Question
Answer using C++ please. The builders.cpp (a file I provide) runs the main loop for the program. This main loop repeatedly goes through two phases:
Answer using C++ please.
The builders.cpp (a file I provide) runs the main loop for the program. This main loop repeatedly goes through two phases: dispatch and work. During the dispatch phase, the main loop reads building requests from a datafile and sends those requests to the appropriate builder. The dispatch phase only sends a limited number of requests per each main loop iteration. During the work phase the main loop gives each builder a chance to build one item from its own work list. The main loop keeps iterating until there are no more building requests (i.e., we've reached the end of the datafile) AND all the builder objects have finished processing all their pending requests.
After the loop ends, the main program sends each builder a request to return back to the base.
Each builder receives commands to build certain structures in certain sectors. The builder receives commands via the addRequest() method. The requests are stored in a linked list in the builder. New requests must be placed at the front of the list. Each request specifies a sector and type of structure to build. The builder doesn't build the structure as soon as it gets the request, instead the builder stores the request in the linked list so that it can be built later. Whenever the builder receives a request, it should print out a message in the following format:
Builder #5: Received request to build a solar energy collector in sector 21
Each builder also is given a chance, once per main loop iteration in builders.cpp, to do some work. This happens when doCycle() is called. When doCycle() is called on the builder, the builder must process the oldest building request and create that structure. Note that since all requests are added to the front of the list, the oldest request is at the back of the list so the builder should remove items from the back of the list. If the structure is to be created in the same sector in which the previous structure for that builder was built, then just build it. Otherwise, the builder must travel to the new sector first. Traveling to sectors and building structures are indicated by printing messages as follows:
Builder #2: Moving to sector 25 Builder #2: Building a water harvester in sector 25
When doCycle() is called, it is possible that there are no pending requests for a builder (that is, the linked list is empty). In that case the builder should do nothing. The doCycle() method should return 'true' if any structures are built and 'false' otherwise. This return value is used by the main program to determine when all builders are done doing work.
Finally, when the main program has determined that all work is complete, it will request each builder to return home by calling the returnHome() method. When this happens, the builder should backtrack through the list of structures that it built, in reverse order. The builder is connecting the structures together so that the water or energy that the structure harvests is delivered to the base station. While returning the builder should print messages to the screen as follows:
Builder #1: Connected to water harvester in sector 50 Builder #1: Connected to water harvester in sector 50 Builder #1: Moving to sector 10 Builder #1: Connected to solar energy collector in sector 10 Builder #1: Moving to sector 23 Builder #1: Connected to wind turbine in sector 23
I have provided two files, structuretype.h/cpp, that define an enumerated type
(enum) for the different structure types.
- builder -- This class represents a builder. Each builder has a builder number (an integer) and a stack and a linked list. You are free to add additional data members as needed. Each builder also has the addRequest(), doCycle(), and returnHome() methods that work as described above.
- stack -- This class implements a stack. This should be implemented using a linked list.
- linkedlist -- This class implements a linkedlist that allows items to be inserted at the front of the list and removed from the back.
- event -- This class is a simple encapsulation of an "event". That is, a sector and building type to be built.
builders.cpp
#include
#include
#include
#include "structuretype.h"
#include "builder.h"
using namespace std;
#define MAX_COMMAND_LENGTH 50
#define COMMANDS_PER_CYCLE 5
int main(int argc, char** argv) {
if (argc != 2) {
cout "
return(0);
}
// Read the data
char* datafile = argv[1];
ifstream infile(datafile);
int builderNum;
int sectorNum;
char typeStr[MAX_COMMAND_LENGTH];
// The first line of the data file contains the number of builders in the format:
// numbuilders x
// We can ignore the text "numbuilders" since we only want the x
char junk[MAX_COMMAND_LENGTH];
int numBuilders;
builder* builders;
infile >> junk; // read and discard the text "numbuilders"
infile >> numBuilders;
builders = new builder[numBuilders];
for (int i=0;i { builders[i].setBuilderNum(i+1); } int cycle = 1; bool doneReadingData = false; bool didSomething = false; bool done = false; while (!done) { cout cout cout cout cycle++; // Process a number of incoming commands cout cout if (!doneReadingData) { for (int i=0;i { infile >> builderNum; infile >> sectorNum; infile >> typeStr; if (infile.eof()) { doneReadingData = true; } else { structure_type type = getFromString(typeStr); builders[builderNum-1].addRequest(sectorNum,type); } } } else { cout } cout // Each builder gets a cycle to do work if it has any didSomething = false; cout cout for (int i=0;i { if (builders[i].doCycle()) { didSomething = true; } } if (!didSomething) { cout } cout // We're done with the loop when there is no more data and none // of the builders did anything during the last cycle. done = (doneReadingData && !didSomething); } // Have each builder return to the base cout cout for (int i=0;i { builders[i].returnHome(); } cout delete [] builders; } structuretype.cpp #include #include "structuretype.h" structure_type getFromString(const char* str) { structure_type ret = WATER; if (strcmp(str,"wind")==0) { ret = WIND; } if (strcmp(str,"water")==0) { ret = WATER; } if (strcmp(str,"solar")==0) { ret = SOLAR; } return ret; } void toString(const structure_type type,char* str) { switch (type) { case WIND: strcpy(str,"wind"); break; case WATER: strcpy(str,"water"); break; case SOLAR: strcpy(str,"solar"); break; } } const char* str(const structure_type type) { const char* ptr; switch (type) { case WIND: ptr = "wind turbine"; break; case WATER: ptr = "water harvester"; break; case SOLAR: ptr = "solar energy collector"; break; } return ptr; } structuretype.h #ifndef STRUCTURETYPE_H #define STRUCTURETYPE_H enum structure_type {WIND, WATER, SOLAR,UNK}; structure_type getFromString(const char* str); void toString(const structure_type type,char* str); const char* str(const structure_type type); #endif sample input numbuilders 100 58 9740 water 58 9740 solar 81 3198 wind 20 9097 wind 28 9990 wind 63 5762 solar 63 5762 water 63 5762 wind 100 1198 solar 100 1198 wind 10 4516 water 10 4516 solar 10 4516 solar 45 5323 solar 47 6658 solar 47 6658 water sample output Take as long as you need. I would appreciate if this could be done by this week. If you have any questions, feel free to comment. Thank you in advance!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
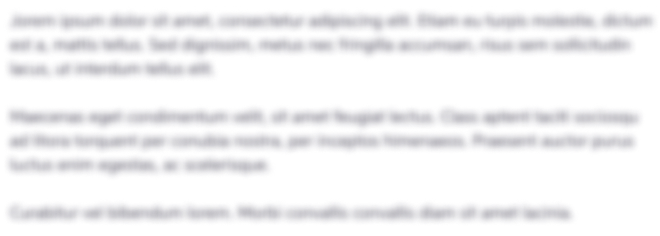
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started