Question
Any help with this Java program would be appreciated! In this lab, we'll implement an ArrayList class but only for Integer objects. This class will
Any help with this Java program would be appreciated!
In this lab, we'll implement an ArrayList class but only for Integer objects. This class will contain the following methods:
//add element to end of list
void add(int element);
//add element at specified index
void add(int index, int element);
//return element at specified index
int get (int index);
//remove element at specified index
//"squish" down the array
int remove( int index);
//return the index of the specified element
int indexOf( int element);
//set the element at the specified index
//to the given element. Return the overwritten element
int set( int index, int element);
//return the number of elements currently stored
int size( );
//Allow the array to be printed
String toString();
To implement the ArrayList, we'll need an array of integers and an index of where we will store the next element.
When we add an integer without a specified index, it implies that we add it to the end of the current list. We'll store the value at next and then increment the next index by 1.
If we add an integer at a specified index (less than next), we'll first shift all elements from index to next-1 to the right, and then store the element at index. For example, we have a 7 element array with 5 elements:
The command ref.add(2, 8) will first shift all elements from 2 to next-1 to the right, store the 8 into the element at index 2, and then increment the next index.
The shift right will consist of 3 moves, starting at next-1:
After the 3 moves, the 8 is put into the element at index 2 and the next index is incremented:
To remove an element at a specific location, you'll need to save the element at index, then shift all elements from index+1 to next-1 to the left, and finally decrement the next index. For example, to remove the element at index 3, we would call: ref.remove(3). Starting with the array above:
Save the element, 2, into a temp variable, and then shift the elements starting at index+1 to the left:
After the shift, decrement the next index, and the method returns the value removed (2).
Note: the next index is where we will insert the next element. With the figure above, the element at next (the 1 at index 5) is not in the ArrayList. The element is considered "dirty" and should be cleared. In the actual ArrayList class, only references are stored and by clearing the "dirty" reference, it speeds up the JVM garbage collection process.
The method size() returns the current number of elements in the array, which is the value of next. In the above case, size() returns 5. We have 5 elements in the array.
When adding an element, it is possible for the static array to fill up. We detect this condition when next is equal to the length of the static array. In this case, create a new array that istwice the length of the static array, copy all elements, and then set the reference of the internal array to the new array. For example, let's say our current static array (ref is arr) is full, so next is equal to arr.length:
Create a new array, nArr, that is twice the length of arr:
Copy all elements from arr to nArr:
Then set the internal reference, arr, to nArr:
You should always check for a full array before adding an element, with or without a specified index.
Implementing the ArrayListInteger Class, Steps:
- Create a class, ArrayListInteger
- Add your instance variables, which will include a reference to an integer array and an integer index, next:
private int [ ] arr;
private int next = 0;
3. Create a 1 argument constructor, that accepts an integer size, which allows you to specify the initial length of the array, arr.
4. Create a default constructor that will create an initial array size of 10 elements.
5. Implement all the methods specified.
In your methods, if you detect an illegal operation, display the string "Exception: (state the reason), ignoring operation".
For example, if you tried to add an element at a specific index that is greater than next, the array would not be changed and the following message would be displayed: "Exception: invalid index - ignoring add"
6. UseTestMyArray.java to test your implementation.
My code (unfinished):
public class ArrayListInteger { private int[] arr; private int next = 0;
public int[] arr(int size) {
return arr;
}
public ArrayListInteger() { arr = new int[10]; }
// add element to end of list public void add(int element) { if (next == arr.length) { b = arr; }
}
// add element at specified index public void add(int index, int element) {
}
// return element at specified index public int get(int index) { int a = arr[index]; return a; }
// remove element at specified index // "squish" down the array public int remove(int index) { }
// return the index of the specified element public int indexOf(int element) {
}
// set the element at the specified index //to the given element. Return the overwritten element public int set(int index, int element) { int over = arr[index]; arr[index] = element; return over; }
//return the number of elements currently stored public int size() { return next; }
//Allow the array to be printed public String toString() { System.out.println(); return arr; }
}
TestMyArray.java Code:
public class TestMyArray {
/** * @param args */
// note, this method tests the ArrayList size() method public static void printArray(String msg, ArrayListInteger a) // public static void printArray(String msg, ArrayList a) { if (msg != null) System.out.println(msg + ":");
for (int k = 0; k < a.size(); k++) { System.out.printf("%5d,", a.get(k)); if ((k + 1) % 10 == 0) System.out.println(); } System.out.println(); }
public static void printTestHeader(int testNum, String msg) { System.out.println(" ================================================================="); System.out.println("Test: " + testNum + ". " + msg + " "); }
public static void printTestTrailer() { System.out.println("================================================================= "); }
public static void main(String[] args) { int testCount = 1; int element = 0;
/* * PLEASE READ: uncomment each test as you go to simplify debugging. Note * printArray calls the ArrayList methods: get(index) and size(), so start off * implementing the constructors, size, get, and add. * * After you complete all tests, comment out the line where we create an * ArrayListInteger and uncomment out the line where we create an * ArrayList. Do the same thing in printArray. Verify you get the same * test results. */ printTestHeader(testCount++, "create an ArrayList, add 2 elements, then call size()"); ArrayListInteger myList = new ArrayListInteger(); myList.add(32); myList.add(16); int size = myList.size(); System.out.println("size of array is: " + size); printArray("myList", myList); printTestTrailer();
printTestHeader(testCount++, "test get(index)"); element = myList.get(0); System.out.println("element at 0: " + element); element = myList.get(1); System.out.println("element at 1: " + element); printTestTrailer();
printTestHeader(testCount++, "add several more elements forcing a call to expandArray()"); for (int k = 0; k < 10; k++) myList.add(k + 5); printArray(null, myList); printTestTrailer();
printTestHeader(testCount++, "test set method, set 1st element to 100, middle to 555 and last to 999 "); element = myList.set(0, 100); System.out.println("previous value at 0 was: " + element); int middle = (myList.size() - 1) / 2; element = myList.set(middle, 555); System.out.println("previous value at " + middle + " was: " + element); element = myList.set(myList.size() - 1, 999); System.out.println("previous value at last index was: " + element); printArray(null, myList); printTestTrailer();
printTestHeader(testCount++, "test indexOf method, find specified element at which index "); printArray(null, myList); int index = myList.indexOf(12); System.out.println("found element 12 at: " + index); index = myList.indexOf(100); System.out.println("found element 100 at: " + index); index = myList.indexOf(999); System.out.println("found element 999 at: " + index); index = myList.indexOf(123); System.out.println("found element 123 at: " + index); printTestTrailer();
printTestHeader(testCount++, "test add (int index, Integer element) "); printArray(null, myList);
System.out.println("adding 1111 at index 0: "); myList.add(0, 1111); printArray(null, myList);
System.out.println("adding 2222 at index : " + myList.size() / 2); myList.add(myList.size() / 2, 2222); printArray(null, myList);
System.out.println("adding 3333 at the end, index : " + myList.size()); myList.add(myList.size(), 3333); printArray(null, myList);
printTestTrailer();
printTestHeader(testCount++, "test invalid indices ");
System.out.println("testing invalid add"); myList.add(myList.size() + 1, 1111);
System.out.println("testing invalid get"); int badVal = myList.get(myList.size());
System.out.println("testing invalid remove"); badVal = myList.remove(myList.size() + 1);
printTestTrailer(); printTestHeader(testCount++, "test remove method"); System.out.println("List before remove: " + myList); System.out.println("Removing value at index 2 and then 8"); myList.remove(2); myList.remove(8); System.out.println("List after remove: " + myList); printTestTrailer();
}
}
Step by Step Solution
3.45 Rating (155 Votes )
There are 3 Steps involved in it
Step: 1
It seems like youre working on implementing an ArrayList class for Integer objects in Java I can help you with your code Lets go step by step First lets take a look at the ArrayListInteger class Heres ...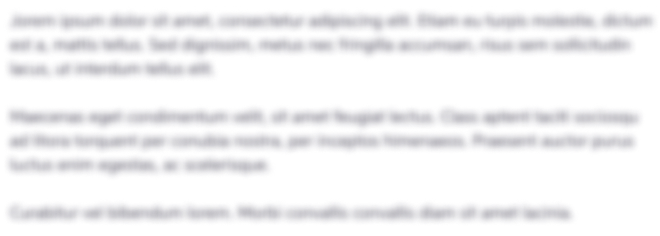
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started