Question
Answer in C language, add comments to code, three files included main.c, Stringstr.c, Stringstr.h each file indicated in bold Provide screenshot of implemented code and
Answer in C language, add comments to code, three files included main.c, Stringstr.c, Stringstr.h each file indicated in bold
Provide screenshot of implemented code and output, provide 2 tests in main.c for SStr_SetAt()
//Stringstr.h file contents
#ifndef STRING_STR #define STRING_STR
typedef struct StringString { // This holds the length of the string int length;
// pointer to the raw data // data is a pointer to an array of characters (c-string) // note that the size of the array data points to is 1 longer // than 'length', because it will hold the terminating char char *data; } SSTRING;
SSTRING *SStr_Create(char *c_str);
void SStr_Delete(SSTRING *str);
// Print function for StringString void SStr_Print(char *Sstr_name, SSTRING *str);
// Return the length of the StringString int SStr_GetLength(SSTRING *str);
// Return the character at index 'index' in the StringString str // Do bounds checking, print an error and return -1 if the index is not valid // for the string char SStr_GetAt(SSTRING *str, int index);
// Change the str at index 'index' to the char char_to_set // Bounds checking and print an error and do nothing else if the index is not // valid for the string void SStr_SetAt(SSTRING *str, int index, char char_to_set);
// Create a new StringString* from existing StringString, str_to_copy // Must be deep copy SSTRING *SStr_Copy(SSTRING *str_to_copy);
#endif
//Stringstr.c contents
#include "Stringstr.h" #include
SSTRING *SStr_Create(char *str_to_copy) { SSTRING *new_s = (SSTRING *)malloc(sizeof(SSTRING));
new_s->length = strlen(str_to_copy);
// Allocate data array dynamically, Add one to data length for string terminator new_s->data = (char *)malloc(new_s->length + 1);
// Copy data over from str_to_copy for (int i = 0; i < new_s->length; i++) { new_s->data[i] = str_to_copy[i]; } new_s->data[new_s->length] = '\0'; return new_s; }
void SStr_Delete(SSTRING *str) { free(str->data); free(str); }
void SStr_Print(char *Sstr_name, SSTRING *str) { printf("StringString %s: { len: %d, data: %s } ", Sstr_name, str->length, str->data); } //ADD THE FOLLOWING FUNCTIONS
//Return the length of the StringString //int SStr_GetLength(SSTRING *str);
// Return the character at index 'index' in the StringString str // Do bounds checking, print an error and return -1 if the index is not valid for the string // char SStr_GetAt(SSTRING *str, int index){ // Change the str at index 'index' to the char char_to_set // Do bounds checking and print an error and do nothing else if the index is not valid for the string /*void SSTR_SetAt(SSTRING *str, int index, char char_to_set){ //do deep copy
} */ // Create a new StringString* from an existing StringString, str_to_copy // Be sure to do a deep copy /*SSTRING *SStr_Copy(SSTRING* str_to_copy){
} */
//main.c contents
#include "Stringstr.h" #include
/* Functions implemented: Create, Delete, Print
Additional functions for GetLength(), GetAt(), SetAt(), and Copy() are declared in the header file. Complete the implementation of following functions in Stringstr.c file
Tests have been provided for SStr_GetLength(), SStr_GetAt(), and SStr_Copy(). Add functions and enable theappropriate tests. Confirm that these tests pass, and ADD (at least) 2 additional tests to test the SStr_SetAt() function. Tests can be print statements. One of the tests should be a valid index and one should be an invalid index.
FUNCTIONS TO ADD:
Return the length of the StringString int SStr_GetLength(SSTRING *str);
Return the character at index 'index' in the SancyString str Do bounds checking, print an error and return -1 if the index is not valid for the string char SStr_GetAt(SSTRING *str, int index);
Change the str at index 'index' to the char char_to_set Do bounds checking and print an error and do nothing else if the index is not valid for the string void SStr_SetAt(SSTRING *str, int index, char char_to_set);
Create a new StringString* from an existing StringString, str_to_copy. Must be a deep copy. Return NULL if an error occurs. SSTRING *SStr_Copy(SSTRING*str_to_copy);
TESTS TO ADD: 2 tests for SStr_SetAt(), one for valid index and one for invalid index */
int main(void) {
printf("SStr_Create() "); char *c_str1 = "Excited Snails"; SSTRING *f_str1 = SStr_Create(c_str1); SStr_Print("f_str1", f_str1);
char *c_str2 = "template for!!"; SSTRING *f_str2 = SStr_Create(c_str2); SStr_Print("f_str2", f_str2);
// int SStr_GetLength(SSTRING *str);
// Run when GetLength() is defined /* printf(" SStr_GetLength() "); int lens1 = SStr_GetLength(f_str1); printf("f_str1 length: %d ", lens1);
// LENGTH TESTS, do not change int fstr1_lens = SStr_GetLength(f_str1); if (fstr1_lens != strlen(c_str1)) { printf("SStr_GetLength() test FAILED, got length %d, wanted %lu ", fstr1_len, strlen(c_str1)); } int emptyLens = SStr_GetLength(SStr_Create("")); if (emptyLens != 0) { printf("SStr_GetLength() test FAILED, got length %d, wanted %d ", emptyLens, 0); } */ // Run when GetAt() is defined /* printf(" SStr_GetAt() "); // GETAT() TESTS, do not change char c1 = SStr_GetAt(f_str1, 0); if (c1 != c_str1[0]) { printf("SStr_GetAt() test FAILED, got char %c, wanted %c ", c1, c_str1[0]); } int lastInd = strlen(c_str1) - 1; c1 = SStr_GetAt(f_str1, lastInd); if (c1 != c_str1[lastInd]) { printf("SStr_GetAt() test FAILED, got char %c, wanted %c ", c1, c_str1[lastInd]); } int badIndex = lastInd + 5; c1 = SStr_GetAt(f_str1, badIndex); if (c1 != -1) { printf("SStr_GetAt() test FAILED, got char %c, wanted %c ", c1, c_str1[badIndex]); } */ /* Run when Copy() is defined
printf(" SStr_Copy() "); printf("Copy fstr2 to fstr1 "); f_str1 = SStr_Copy(f_str2); SStr_Print("f_str1", f_str1);
// COPY TESTS, do not change if (f_str1->length != strlen(c_str2)) { printf(" SStr_Copy() test FAILED, got f_str1->length %d, wanted %lu ", f_str1->length, strlen(c_str2)); } if (strcmp(f_str1->data, c_str2) != 0) { printf( " SStr_Copy() test FAILED, got f_str1->data \"%s\", wanted \"%s\" ", f_str1->data, c_str2); }
char str2_cpy[100]; strcpy(str2_cpy, c_str2); c_str2 = "Exit"; printf("Changed c_str2 to %s ", c_str2); printf("StringString f_str1 shouldn't have changed "); SStr_Print("f_str1", f_str1);
if (strcmp(f_str1->data, str2_cpy) != 0) { printf( " SStr_Copy() test FAILED, got f_str1->data \"%s\", wanted \"%s\" ", f_str1->data, str2_cpy); } */
printf(" SStr_Delete() "); SStr_Delete(f_str1); SStr_Delete(f_str2);
return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
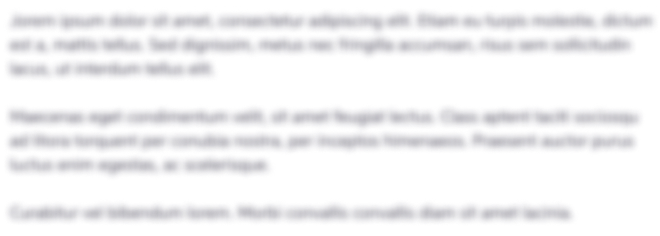
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started