Question
Anyone have a solution for my code? //Main.cpp #include TemperatureDatabase.h #include using namespace std; int main(int argc, char** argv) { if (argc < 3) {
Anyone have a solution for my code?
//Main.cpp
#include "TemperatureDatabase.h"
#include
using namespace std;
int main(int argc, char** argv) {
if (argc < 3) {
cout << "Usage: " << argv[0] << " data_file query_file" << endl;
return 1;
} else {
TemperatureDatabase database;
database.loadData(argv[1]);
//database.performQuery(argv[2]); // Will be done in Part 2
}
}
//LinkedList.cpp
#include
#include
#include "LinkedList.h"
#include "Node.h"
using namespace std;
LinkedList::LinkedList() {
head = nullptr;
tail = nullptr;
// Implement this function
}
LinkedList::~LinkedList() {
// Implement this function
clear();
}
LinkedList::LinkedList(const LinkedList& source) {
Node* previous = source.head;
this->head = nullptr;
if (previous != nullptr)
{
Node* node = new Node;
node->location = previous->location;
node->year = previous->year;
node->month = previous->month;
node->temperature = previous->temperature;
node->query = previous->query;
node->next = previous->next;
this->head = node;
Node* current = this->head;
previous = previous->next;
while (previous != nullptr)
{
node = new Node;
node->location = previous->location;
node->year = previous->year;
node->month = previous->month;
node->temperature = previous->temperature;
node->query = previous->query;
node->next = previous->next;
current->next = node;
current = current->next;
previous = previous->next;
}
}
}
LinkedList& LinkedList::operator=(const LinkedList& source) {
{
clear();
Node* previous = source.head;
if (previous != nullptr){
newNode = new Node;
newNode->location = oldNode->location;
newNode->year = oldNode->year;
newNode->month = oldNode->month;
newNode->temp = oldNode->temp;
newNode->query = oldNode->query;
newNode->next = oldNode->next;
this->head = newNode;
Node* curr = this->head;
oldNode = oldNode->next;
while (oldNode != NULL) {
newNode = new Node;
newNode->location = oldNode->location;
newNode->year = oldNode->year;
newNode->month = oldNode->month;
newNode->temp = oldNode->temp;
newNode->query = oldNode->query;
newNode->next = oldNode->next;
curr->next = newNode;
curr = curr->next;
oldNode = oldNode->next;
}
}
return *this;
}
void LinkedList::insert(string location, int year, int month, double temperature) {
Node* node = new Node(location, year, month, temperature);
Node* current = head;
Node* previous = nullptr;
if (this->head == nullptr && this->tail == nullptr)
{
head = node;
tail = node;
return;
}
else {
current->next = temp;
tail = temp;
temp->next = nullptr;
}
}
void LinkedList::clear() {
Node* current = this->head;
while (current != NULL) {
Node* deleteNode = current;
current = current->next;
delete deleteNode;
}
return;
}
Node* LinkedList::getHead() const {
return this-> head;
}
string LinkedList::print() const {
string outputString;
cout << outputString;
return outputString;
}
ostream& operator<<(ostream& os, const LinkedList& ll) {
/* Do not modify this function */
os << ll.print();
return os;
}
// LinkedList.h
#ifndef LINKEDLIST
#define LINKEDLIST
#include
#include
#include "Node.h"
class LinkedList {
private:
Node* head;
Node* tail;
public:
// Default constructor
LinkedList();
// Destructor
~LinkedList();
// Copy constructor
LinkedList(const LinkedList& other);
// Copy assignment
LinkedList& operator=(const LinkedList& other);
// Insert a record to the linked list
void insert(std::string location, int year, int month, double temperature);
// Clear the content of this linked list
void clear();
// The functions below are written already. Do not modify them.
std::string print() const;
Node* getHead() const;
};
std::ostream& operator<<(std::ostream& os, const LinkedList& ll);
#endif
//Node.cpp
#include
#include "Node.h"
using namespace std;
// Default constructor
Node::Node():next(nullptr) {} // remember to initialize next to nullptr
// Parameterized constructor
Node::Node(string id, int year, int month, double temperature): id(id), year(year), month(month), temperature(temperature), next(nullptr) {}
// remember to initialize next to nullptr
bool Node::operator<(const Node& b) {
return this->data < b.data;
}
//Node.h
#ifndef NODE
#define NODE
#include "TemperatureData.h"
struct Node {
TemperatureData data;
Node* next;
std::string id;
int month;
int year;
double temperature;
// Default constructor
Node(); // remember to initialize next to nullptr
Node(std::string id, int year, int month, double temperature); // remember to initialize next to nullptr
// This operator will allow you to just ask if a node is smaller than another
// rather than looking at all of the location, temperature and date information
bool operator<(const Node& b);
// The function below is written. Do not modify it
virtual ~Node() {}
};
#endif
TemperatureData.cpp
#include "TemperatureData.h"
#include
#include
#include
#include
#include
#include
#include
using namespace std;
using namespace std;
TemperatureData::TemperatureData() {} //initialize everything
TemperatureData::TemperatureData(std::string id, int year, int month, double temperature) {} //initialize everything
TemperatureData::~TemperatureData() {} // You should not need to implement this
bool TemperatureData::operator<(const TemperatureData& b) {
// Implement this
}
TemperatureData.h
#ifndef TEMPERATUREDATA
#define TEMPERATUREDATA
struct TemperatureData {
// Put data members here
TemperatureData();
TemperatureData(std::string id, int year, int month, double temperature);
virtual ~TemperatureData();
bool operator<(const TemperatureData& b);
};
#endif /* TEMPERATUREDATA */
TemperatureDatabase.cpp
#include "TemperatureDatabase.h"
#include
#include
#include
#include
#include
#include
#include
using namespace std;
// Default constructor/destructor. Modify them if you need to.
TemperatureDatabase::TemperatureDatabase() {}
TemperatureDatabase::~TemperatureDatabase() {}
void TemperatureDatabase::loadData(const string& filename) {
ifstream inFS("temps.dat");
if (!inFS.is_open()) {
cout << "Could not open file." << endl;
}
else {
while (!inFS.eof()) {
try {
int year;
int month;
int location;
double temp;
inFS >> location >> year >> month >> temp;
if (location > 99999 && location < 1000000) {
if (year > 1799 && year < 2018) {
if (month > 0 && month < 13) {
if (temp > -51 && temp < 51) {
records.insert(location, year, month, temp);
}
else
throw runtime_error("Temp out of range");
}
else
throw runtime_error("Month of of range");
}
else
throw runtime_error("Year out of range");
}
}
catch (const runtime_error& e) {
cout << e.what() << endl;
}
}
}
inFS.close();
return;
}
}
void TemperatureDatabase::performQuery(const string& filename) {
// Implement this function for part 2
// Leave it blank for part 1
}
TemperatureDatabase.h
#ifndef TEMP_DB
#define TEMP_DB
#include
#include "LinkedList.h"
class TemperatureDatabase {
public:
TemperatureDatabase();
~TemperatureDatabase();
// The two functions below are required
// Read the temperature records from the data file and populate the linked list
// Implement for Part 1
void loadData(const std::string& data_file);
// Read the queries from the query file and perform a series of queries
// Implement for Part 2
//void performQuery(const std::string& query_filename);
private:
// Linked list to store the temperature records. You need to properly populate
// this link list.
LinkedList records;
// You can add any private member variables/functions you feel useful in this class.
};
#endif // TEMP_DB
Step by Step Solution
There are 3 Steps involved in it
Step: 1
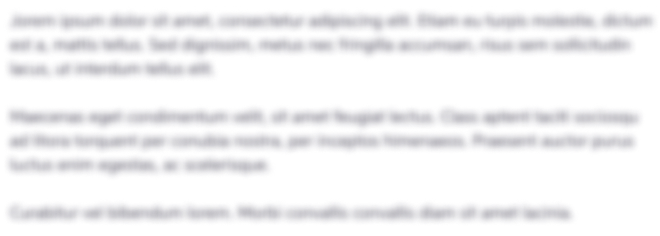
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started