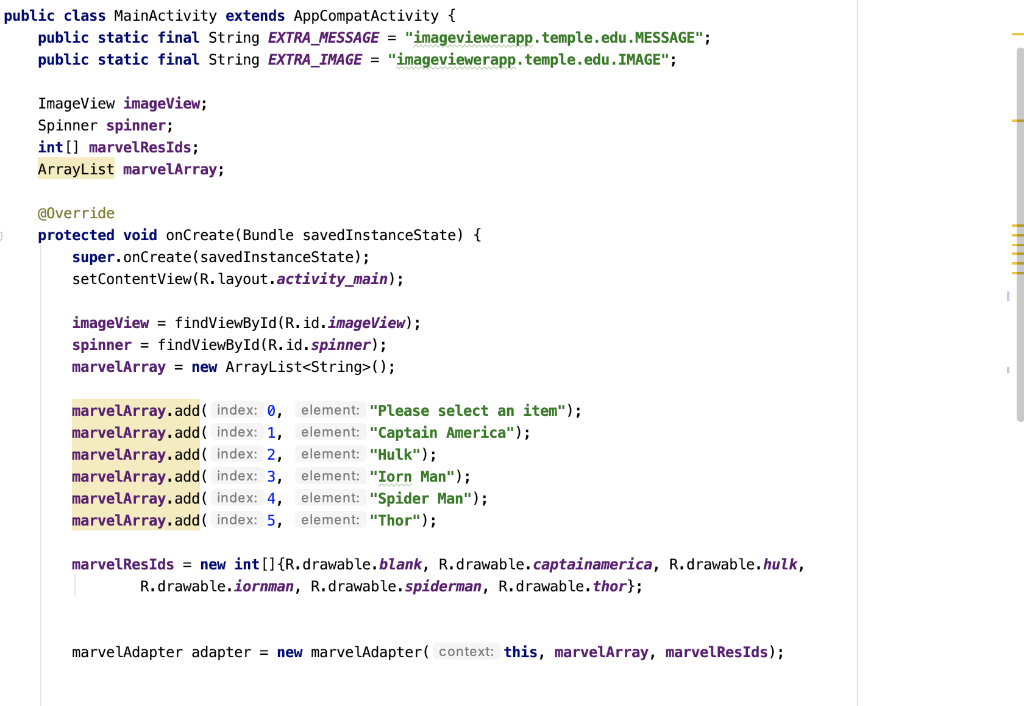
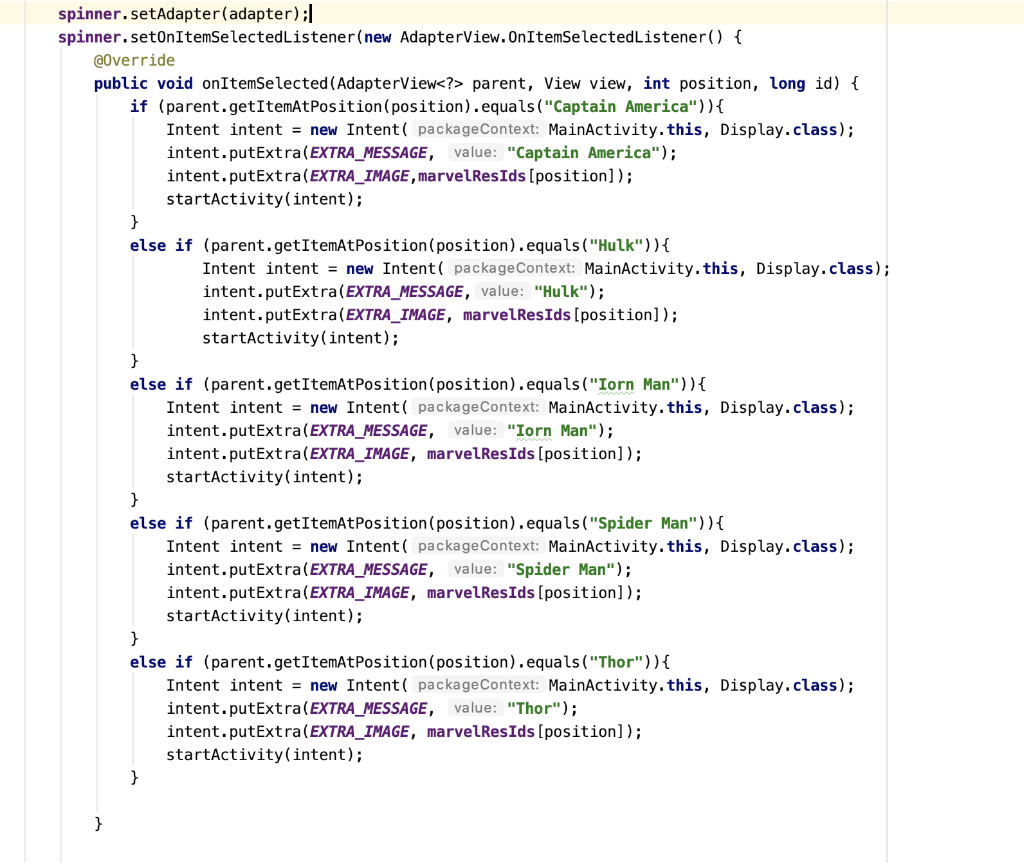
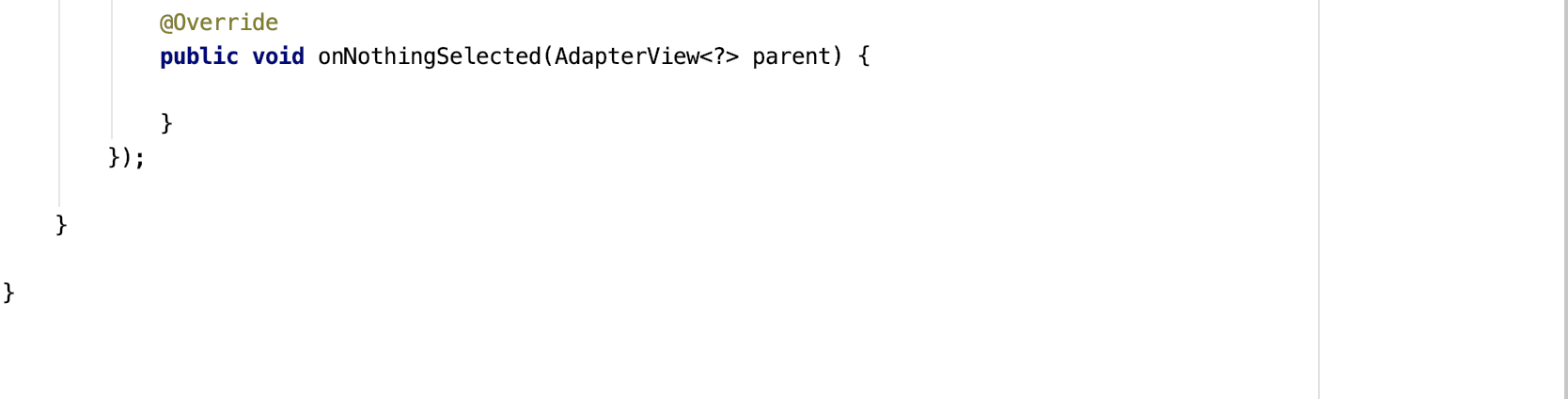
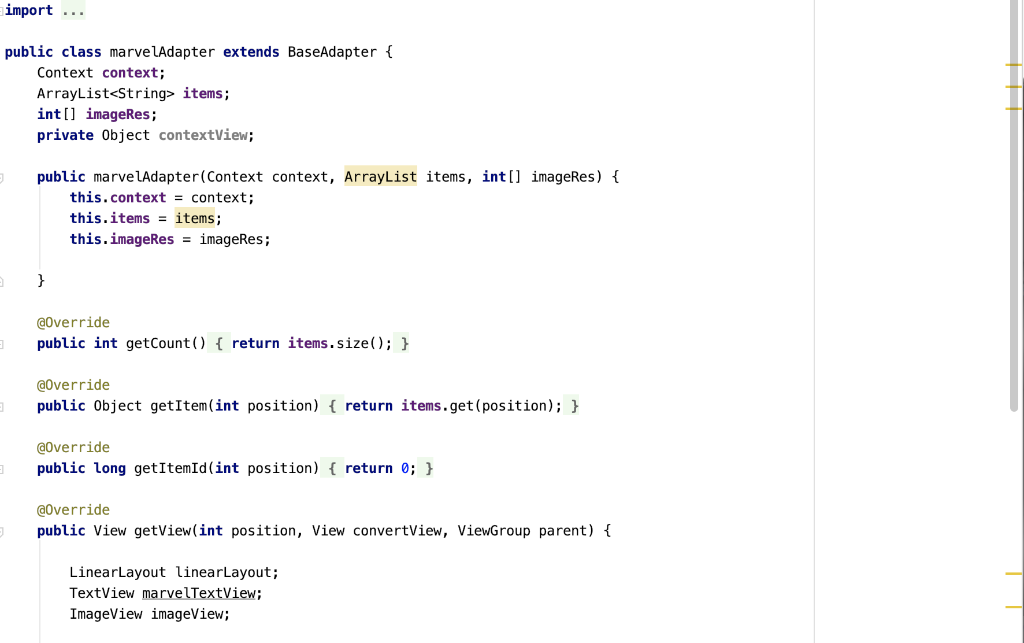
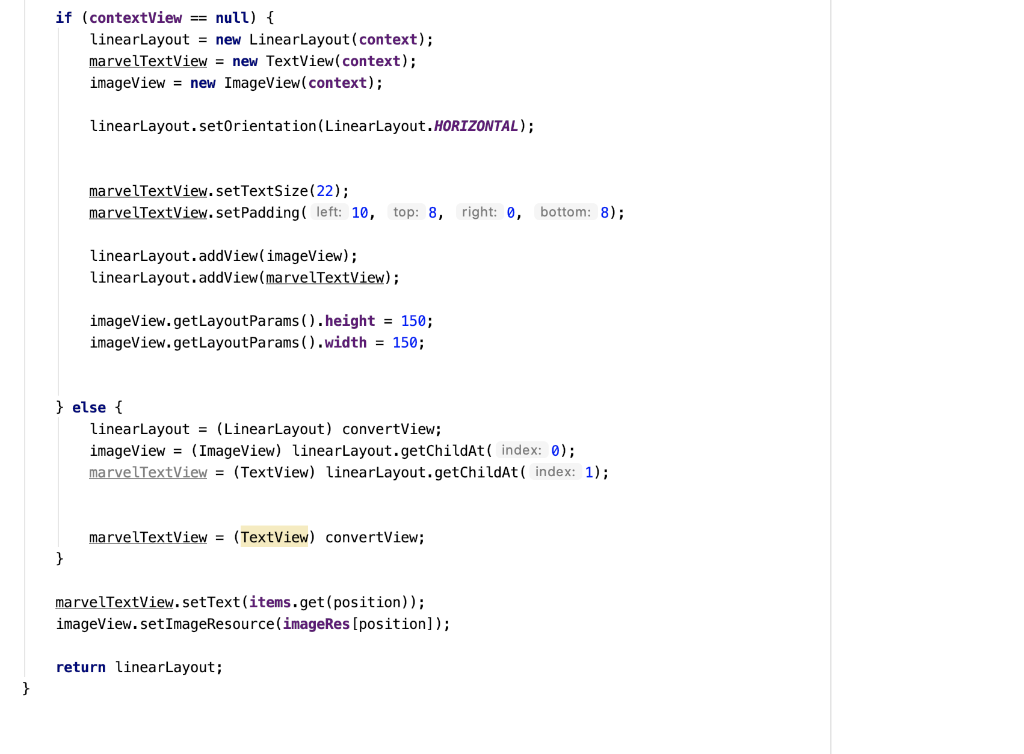
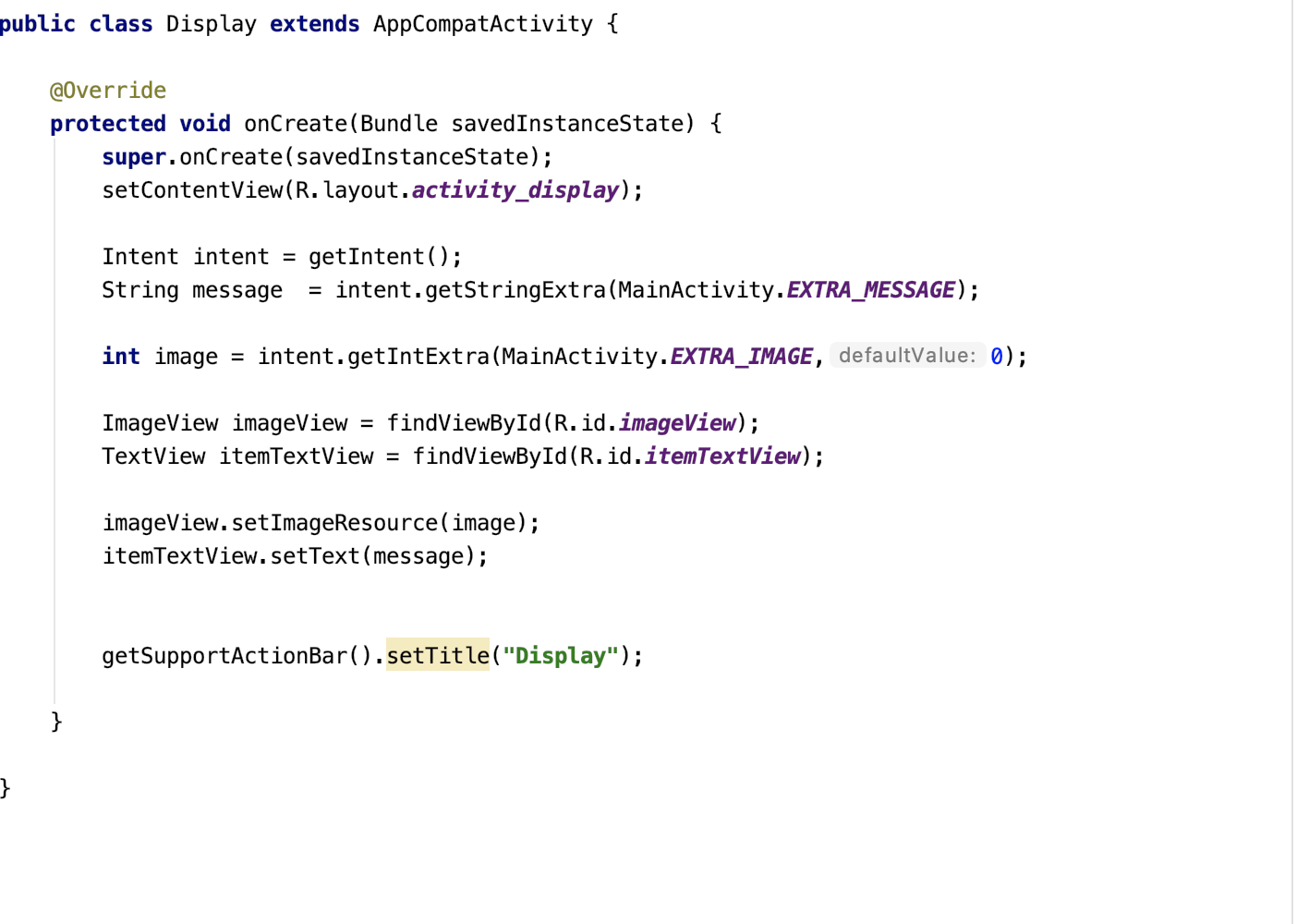
Instructions: In this assignment you will modify your previous application by swapping out the Spinner for a GridView. 1. Create a new branch of your previous application and use it as a starting point. If your previous application was incomplete, email the instructor about getting a working implementation to use as a starting point. As a reminder, please perform commits relatively frequently with descriptive commit messages. 2. Place a label (TextView) at the top of your activity to display instructions and replace the current Spinner with an instance of GridView. Have your TextView display instructions to the user to click one of the images below. . Set up your GridView to have no fewer than 3 columns 3. Provide your spinner with an instance of a custom adapter that will generate views with the following design: The image of the item should be seen as the "background of the view The name of the item should be seen "superimposed over the background image in other words, the views generated by your custom adapter should contain the image of the item and then the name displayed above it on the Z axis). The text should be perfectly centered in the view both horizontally and vertically. If your list of names are too long to fit neatly in the views, replace them with shorter names/descriptions. 4. When a user clicks an item in the GridView, the second activity should be launched and this new activity should display the name/description and image of the selected item. 5. Once your application has been tested, update the version number and generate a new APK 1. Modify the file build.gradle (Module:app) found under Gradle Scripts in the project view Under default config, change versionCode to 2, and version Name to 1.0.1-collage 2. Click on Build and select Generate Signed Bundle/APK 3. Follow the steps for creating a new APK, but when requested, locate the certificate you generated during a previous lab and use it to generate this new version of your app. Please note: If you are unable to find your previous key store, or are unable to recall one or both passwords, then you can create a new key store and key. Keep in mind however that had this application been previously published on an app distribution platform, you would have lost the ability to update the application currently deployed and would instead have to change the package name (your apps unique identifier), lose your current user base and start all over. 4. Locate and rename your generated APK to app-release-1.0.1.apk 6. Update your project on GitHub and submit it along with the signed APK file to Canvas. k?xml version="1.0" encoding="utf-8"?> E
public class MainActivity extends AppCompatActivity { public static final String EXTRA_MESSAGE = "imageviewerapp. temple.edu.MESSAGE"; public static final String EXTRA_IMAGE = "imageviewerapp. temple.edu.IMAGE"; ImageView imageView; Spinner spinner; int[] marvelResIds; ArrayList marvelArray; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate( savedInstanceState); setContentView(R.layout.activity_main); imageView = findViewById(R.id.imageView); spinner = findViewById(R.id. spinner); marvelArray = new ArrayList(); marvelArray.add( index: 0, element: "Please select an item"); marvelArray.add( index: 1, element: "Captain America"); marvelArray.add( index: 2, element: "Hulk"); marvelArray.add( index: 3, element: "Iorn Man"); marvelArray.add( index: 4, element: "Spider Man"); marvelArray.add( index: 5, element: "Thor"); marvelResIds = new int[]{R.drawable.blank, R.drawable.captainamerica, R.drawable. hulk, R.drawable. iornman, R.drawable.spiderman, R.drawable. thor}; marvelAdapter adapter = new marvelAdapter( context: this, marvelArray, marvelResIds); spinner.setAdapter(adapter);|| spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected (AdapterView> parent, View view, int position, long id) { if (parent.getItemAtPosition (position).equals("Captain America")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Captain America"); intent.putExtra(EXTRA_IMAGE, marvelResIds [position]); startActivity(intent); } else if (parent.getItemAt Position (position).equals("Hulk")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Hulk"); intent.putExtra(EXTRA_IMAGE, marvelResIds [position]); startActivity(intent); } else if (parent.getItemAt Position (position).equals("Iorn Man")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Iorn Man"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity(intent); } else if (parent.getItemAtPosition (position).equals("Spider Man")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Spider Man"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity (intent); } else if (parent.getItemAt Position (position).equals("Thor")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Thor"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity(intent); } } @Override public void onNothingSelected (AdapterView> parent) { } }); } } import ... public class marvelAdapter extends BaseAdapter { Context context; ArrayList items; int[] imageRes; private object contextView; public marvelAdapter(Context context, ArrayList items, int[] imageRes) { this.context = context; this. items = items; this imageRes = imageRes; } @Override public int getCount() { return items.size(); } @Override public Object getItem(int position) { return items.get(position); } @Override public long getItemId(int position) { return 0; } @Override public View getView(int position, View convertView, ViewGroup parent) { LinearLayout linearLayout; TextView marvelTextView; ImageView imageView; if (contextView == null) { linearLayout = new LinearLayout(context); marvelTextView = new TextView( context); imageView = new ImageView(context); linearLayout.setOrientation (LinearLayout.HORIZONTAL); marvelTextView.setTextSize(22); marvelTextView.setPadding( left: 10, top: 8, right: 0, bottom: 8); linearLayout.addView(imageView); linearLayout.addView(marvelTextView); imageView.getLayoutParams ().height = 150; imageView.getLayoutParams ().width = 150; } else { linearLayout = (LinearLayout) convertView; imageView = (ImageView) linearLayout.getChildAt( index: 0); marvelTextView = (TextView) linearLayout.getChildAt( index: 1); marvelTextView = (TextView) convertView; } marvelTextView.setText(items.get(position)); imageView.setImageResource(imageRes (position]); return linearLayout; } public class Display extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R. layout.activity_display); Intent intent = String message getIntent(); intent.getStringExtra(MainActivity.EXTRA_MESSAGE); = int image intent.getIntExtra (MainActivity.EXTRA_IMAGE, defaultValue: 0); ImageView imageView = findViewById(R.id.imageView); TextView itemTextView = findViewById(R.id.itemTextView); imageView.setImageResource(image); itemTextView.setText(message); getSupportActionBar().setTitle("Display"); } } Instructions: In this assignment you will modify your previous application by swapping out the Spinner for a GridView. 1. Create a new branch of your previous application and use it as a starting point. If your previous application was incomplete, email the instructor about getting a working implementation to use as a starting point. As a reminder, please perform commits relatively frequently with descriptive commit messages. 2. Place a label (TextView) at the top of your activity to display instructions and replace the current Spinner with an instance of GridView. Have your TextView display instructions to the user to click one of the images below. . Set up your GridView to have no fewer than 3 columns 3. Provide your spinner with an instance of a custom adapter that will generate views with the following design: The image of the item should be seen as the "background of the view The name of the item should be seen "superimposed over the background image in other words, the views generated by your custom adapter should contain the image of the item and then the name displayed above it on the Z axis). The text should be perfectly centered in the view both horizontally and vertically. If your list of names are too long to fit neatly in the views, replace them with shorter names/descriptions. 4. When a user clicks an item in the GridView, the second activity should be launched and this new activity should display the name/description and image of the selected item. 5. Once your application has been tested, update the version number and generate a new APK 1. Modify the file build.gradle (Module:app) found under Gradle Scripts in the project view Under default config, change versionCode to 2, and version Name to 1.0.1-collage 2. Click on Build and select Generate Signed Bundle/APK 3. Follow the steps for creating a new APK, but when requested, locate the certificate you generated during a previous lab and use it to generate this new version of your app. Please note: If you are unable to find your previous key store, or are unable to recall one or both passwords, then you can create a new key store and key. Keep in mind however that had this application been previously published on an app distribution platform, you would have lost the ability to update the application currently deployed and would instead have to change the package name (your apps unique identifier), lose your current user base and start all over. 4. Locate and rename your generated APK to app-release-1.0.1.apk 6. Update your project on GitHub and submit it along with the signed APK file to Canvas. k?xml version="1.0" encoding="utf-8"?> E public class MainActivity extends AppCompatActivity { public static final String EXTRA_MESSAGE = "imageviewerapp. temple.edu.MESSAGE"; public static final String EXTRA_IMAGE = "imageviewerapp. temple.edu.IMAGE"; ImageView imageView; Spinner spinner; int[] marvelResIds; ArrayList marvelArray; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate( savedInstanceState); setContentView(R.layout.activity_main); imageView = findViewById(R.id.imageView); spinner = findViewById(R.id. spinner); marvelArray = new ArrayList(); marvelArray.add( index: 0, element: "Please select an item"); marvelArray.add( index: 1, element: "Captain America"); marvelArray.add( index: 2, element: "Hulk"); marvelArray.add( index: 3, element: "Iorn Man"); marvelArray.add( index: 4, element: "Spider Man"); marvelArray.add( index: 5, element: "Thor"); marvelResIds = new int[]{R.drawable.blank, R.drawable.captainamerica, R.drawable. hulk, R.drawable. iornman, R.drawable.spiderman, R.drawable. thor}; marvelAdapter adapter = new marvelAdapter( context: this, marvelArray, marvelResIds); spinner.setAdapter(adapter);|| spinner.setOnItemSelectedListener(new AdapterView.OnItemSelectedListener() { @Override public void onItemSelected (AdapterView> parent, View view, int position, long id) { if (parent.getItemAtPosition (position).equals("Captain America")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Captain America"); intent.putExtra(EXTRA_IMAGE, marvelResIds [position]); startActivity(intent); } else if (parent.getItemAt Position (position).equals("Hulk")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Hulk"); intent.putExtra(EXTRA_IMAGE, marvelResIds [position]); startActivity(intent); } else if (parent.getItemAt Position (position).equals("Iorn Man")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Iorn Man"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity(intent); } else if (parent.getItemAtPosition (position).equals("Spider Man")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Spider Man"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity (intent); } else if (parent.getItemAt Position (position).equals("Thor")) { Intent intent = new Intent( package Context: MainActivity. this, Display.class); intent.putExtra(EXTRA_MESSAGE, value: "Thor"); intent.putExtra(EXTRA_IMAGE, marvelResIds (position]); startActivity(intent); } } @Override public void onNothingSelected (AdapterView> parent) { } }); } } import ... public class marvelAdapter extends BaseAdapter { Context context; ArrayList items; int[] imageRes; private object contextView; public marvelAdapter(Context context, ArrayList items, int[] imageRes) { this.context = context; this. items = items; this imageRes = imageRes; } @Override public int getCount() { return items.size(); } @Override public Object getItem(int position) { return items.get(position); } @Override public long getItemId(int position) { return 0; } @Override public View getView(int position, View convertView, ViewGroup parent) { LinearLayout linearLayout; TextView marvelTextView; ImageView imageView; if (contextView == null) { linearLayout = new LinearLayout(context); marvelTextView = new TextView( context); imageView = new ImageView(context); linearLayout.setOrientation (LinearLayout.HORIZONTAL); marvelTextView.setTextSize(22); marvelTextView.setPadding( left: 10, top: 8, right: 0, bottom: 8); linearLayout.addView(imageView); linearLayout.addView(marvelTextView); imageView.getLayoutParams ().height = 150; imageView.getLayoutParams ().width = 150; } else { linearLayout = (LinearLayout) convertView; imageView = (ImageView) linearLayout.getChildAt( index: 0); marvelTextView = (TextView) linearLayout.getChildAt( index: 1); marvelTextView = (TextView) convertView; } marvelTextView.setText(items.get(position)); imageView.setImageResource(imageRes (position]); return linearLayout; } public class Display extends AppCompatActivity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R. layout.activity_display); Intent intent = String message getIntent(); intent.getStringExtra(MainActivity.EXTRA_MESSAGE); = int image intent.getIntExtra (MainActivity.EXTRA_IMAGE, defaultValue: 0); ImageView imageView = findViewById(R.id.imageView); TextView itemTextView = findViewById(R.id.itemTextView); imageView.setImageResource(image); itemTextView.setText(message); getSupportActionBar().setTitle("Display"); } }