Question
Application #2 ArrayStack class (provided as a skeleton) is to implement TextbookStackInterface The instance variable myStack is defined as an array and it should contain
Application #2
ArrayStack class (provided as a skeleton) is to implement TextbookStackInterface
The instance variable myStack is defined as an array and it should contain stacks entries. The array should be expanded dynamically as necessary.
The instance variable topIndex is defined to keep the index of the stacks top entry.
When the stack is empty, the first object pushed on the stack will be at the last slot of the array. Maintain the stacks bottom entry in this.myStack[this.myStack.length 1]. It means that the constructor must set this.topIndex to this.mystack.length also special care must be taken when array is resized. See the example below:
public interface TextbookStackInterface{ /** Adds a new entry to the top of this stack. @param newEntry An object to be added to the stack. */ public void push(T newEntry); /** Removes and returns this stack's top entry. @return The object at the top of the stack. throws appropriate exception if the stack is empty before the operation. */ public T pop(); /** Retrieves this stack's top entry. @return The object at the top of the stack. throws appropriate exception if the stack is empty. */ public T peek(); /** Detects whether this stack is empty. @return True if the stack is empty. */ public boolean isEmpty(); /** Removes all entries from this stack. */ public void clear(); /** ADDED TO TEST THE ARRAY IMPLEMENTATION ONLY */ public int getTopIndex(); public int getCapacity(); } // end StackInterface
public final class ArrayStack implements TextbookStackInterface { private T[] myStack; // array of myStack entries private int topIndex; // index of top entry private boolean initialized = false; private static final int DEFAULT_CAPACITY = 50; private static final int MAX_CAPACITY = 10000; public ArrayStack() { // TODO PROJECT #2 } // end default constructor public ArrayStack(int initialCapacity) { // TODO PROJECT #2 } // end constructor public void push(T newEntry) { // TODO PROJECT #2 } // end push public T peek() throws InsufficientNumberOfElementsOnStackException { // TODO PROJECT #2 return null; // THIS IS A STUB } // end peek public T pop() throws InsufficientNumberOfElementsOnStackException { // TODO PROJECT #2 return null; // THIS IS A STUB } // end pop public boolean isEmpty() { // TODO PROJECT #2 return false; //THIS IS A STUB } // end isEmpty public void clear() { // TODO PROJECT #2 } // end clear // Throws an exception if this object is not initialized. private void checkInitialization() throws SecurityException { // TODO PROJECT #2 } // end checkInitialization // Throws an exception if the client requests a capacity that is too large. private void checkCapacity(int capacity) throws IllegalStateException { // TODO PROJECT #2 } // end checkCapacity // Doubles the size of the array myStack if it is full // Precondition: checkInitialization has been called. private void ensureCapacity() { // TODO PROJECT #2 } // end ensureCapacity // These methods are only for testing of the array implementation public int getTopIndex() { return this.topIndex; } public int getCapacity() { return this.myStack.length; } public static void main(String[] args) { System.out.println("*** Creating a stack with default constructor ***"); TextbookStackInterface defaultStack = new ArrayStack<>(); System.out.println("---> The stack capacity is set by the constructor to: " + defaultStack.getCapacity()); System.out.println("---> The topIndex is: " + defaultStack.getTopIndex()); System.out.println(" ---> isEmpty() returns " + defaultStack.isEmpty()); int topLocation = defaultStack.getTopIndex(); int capacity = defaultStack.getCapacity(); if (topLocation == capacity) System.out.println("CORRECT - the top index is set to " + capacity); else System.out.println("INCORRECT - the top index is set to " + topLocation); System.out.println(" ---> Adding to stack to get: " + "Joe Jane Jill Jess Jim"); defaultStack.push("Jim"); defaultStack.push("Jess"); defaultStack.push("Jill"); defaultStack.push("Jane"); defaultStack.push("Joe"); System.out.println("---> Done adding 5 elements; the topIndex is: " + defaultStack.getTopIndex()); System.out.println("---> isEmpty() returns " + defaultStack.isEmpty()); System.out.println(" --> Testing peek and pop:"); while (!defaultStack.isEmpty()) { String top = defaultStack.peek(); System.out.println(top + " is at the top of the stack."); top = defaultStack.pop(); System.out.println(top + " is removed from the stack."); } // end while System.out.println("--> The stack should be empty: "); System.out.println("isEmpty() returns " + defaultStack.isEmpty()); System.out.println(" --> Adding to stack to get: " + "Jim Jess Joe"); defaultStack.push("Joe"); defaultStack.push("Jess"); defaultStack.push("Jim"); System.out.println("---> Done adding 3 elements; the topIndex is: " + defaultStack.getTopIndex()); System.out.println(" --> Testing clear:"); defaultStack.clear(); System.out.println("--> The stack should be empty: "); System.out.println("isEmpty() returns " + defaultStack.isEmpty()); try { System.out.println("defaultStack.peek() returns: "); System.out.println(defaultStack.peek()); } catch (InsufficientNumberOfElementsOnStackException inoeose) { System.out.println(" CORRECT - exception has been thrown: " + inoeose.getMessage()); } try { System.out.println("defaultStack.pop() returns: "); System.out.println(defaultStack.pop()); } catch (InsufficientNumberOfElementsOnStackException inoeose) { System.out.println(" CORRECT - exception has been thrown: " + inoeose.getMessage()); } System.out.println(" *** Creating a stack with the secondary constructor ***"); TextbookStackInterface smallStack = new ArrayStack<>(3); System.out.println("---> The stack capacity is set by the constructor to: " + smallStack.getCapacity()); System.out.println("---> The topIndex is: " + smallStack.getTopIndex()); System.out.println(" --> isEmpty() returns " + smallStack.isEmpty()); System.out.println(" --> Adding to stack to get: " + "Joe Jane Jill Jess Jim"); smallStack.push("Jim"); smallStack.push("Jess"); smallStack.push("Jill"); smallStack.push("Jane"); smallStack.push("Joe"); System.out.println("---> Done adding 5 elements; the topIndex is: " + smallStack.getTopIndex()); System.out.println("--> isEmpty() returns " + smallStack.isEmpty()); System.out.println(" -->Testing peek and pop:"); while (!smallStack.isEmpty()) { String top = smallStack.peek(); System.out.println(top + " is at the top of the stack."); top = smallStack.pop(); System.out.println(top + " is removed from the stack."); } // end while System.out.println("--> The stack should be empty: "); System.out.println("isEmpty() returns " + smallStack.isEmpty()); topLocation = smallStack.getTopIndex(); capacity = smallStack.getCapacity(); if (topLocation == capacity) System.out.println("CORRECT - the top index is set to " + capacity); else System.out.println("INCORRECT - the top index is set to " + topLocation); System.out.println(" --> Adding to stack to get: " + "Jim Jess Joe"); smallStack.push("Joe"); smallStack.push("Jess"); smallStack.push("Jim"); System.out.println("---> Done adding 3 elements; the topIndex is: " + smallStack.getTopIndex()); System.out.println(" --> Testing clear:"); smallStack.clear(); System.out.println("--> The stack should be empty: "); System.out.println("isEmpty() returns " + smallStack.isEmpty()); try { System.out.println("smallStack.peek() returns: "); System.out.println(smallStack.peek()); } catch (InsufficientNumberOfElementsOnStackException inoeose) { System.out.println(" CORRECT - exception has been thrown: " + inoeose.getMessage()); } try { System.out.println("smallStack.pop() returns: "); System.out.println(smallStack.pop()); } catch (InsufficientNumberOfElementsOnStackException inoeose) { System.out.println(" CORRECT - exception has been thrown: " + inoeose.getMessage()); } System.out.println("*** Done ***"); } // end main } // end ArrayStack
SAMPLE RUN:
*** Creating a stack with default constructor ***
---> The stack capacity is set by the constructor to: 50
---> The topIndex is: 50
---> isEmpty() returns true
CORRECT - the top index is set to 50
---> Adding to stack to get: Joe Jane Jill Jess Jim
---> Done adding 5 elements; the topIndex is: 45
---> isEmpty() returns false
--> Testing peek and pop:
Joe is at the top of the stack.
Joe is removed from the stack.
Jane is at the top of the stack.
Jane is removed from the stack.
Jill is at the top of the stack.
Jill is removed from the stack.
Jess is at the top of the stack.
Jess is removed from the stack.
Jim is at the top of the stack.
Jim is removed from the stack.
--> The stack should be empty:
isEmpty() returns true
--> Adding to stack to get: Jim Jess Joe
---> Done adding 3 elements; the topIndex is: 47
--> Testing clear:
--> The stack should be empty:
isEmpty() returns true
defaultStack.peek() returns:
CORRECT - exception has been thrown: peek operation failed
defaultStack.pop() returns:
CORRECT - exception has been thrown: pop operation failed
*** Creating a stack with the secondary constructor ***
---> The stack capacity is set by the constructor to: 3
---> The topIndex is: 3
--> isEmpty() returns true
--> Adding to stack to get: Joe Jane Jill Jess Jim
-----> The stack capacity has been doubled and it is now: 6; with topIndex = 3
---> Done adding 5 elements; the topIndex is: 1
--> isEmpty() returns false
-->Testing peek and pop:
Joe is at the top of the stack.
Joe is removed from the stack.
Jane is at the top of the stack.
Jane is removed from the stack.
Jill is at the top of the stack.
Jill is removed from the stack.
Jess is at the top of the stack.
Jess is removed from the stack.
Jim is at the top of the stack.
Jim is removed from the stack.
--> The stack should be empty:
isEmpty() returns true
CORRECT - the top index is set to 6
--> Adding to stack to get: Jim Jess Joe
---> Done adding 3 elements; the topIndex is: 3
--> Testing clear:
--> The stack should be empty:
isEmpty() returns true
smallStack.peek() returns:
CORRECT - exception has been thrown: peek operation failed
smallStack.pop() returns:
CORRECT - exception has been thrown: pop operation failed
*** Done ***
Step by Step Solution
There are 3 Steps involved in it
Step: 1
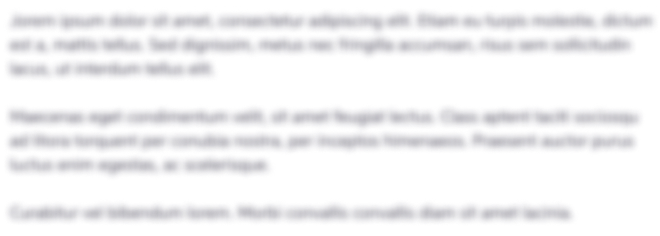
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started