Question
Are they adjacent? Can you send me the original code which i can copy and paste please. thank you so much In this assignment you
Are they adjacent?
Can you send me the original code which i can copy and paste please. thank you so much
In this assignment you will write two functions which will both determine if two vertices are adjacent. One will work on matrix representations, one will work on list representations. They will both take the representation of the graph as the first argument and the two vertices to check in the second and third arguments. In this assignment, we will assume all graphs are bidirectional and unweighted. Nodes will be labeled with integers for ease of coding
Example graph:
Its list representation:
graph_list = [
[1],
[0, 2, 3, 4],
[1, 3],
[1, 2, 4],
[1, 3],
]
Its matrix representation:
graph_matrix = [
[0, 1, 0, 0, 0],
[1, 0, 1, 1, 1],
[0, 1, 0, 1, 0],
[0, 1, 1, 0, 1],
[0, 1, 0, 1, 0],
]
Expected behavior:
adjacent_list(graph_list, 0, 1) == True adjacent_list(graph_list, 0, 2) == False
adjacent_list(graph_list, 1, 4) == True
adjacent_list(graph_list, 4, 2) == False
adjacent_matrix(graph_matrix, 0, 1) == True adjacent_matrix(graph_matrix, 0, 2) == False
adjacent_matrix(graph_matrix, 1, 4) == True
adjacent_matrix(graph_matrix, 4, 2) == False
Bonus (2 points):
Write a function called adjacent(), which takes either a list or a graph as its first argument and node labels as its second and third arguments and then determines which representation we're looking at before determining if two nodes are adjacent.
Expected behavior:
adjacent(graph_list, 0, 1) == True adjacent(graph_list, 0, 2) == False
adjacent(graph_list, 1, 4) == True
adjacent(graph_list, 4, 2) == False
adjacent(graph_matrix, 0, 1) == True adjacent(graph_matrix, 0, 2) == False
adjacent(graph_matrix, 1, 4) == True
adjacent(graph_matrix, 4, 2) == False
use this same code please.
def adjacent_matrix(graph, node_1, node_2): # Your code here
### BONUS def adjacent(graph, node_1, node_2): # Your bonus code here. Remove the print statement print ('Not Implemented')
4 0 2 3Step by Step Solution
There are 3 Steps involved in it
Step: 1
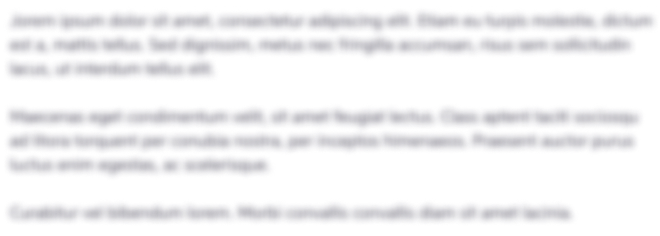
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started