Answered step by step
Verified Expert Solution
Question
1 Approved Answer
ArrayList.h: 8 : #ifndef _ ARRAY _ LIST 9 : #define _ ARRAY _ LIST 1 1 : #include ListInterface.h 1 2 : #include
ArrayList.h: : #ifndef ARRAYLIST : #define ARRAYLIST : #include "ListInterface.h : #include "PrecondViolatedExcep.h : template : class ArrayList : public ListInterface : : private: : static const int DEFAULTCAPACITY ; Small capacity to test for a full list : ItemType itemsDEFAULTCAPACITY; Array of list items : int itemCount; Current count of list items : int maxItems; Maximum capacity of the list : : public: : ArrayList; : Copy constructor and destructor are supplied by compiler : : bool isEmpty const; : int getLength const; : bool insertint newPosition, const ItemType& newEntry; : bool removeint position; : void clear; : : @throw PrecondViolatedExcep if position or : position getLength : ItemType getEntryint position const throwPrecondViolatedExcep; : @throw PrecondViolatedExcep if position or : position getLength : void setEntryint position, const ItemType& newEntry : throwPrecondViolatedExcep; : ; end ArrayList : #include "ArrayList.cpp : #endif ArrayList.cpp: : #include "ArrayList.h Header file : template : ArrayList::ArrayList : itemCount maxItemsDEFAULTCAPACITY : : end default constructor : template : bool ArrayList::isEmpty const : : return itemCount ; : end isEmpty : template : int ArrayList::getLength const : : return itemCount; : end getLength : template : bool ArrayList::insertint newPosition, const ItemType& newEntry : : bool ableToInsert newPosition && newPosition itemCount && : itemCount maxItems; : if ableToInsert : : Make room for new entry by shifting all entries at : positions newPosition toward the end of the array : no shift if newPosition itemCount : for int pos itemCount; pos newPosition; pos : itemspos itemspos ; : : Insert new entry : itemsnewPosition newEntry; : itemCount; Increase count of entries : end if : : return ableToInsert; : end insert : template : bool ArrayList::removeint position : : bool ableToRemove position && position itemCount; : if ableToRemove : : Remove entry by shifting all entries after the one at : position toward the beginning of the array : no shift if position itemCount : for int fromIndex position, toIndex fromIndex ; fromIndex itemCount; : fromIndex toIndex : itemstoIndex itemsfromIndex; : : itemCount; Decrease count of entries : end if : : return ableToRemove; : end remove : template : void ArrayList::clear : : itemCount ; : end clear : template : ItemType ArrayList::getEntryint position const throwPrecondViolatedExcep : : Enforce precondition : bool ableToGet position && position itemCount; : if ableToGet : return itemsposition ; : else : : string message "getEntry called with an empty list or ; : message message "invalid position."; : throwPrecondViolatedExcepmessage; : end if : end getEntry : template : void ArrayList::setEntryint position, const ItemType& newEntry throwPrecondViolatedExcep : : Enforce precondition : bool ableToSet position && position itemCount; : if ableToSet : itemsposition newEntry; : else : : string message "setEntry called with an empty list or ; : message message "invalid position."; : throwPrecondViolatedExcepmessage; : end if :
ArrayList.h:
: #ifndef ARRAYLIST
: #define ARRAYLIST
: #include "ListInterface.h
: #include "PrecondViolatedExcep.h
: template
: class ArrayList : public ListInterface
:
: private:
: static const int DEFAULTCAPACITY ; Small capacity to test for a full list
: ItemType itemsDEFAULTCAPACITY; Array of list items
: int itemCount; Current count of list items
: int maxItems; Maximum capacity of the list
:
: public:
: ArrayList;
: Copy constructor and destructor are supplied by compiler
:
: bool isEmpty const;
: int getLength const;
: bool insertint newPosition, const ItemType& newEntry;
: bool removeint position;
: void clear;
:
: @throw PrecondViolatedExcep if position or
: position getLength
: ItemType getEntryint position const throwPrecondViolatedExcep;
: @throw PrecondViolatedExcep if position or
: position getLength
: void setEntryint position, const ItemType& newEntry
: throwPrecondViolatedExcep;
: ; end ArrayList
: #include "ArrayList.cpp
: #endif
ArrayList.cpp:
: #include "ArrayList.h Header file
: template
: ArrayList::ArrayList : itemCount maxItemsDEFAULTCAPACITY
:
: end default constructor
: template
: bool ArrayList::isEmpty const
:
: return itemCount ;
: end isEmpty
: template
: int ArrayList::getLength const
:
: return itemCount;
: end getLength
: template
: bool ArrayList::insertint newPosition, const ItemType& newEntry
:
: bool ableToInsert newPosition && newPosition itemCount &&
: itemCount maxItems;
: if ableToInsert
:
: Make room for new entry by shifting all entries at
: positions newPosition toward the end of the array
: no shift if newPosition itemCount
: for int pos itemCount; pos newPosition; pos
: itemspos itemspos ;
:
: Insert new entry
: itemsnewPosition newEntry;
: itemCount; Increase count of entries
: end if
:
: return ableToInsert;
: end insert
: template
: bool ArrayList::removeint position
:
: bool ableToRemove position && position itemCount;
: if ableToRemove
:
: Remove entry by shifting all entries after the one at
: position toward the beginning of the array
: no shift if position itemCount
: for int fromIndex position, toIndex fromIndex ; fromIndex itemCount;
: fromIndex toIndex
: itemstoIndex itemsfromIndex;
:
: itemCount; Decrease count of entries
: end if
:
: return ableToRemove;
: end remove
: template
: void ArrayList::clear
:
: itemCount ;
: end clear
: template
: ItemType ArrayList::getEntryint position const throwPrecondViolatedExcep
:
: Enforce precondition
: bool ableToGet position && position itemCount;
: if ableToGet
: return itemsposition ;
: else
:
: string message "getEntry called with an empty list or ;
: message message "invalid position.";
: throwPrecondViolatedExcepmessage;
: end if
: end getEntry
: template
: void ArrayList::setEntryint position, const ItemType& newEntry throwPrecondViolatedExcep
:
: Enforce precondition
: bool ableToSet position && position itemCount;
: if ableToSet
: itemsposition newEntry;
: else
:
: string message "setEntry called with an empty list or ;
: message message "invalid position.";
: throwPrecondViolatedExcepmessage;
: end if
:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
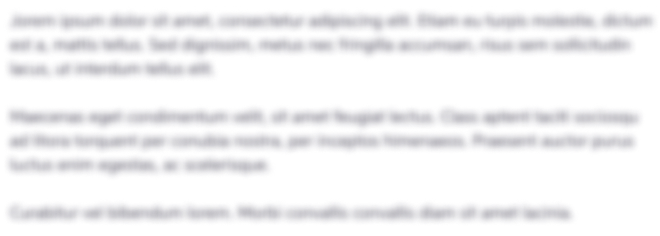
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started