app.py ----------------------------------------------------- import flask from flask import request, g, jsonify from passlib.hash import sha256_crypt import sqlite3 from flask_basicauth import BasicAuth app = flask.Flask(__name__) app.config[debug] =
app.py
----------------------------------------------------- import flask from flask import request, g, jsonify from passlib.hash import sha256_crypt import sqlite3 from flask_basicauth import BasicAuth
app = flask.Flask(__name__) app.config["debug"] = True DATABASE = "database.db"
class bAuth(BasicAuth): def check_credentials(self, username, password): password = sha256_crypt.encrypt("password") query = "SELECT * FROM users WHERE username = ? AND password = ?" query_filter = (username, sha256_crypt.verify("password", password_hash)) conn = sqlite3.connect(DATABASE) cur = conn.cursor()
result = cur.execute(query, query_filter).fetchall() return str(result[0])
auth = bAuth(app)
@app.cli.command() def init_db(): with app.app_context(): db = get_db() with app.open_resource('ini_DB.sql', mode='r') as f: db.cursor().exexcutescript(f.read()) db.commit()
def make_dicts(cursor, row): return dict((cursor.description[idx][0], value) for idx, value in enumerate(row))
def get_db(): db = getattr(g, '_database', None) if db is None: db = g._database = sqlite3.connect(DATABASE) db.row_factory = make_dicts return db
@app.teardown_appcontext def close_connection(exception): db = getattr(g, '_database', None) if db is not None: db.close()
def query_db(query, args=(), one=False): try: db = get_db().execute(query, args) rv = db.fetchall() db.close() return (rv[0] if rv else None) if one else rv except sqlite3.Error as e: print('EXCEPTION AT query_db:%s' %(e)) if one: return None else: return []
def insert_db(query, args=()): try: db = get_db().cursor() db.execute(query, args) get_db().commit() except sqlite3.Error as e: print('EXCEPTION AT insert_db:%s' %(e))
def user_exists(username): conn = sqlite3.connect(DATABASE) conn.row_factory = dict_factory cur = conn.cursor() query = "SELECT id FROM users WHERE username = ?" result = cur.execute(query, [username]).fetchone() cur.close() if result: return 'YES' else: return 'NO' # User Register @app.route('/register', methods=['GET','POST','PUT']) def register(): if request.method == 'GET': return 'HTTP 499, You can not make a get request to this route' elif request.method == 'POST': data = request.json username = data['username'] if (user_exists(username) == 'YES'): return 'username already used' else: conn = sqlite3.connect(DATABASE) conn.row_factory = dict_factory cur = conn.cursor() cur.execute('INSERT INTO users(username) VALUES (?)', [username]) conn.comit() cur.close() conn.close() return 'You are now registered' elif request.method == 'PUT': data = request.json username = data['username'] password = sha256_crypt.encrypt(str(data['password'])) if(user_exists(username) == 'YES'): conn.sqlite3.connect(DATABASE) conn.row_factory = dict_factory cur = conn.cursor() cur.execute('UPDATE users SET password = ? WHERE username = ?', (username, password)) conn.commit() cur.close() conn.close() return jsonify(data) else: return 'HTTP 404 Not found if username does not exist. HTTP 409 Conflict if username does not match the current authenticated user' app.run()
and here is ini_DB.sql
------------------------------------
DROP TABLE IF EXISTS users; DROP TABLE IF EXISTS articles;
CREATE TABLE users( id INTEGER PRIMARY KEY, username TEXT, email TEXT, password TEXT, timestamp TEXT );
CREATE TABLE articles( id INTEGER PRIMARY KEY, title TEXT, body TEXT, author TEXT, timestamp TEXT );
INSERT INTO users ( name, username, email, password, timestamp ) VALUES ( 'abc', 'abc3', 'abc@example.com', 'password', DATETIME('now','localtime') );
INSERT INTO Articles( title, body, author, timestamp ) VALUES ( 'title 1', 'this is the body', (select username from users where username = 'tho96'), DATETIME('now','localtime') ); commit;
CAN YOU PLEASE HELP ME FIGURE OUT WHAT'S WRONG WITH THIS?
I COULDN'T EXECUTE THE CODE IN FLASK
COMMAND LINE: python app.py
curl localhost:5000/register
Step by Step Solution
There are 3 Steps involved in it
Step: 1
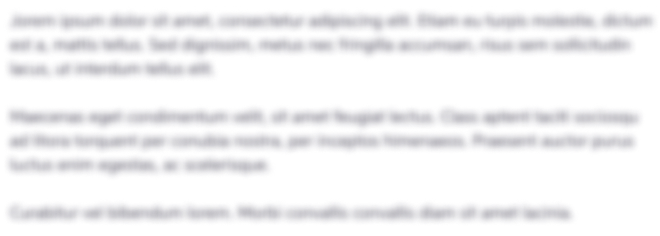
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started