Question
ArrayLists and Collections Only use Array List to answer please, take your time. it is due mid way friday Summary Create a data type class
ArrayLists and Collections
Only use Array List to answer please, take your time. it is due mid way friday
Summary
Create a data type class for a deck of cards. I have provided you with a data type Card for the cards themselves, and a program Game that simply deals out hands and then returns them to the deck.
The Cards
I have provided you with a class Card that represents a single playing card.
Do not modify this class. It performs exactly the way I want it to.
It contains several constants and two Lists that will be helpful to you:
- SPADES, HEARTS, DIAMONDS, CLUBS: ints representing the four suits a card might be from;
- ACE, JACK, QUEEN, KING: ints representing the four non-numeric ranks a card might have;
- SUITS: a List of Integers representing the four suits (SPADES, HEARTS, DIAMONDS, CLUBS); and
- RANKS: a List of Integers representing the thirteen ranks (ACE, 2, 3, 4, ..., 10, JACK, QUEEN, KING).
The Card constructor is expecting to be given two int values representing the rank and the suit of the card to be constructed. For example,
Card aceOfClubs = new Card(Card.ACE, Card.CLUBS);
creates the ace of clubs. And, of course, you can use variables in the constructor. So if r = 3 and s = Card.DIAMONDS then
Card card = new Card(r, s);
creates the 3 of diamonds.
The Deck
Your task in this assignment is to create the Deck class. It represents a deck of cards using a List. The class implements the following methods and constructors:
- Deck() create a standard deck of cards
- int cardsLeft() return the number of cards left in the deck
- Card takeTopCard() return the top card of the deck (which is removed from the deck)
- void shuffle() shuffle the deck
- List deal(int numCards) deal the given number of cards from the deck param numCards the number of cards to be dealt return a sorted list of cards (which are removed from the deck)
- void returnCards(List cards) add cards back into the deck (at the bottom) param cards a list of the cards to be returned to the deck
- void shuffleIn(List cards) add cards back into the deck and shuffle the resulting deck param cards a list of the cards to be returned to the deck
- void shuffleIn(Deck otherDeck) add another deck to this one and shuffle the resulting deck param otherDeck the deck to be added to this one
The longest of those is the constructor -- which must figure out what all 52 cards are and create them. The majority of the others can be done in a single line.
Remember to call your own methods where appropriate rather than copying-and-pasting code.
Remember also to start with stubs for all the methods above. Once you have all the stubs written, compile and run the game. It won't do much, but will start to fill out as you fill in the proper method definitions.
The Game
Card.java starts here
package a07; import java.util.List; import java.util.Arrays; /** * A class representing a single playing card * * @author */ public class Card implements Comparable { public final static int SPADES = 0, // Codes for the 4 suits. HEARTS = 1, DIAMONDS = 2, CLUBS = 3; public final static int ACE = 1, // Codes for the non-numeric cards. JACK = 11, // Cards 2 through 10 have their QUEEN = 12, // numerical values for their codes. KING = 13; /** a list of all the suits */ public final static List SUITS = Arrays.asList(SPADES, HEARTS, DIAMONDS, CLUBS); /** a list of all the ranks */ public final static List RANKS = Arrays.asList(ACE, 2, 3, 4, 5, 6, 7, 8, 9, 10, JACK, QUEEN, KING); private final int suit; // The suit of this card, one of the constants // SPADES, HEARTS, DIAMONDS, CLUBS. private final int rank; // The rank of this card, from 1 to 13. /** * Create a single playing card. * * @param theRank the rank (1 to 13) of this playing card * @param theSuit the suit (0 to 3) of this playing card */ public Card(int theRank, int theSuit) { // Construct a card with the specified rank and suit. // Rank must be between 1 and 13. Suit must be between // 0 and 3. If the parameters are outside these ranges, // the constructed card object will be invalid. mustBeRank(theRank); mustBeSuit(theSuit); rank = theRank; suit = theSuit; } /** * The suit of this card. * * @return the suit (0 to 3) of this card */ public int getSuit() { // Return the int that codes for this card's suit. return suit; } /** * The rank of this card. * * @return the rank (1 TO 13) of this card */ public int getRank() { // Return the int that codes for this card's rank. return rank; } /** * The name of this card's suit. * * @return the English name of the suit of this card */ public String getSuitAsString() { switch ( suit ) { case SPADES: return "Spades"; case HEARTS: return "Hearts"; case DIAMONDS: return "Diamonds"; case CLUBS: return "Clubs"; // THE DEFAULT CASE SHOULD NEVER HAPPEN! default: throw new IllegalStateException(); } } /** * The first letter of this card's suit name. * * @return the first letter of the English name of this card's suit */ public String getSuitAbbreviation() { return getSuitAsString().substring(0, 1); } /** * Return the name of this card's rank. * * @return a String representing the rank of this card */ public String getRankAsString() { switch ( rank ) { case 1: return "Ace"; case 2: return "2"; case 3: return "3"; case 4: return "4"; case 5: return "5"; case 6: return "6"; case 7: return "7"; case 8: return "8"; case 9: return "9"; case 10: return "10"; case 11: return "Jack"; case 12: return "Queen"; case 13: return "King"; // THE DEFAULT CASE SHOULD NEVER HAPPEN! default: throw new IllegalStateException(); } } /** * Return the rank of this card abbreviated to one character (or two * characters, for the 10). * * @return the one or two character abbreviation of this card's rank */ public String getRankAbbreviation() { String rankString = getRankAsString(); // only the named ranks have length greater than two if (rankString.length() > 2) { // in which case we return their first lettter (A, K, Q, J) return rankString.substring(0, 1); } return rankString; } /** * Return the short version of this Card's identity -- its rank and suit * abbreviated to one letter each (or two letters, for the 10. * * @return a String representing this card (abbreviated) */ public String toString() { return getRankAbbreviation() + getSuitAbbreviation(); } /** * compare this card to another card * * @param otherCard the card to compare to * @return a negative/zero/positive number depending on whether this card * is before/equal/after the other */ public int compareTo(Card otherCard) { int myValue = getValue(); int itsValue = otherCard.getValue(); return myValue - itsValue; } /** * Return a number representing this card's position in a sorted deck. * (Used, of course, in the compareTo method.) * * @return a single number (1 to 52) representing this card */ private int getValue() { // There are two ways to sort cards // -- by suit: all cards in the same suit are put together // and then sorted within the suit from low to high // -- by rank: all cards of the same rank are put together // and by an arbitrary suit order within rank // We should have a static method or class variables to tell us // which way to sort the cards. For now, we'll just do suit order. // Suit ordered return suit * RANKS.size() + rank; // Rank-ordered // return rank * SUITS.size() + suit; } /** * Throw an exception if the argument isn't a rank. * * @param value the value to check */ private static void mustBeRank(int value) { if (!Card.RANKS.contains(value)) { throw new IllegalArgumentException("Not a rank: " + value); } } /** * Throw an exception if the argument isn't a suit. * * @param value the value to check */ private static void mustBeSuit(int value) { if (!Card.SUITS.contains(value)) { throw new IllegalArgumentException("Not a suit: " + value); } } } // end class Card
Game.java starts below
package a07; import java.util.*; /** * A program to run the Deck class thru its paces. * * @author */ public class Game { public static void main(String[] args) { Scanner kbd = new Scanner(System.in); Deck d; int numPlayers, numCards, cardsNeeded; // introduce this program System.out.print(" " + "Simple Card Game " + "================ " + "This game just deals cards. "); // Announce the first game System.out.print("First game " + "---------- "); // get a new deck of cards d = new Deck(); // activate next line after you've got the constructor working properly // d.shuffle(); // get game input System.out.print("How many players are in the game? "); numPlayers = kbd.nextInt(); kbd.nextLine(); System.out.print("How many cards does each player get? "); numCards = kbd.nextInt(); kbd.nextLine(); cardsNeeded = numPlayers * numCards; System.out.println(); // play the game -- if there are enuf cards! if (cardsNeeded >= d.cardsLeft()) { System.out.println("Not enuf cards to play the first game!"); } else { for (int p = 1; p d.cardsLeft()) { System.out.println("Not enuf cards to play the second game!"); } else { for (int p = 1; pSAMPLE OUTPUT
Note; only a file Deck.java is to be created without any changes in Game.java and Card.java
The Game I have provided the "game" for you. It doesn't do much -- essentially it deals out hands from a single deck, then deals out hands from a double deck. (The hands from the double deck are returned to the bottom of the deck after being shown to the user, so you can have as many players as you want, with up to 104 cards in each hand.) You should stick with the first game until you have the Deck class pretty much finished. The hands in the first game will be sequential cards -- for example, six hands of five cards might come out as Player 1 gets (AS, 2S, 3S, 4s, 5S] Player 2 gets [6S, 75, 88, 99, 10S] Player 3 gets [JS, QS, KS, AH, 2H] Player 4 gets [3H, 4H, 5H, 6H, TH] Player 5 gets [8H, 9H, 10H, JH, QH] Player 6 gets [KH, AD, 2D, 3D, 4D] It doesn't matter if they come out different than that -- so long as you can tell that all 52 cards are there, and none are duplicated. After you're sure of that, you can activate the line in Game that shuffles the cards. That should give you hands more like: Player 1 gets [4S, AD, 3D, 20, 75] Player 2 gets [9H, 8D, 4C, 6D, QC] Player 3 gets [Qs, 3H, 4, 35, 10H] Player 4 gets [95, 2H, 6S, JS, AS] Player 5 gets [7C, KH, 7D, 8H, 4D] Player 6 gets [3C, 89, 29, 7H, 6H] And after you have the shuffling working, you must revise the Game Deck so that the hands are sorted before they are printed out. Your hands should now look like: Player 1 gets (AS, 3S, AH, 10H, QH, 2D, 4D, QD, 3C, 6C] Player 2 gets [45, 5S, 105, 4H, 3D, 8D, AC, 20, 4C, KC] Player 3 gets [25, 65, 85, 9s, KS, 5H, 9H, 10D, JD, 10C] Player 4 gets [7S, JS, Qs, 2H, 6H, 5D, KD, 7C, JC, QC] Player 5 gets [3H, 7H, 8H, JH, 6D, 7D, 9D, 5C, 8C, 9C] (Notice that the cards are sorted by suit (spades, hearts, diamonds, clubs), and from lowest to highest within each suit -- that is the way cards just "naturally sort". Remember, there is a one-line way to sort your list of cards!) The game with the double deck is used to test the shuffleIn(Deck) method (obviously), but also the returnCards method. If you use 10 players with 13 cards each in their hands, the last two players should get exactly the same hands as the first two. For example: Player 1 gets [75, 2C, JS, QD, 100, 9s, 3D, 6D, KC, AS, 2s, QC, 8D] Player 2 gets [6H, TH, JH, 5D, 5H, JS, 5S, QD, 20, 8H, AC, 41, 3C] Player 3 gets [75, 90, 2D, 2D, 10D, 105, 5C, 85, 6C, AD, KD, 7D, 6H] Player 4 gets [2H, QH, 9H, KS, 10H, JH, 100, KS, 8D, KH, 95, 3C, 5C] Player 5 gets [6S, AD, JC, 8C, 6D, 7H, 5D, 4D, 40, 40, 9H, 2H, KH] Player 6 gets [7C, AC, 3H, 35, QC, 89, 4H, JD, JD, JC, 9C, 3D, QS] Player 7 gets [10s, 45, 7C, AS, 3H, AH, 9D, KD, 8H, 39, 40, 5H, 7D] Player 8 gets [KC, 10H, QH, 6C, AH, 2s, 8C, 45, os, 65, 9D, 5S, 10D] Player 9 gets [75, 2C, JS, QD, 100, 9s, 3D, 6D, KC, AS, 2S, QC, 8D] Player 10 gets [6H, 7H, JH, 5D, 5H, JS, 5S, QD, 2C, 8H, AC, 41, 3C] First Sample -- no shuffle, no sort Simple Card Game This game just deals cards. First game How many players are in the game? 4 How many cards does each player get? 8 Player 1 gets [AS, AH, AD, AC, 25, 2H, 2D, 2C] Player 2 gets [35, 3H, 3D, 3C, 45, 41, 4D, 4C] Player 3 gets [5S, 51, 5D, 5C, 65, 6H, 6D, 6C] Player 4 gets [75, 7H, 7D, 70, 85, 8H, 8D, 8C] Top card from deck: 95 Second game How many players are in the game? 102 How many cards does each player get? 132 Player 1 gets [5H, 3D, 10D, KD, 25, QC, 2C, AC, 75, 3D, 10H, JD, AS] Player 2 gets [KD, 8H, KS, JH, AS, 7D, 9s, 2D, KC, 2H, 2C, 105, 5C] Player 3 gets [6D, 4H, QH, 2H, 8H, 6H, 89, 30, 3C, 100, 7H, 5H, 3S] Player 4 gets [45, 10D, 7D, 41, 9D, KC, 5s, 9C, JH, 9H, 40, 9C, 8C] Player 5 gets [6S, 9H, 6H, 6C, JS, 6S, 7H, QD, 8D, 7S, QH, JD, AD] Player 6 gets [35, 2D, AC, KS, 100, 5C, AH, 8D, 5D, 4C, 6C, 4D, QS] Player 7 gets [9D, Qs, 5s, 5D, 99, 45, 8S, JC, JS, 70, QC, 8C, 10H] Player 8 gets [AD, 70, 25, 105, QD, 3H, AH, KH, JC, 6D, KH, 3H, 4D] Player 9 gets [5H, 3D, 10D, KD, 2S, QC, 2C, AC, 75, 3D, 10H, JD, AS] Player 10 gets [KD, 8H, KS, JH, AS, 7D, 9S, 2D, KC, 2H, 20, 105, 5C] Second Sample -- shuffle, no sort Simple Card Game This game just deals cards. First game How many players are in the game? 42 How many cards does each player get? 8 Player 1 gets [2D, 8C, 20, 50, 6H, KS, 105, 10C] Player 2 gets [4C, KC, 70, 8H, 9s, 5D, JH, 10H] Player 3 gets [2H, JC, 8D, 5S, JD, 9D, KD, 4H] Player 4 gets [AS, 7D, 3D, QH, 5H, 85, 6S, QC] Top card from deck: 4D Second game How many players are in the game? 10 How many cards does each player get? 132 Player 1 gets [2H, 9C, 6C, 51, 8D, 100, 35, 6D, JH, 7H, 5, 4D, KH] Player 2 gets (JD, 75, 2D, 8C, 7H, 5S, KD, 8H, Qs, 9C, 3S, AS, 3C] Player 3 gets [KH, JC, 7D, 4H, 10H, 5S, KC, 89, 100, 99, KD, 5D, AS] Player 4 gets [JH, 65, 5C, 89, 4S, QD, 7C, JS, 105, AH, 4D, QH, KS] Player 5 gets [8D, 9D, AD, 5C, 4C, JD, 95, AC, 4C, 3H, 7C, 2S, JC] Player 6 gets [25, 7D, 9D, 3C, 45, QC, QC, 10H, 4H, 75, 10D, 20, 2C] Player 7 gets (JS, 2H, 5D, 9H, 105, 3H, QS, 8C, QH, 3D, 6C, 2D, AC] Player 8 gets [6H, 8H, KS, KC, QD, 10D, 6S, 3D, AH, AD, 6D, 9H, 6H] Player 9 gets [2H, 90, 60, 5H, 8D, 100, 35, 6D, JH, 7H, 5H, 4D, KH] Player 10 gets [JD, 75, 2D, 8C, 7H, 5S, KD, 8H, Qs, 90, 3S, AS, 3C] Third Sample -- shuffle, sort (example 1) Simple Card Game This game just deals cards. First game How many players are in the game? 44 How many cards does each player get? se Player 1 gets [6S, JH, 4D, 40, 60, 70, 90, QC] Player 2 gets [55, 75, 9S, AH, QH, AD, JD, KC] Player 3 gets [85, 9H, KH, 8D, 9D, AC, 30, 5C] Player 4 gets [45, 105, JS, KS, 2D, 7D, 2C, 8C] Top card from deck: 8H Second game How many players are in the game? 102 How many cards does each player get? 13 Player 1 gets (AS, 45, 85, 95, 105, 105, 7H, 6D, 6D, 10D, KD, AC, 2C] Player 2 gets [39, JS, KS, 2H, 6H, 7H, 9H, QH, KH, KH, AD, 5D, QC] Player 3 gets [35, 5S, 75, 9H, JH, AD, 2D, 3D, JD, OD, 20, 3C, 70] Player 4 gets [2s, os, KS, 3H, 3H, 8H, QH, 7D, 8D, 8D, 9D, QD, AC] Player 5 gets [55, 85, Q5, 6H, 10H, 4D, 10D, JD, 40, 80, 90, JC, KC] Player 6 gets [6S, JS, AH, AH, 5H, 10H, 3D, 5D, 5C, 60, 60, 90, 10C] Player 7 gets [2S, 75, 4H, 8H, JH, KD, 30, 40, 70, 80, JC, QC, KC] Player 8 gets [AS, 45, 65, 9s, 2H, 41, 5H, 2D, 4D, 7D, 9D, 5C, 10C] Player 9 gets [AS, 48, 89, 99, 105, 105, 7H, 6D, 6D, 10D, KD, AC, 2C] Player 10 gets [3S, JS, KS, 2H, 6H, 7H, 9H, QH, KH, KH, AD, 5D, QC] Fourth Sample -- shuffle, sort (example 2) Simple Card Game ================ This game just deals cards. First game How many players are in the game? 42 How many cards does each player get? Player 1 gets [6H, 9H, 107, 7D, 9D, 6C, 10C, KC] Player 2 gets [35, 105, gs, JH, AD, 2D, 10D, AC] Player 3 gets [AS, 55, AH, 7H, 5D, 6D, 30, 5C] Player 4 gets [45, 75, 5H, 4D, 8D, 40, 80, 9C] Top card from deck: 4H Second game How many players are in the game? 102 How many cards does each player get? 132 Player 1 gets [2S, 4S, 89, 7H, 10H, KH, 2D, 4D, 5D, 6D, AC, AC, QC] Player 2 gets [KS, AH, 3H, 5H, AD, 3D, 4D, 7D, 9D, 6C, 8C, JC, QC] Player 3 gets (105, KS, 3H, JH, QH, 2D, 10D, JD, JD, QD, 3C, 6C, JC] Player 4 gets [AS, 35, 5s, 5s, 10s, 47, 6, 8H, 3D, 6D, QD, 2C, 10C] Player 5 gets [AS, 3S, 6S, 9S, AH, 2H, JH, KD, 20, 40, 50, 70, 7C] Player 6 gets [2s, 45, 9s, JS, 4H, 8H, 9H, KH, 5D, 9D, 10D, 8C, KC] Player 7 gets [8S, JS, Qs, 2H, 5H, 6H, 10H, AD, 8D, KD, 30, 40, 9C] Player 8 gets [6S, 75, 75, qs, 7H, 9H, QH, 7D, 8D, 5C, 9C, 10C, KC] Player 9 gets [2S, 48, 89, 7H, 10H, KH, 2D, 4D, 5D, 6D, AC, AC, QC] Player 10 gets [KS, AH, 3H, 5H, AD, 3D, 4D, 7D, 9D, 6C, 80, JC, QC] The Game I have provided the "game" for you. It doesn't do much -- essentially it deals out hands from a single deck, then deals out hands from a double deck. (The hands from the double deck are returned to the bottom of the deck after being shown to the user, so you can have as many players as you want, with up to 104 cards in each hand.) You should stick with the first game until you have the Deck class pretty much finished. The hands in the first game will be sequential cards -- for example, six hands of five cards might come out as Player 1 gets (AS, 2S, 3S, 4s, 5S] Player 2 gets [6S, 75, 88, 99, 10S] Player 3 gets [JS, QS, KS, AH, 2H] Player 4 gets [3H, 4H, 5H, 6H, TH] Player 5 gets [8H, 9H, 10H, JH, QH] Player 6 gets [KH, AD, 2D, 3D, 4D] It doesn't matter if they come out different than that -- so long as you can tell that all 52 cards are there, and none are duplicated. After you're sure of that, you can activate the line in Game that shuffles the cards. That should give you hands more like: Player 1 gets [4S, AD, 3D, 20, 75] Player 2 gets [9H, 8D, 4C, 6D, QC] Player 3 gets [Qs, 3H, 4, 35, 10H] Player 4 gets [95, 2H, 6S, JS, AS] Player 5 gets [7C, KH, 7D, 8H, 4D] Player 6 gets [3C, 89, 29, 7H, 6H] And after you have the shuffling working, you must revise the Game Deck so that the hands are sorted before they are printed out. Your hands should now look like: Player 1 gets (AS, 3S, AH, 10H, QH, 2D, 4D, QD, 3C, 6C] Player 2 gets [45, 5S, 105, 4H, 3D, 8D, AC, 20, 4C, KC] Player 3 gets [25, 65, 85, 9s, KS, 5H, 9H, 10D, JD, 10C] Player 4 gets [7S, JS, Qs, 2H, 6H, 5D, KD, 7C, JC, QC] Player 5 gets [3H, 7H, 8H, JH, 6D, 7D, 9D, 5C, 8C, 9C] (Notice that the cards are sorted by suit (spades, hearts, diamonds, clubs), and from lowest to highest within each suit -- that is the way cards just "naturally sort". Remember, there is a one-line way to sort your list of cards!) The game with the double deck is used to test the shuffleIn(Deck) method (obviously), but also the returnCards method. If you use 10 players with 13 cards each in their hands, the last two players should get exactly the same hands as the first two. For example: Player 1 gets [75, 2C, JS, QD, 100, 9s, 3D, 6D, KC, AS, 2s, QC, 8D] Player 2 gets [6H, TH, JH, 5D, 5H, JS, 5S, QD, 20, 8H, AC, 41, 3C] Player 3 gets [75, 90, 2D, 2D, 10D, 105, 5C, 85, 6C, AD, KD, 7D, 6H] Player 4 gets [2H, QH, 9H, KS, 10H, JH, 100, KS, 8D, KH, 95, 3C, 5C] Player 5 gets [6S, AD, JC, 8C, 6D, 7H, 5D, 4D, 40, 40, 9H, 2H, KH] Player 6 gets [7C, AC, 3H, 35, QC, 89, 4H, JD, JD, JC, 9C, 3D, QS] Player 7 gets [10s, 45, 7C, AS, 3H, AH, 9D, KD, 8H, 39, 40, 5H, 7D] Player 8 gets [KC, 10H, QH, 6C, AH, 2s, 8C, 45, os, 65, 9D, 5S, 10D] Player 9 gets [75, 2C, JS, QD, 100, 9s, 3D, 6D, KC, AS, 2S, QC, 8D] Player 10 gets [6H, 7H, JH, 5D, 5H, JS, 5S, QD, 2C, 8H, AC, 41, 3C] First Sample -- no shuffle, no sort Simple Card Game This game just deals cards. First game How many players are in the game? 4 How many cards does each player get? 8 Player 1 gets [AS, AH, AD, AC, 25, 2H, 2D, 2C] Player 2 gets [35, 3H, 3D, 3C, 45, 41, 4D, 4C] Player 3 gets [5S, 51, 5D, 5C, 65, 6H, 6D, 6C] Player 4 gets [75, 7H, 7D, 70, 85, 8H, 8D, 8C] Top card from deck: 95 Second game How many players are in the game? 102 How many cards does each player get? 132 Player 1 gets [5H, 3D, 10D, KD, 25, QC, 2C, AC, 75, 3D, 10H, JD, AS] Player 2 gets [KD, 8H, KS, JH, AS, 7D, 9s, 2D, KC, 2H, 2C, 105, 5C] Player 3 gets [6D, 4H, QH, 2H, 8H, 6H, 89, 30, 3C, 100, 7H, 5H, 3S] Player 4 gets [45, 10D, 7D, 41, 9D, KC, 5s, 9C, JH, 9H, 40, 9C, 8C] Player 5 gets [6S, 9H, 6H, 6C, JS, 6S, 7H, QD, 8D, 7S, QH, JD, AD] Player 6 gets [35, 2D, AC, KS, 100, 5C, AH, 8D, 5D, 4C, 6C, 4D, QS] Player 7 gets [9D, Qs, 5s, 5D, 99, 45, 8S, JC, JS, 70, QC, 8C, 10H] Player 8 gets [AD, 70, 25, 105, QD, 3H, AH, KH, JC, 6D, KH, 3H, 4D] Player 9 gets [5H, 3D, 10D, KD, 2S, QC, 2C, AC, 75, 3D, 10H, JD, AS] Player 10 gets [KD, 8H, KS, JH, AS, 7D, 9S, 2D, KC, 2H, 20, 105, 5C] Second Sample -- shuffle, no sort Simple Card Game This game just deals cards. First game How many players are in the game? 42 How many cards does each player get? 8 Player 1 gets [2D, 8C, 20, 50, 6H, KS, 105, 10C] Player 2 gets [4C, KC, 70, 8H, 9s, 5D, JH, 10H] Player 3 gets [2H, JC, 8D, 5S, JD, 9D, KD, 4H] Player 4 gets [AS, 7D, 3D, QH, 5H, 85, 6S, QC] Top card from deck: 4D Second game How many players are in the game? 10 How many cards does each player get? 132 Player 1 gets [2H, 9C, 6C, 51, 8D, 100, 35, 6D, JH, 7H, 5, 4D, KH] Player 2 gets (JD, 75, 2D, 8C, 7H, 5S, KD, 8H, Qs, 9C, 3S, AS, 3C] Player 3 gets [KH, JC, 7D, 4H, 10H, 5S, KC, 89, 100, 99, KD, 5D, AS] Player 4 gets [JH, 65, 5C, 89, 4S, QD, 7C, JS, 105, AH, 4D, QH, KS] Player 5 gets [8D, 9D, AD, 5C, 4C, JD, 95, AC, 4C, 3H, 7C, 2S, JC] Player 6 gets [25, 7D, 9D, 3C, 45, QC, QC, 10H, 4H, 75, 10D, 20, 2C] Player 7 gets (JS, 2H, 5D, 9H, 105, 3H, QS, 8C, QH, 3D, 6C, 2D, AC] Player 8 gets [6H, 8H, KS, KC, QD, 10D, 6S, 3D, AH, AD, 6D, 9H, 6H] Player 9 gets [2H, 90, 60, 5H, 8D, 100, 35, 6D, JH, 7H, 5H, 4D, KH] Player 10 gets [JD, 75, 2D, 8C, 7H, 5S, KD, 8H, Qs, 90, 3S, AS, 3C] Third Sample -- shuffle, sort (example 1) Simple Card Game This game just deals cards. First game How many players are in the game? 44 How many cards does each player get? se Player 1 gets [6S, JH, 4D, 40, 60, 70, 90, QC] Player 2 gets [55, 75, 9S, AH, QH, AD, JD, KC] Player 3 gets [85, 9H, KH, 8D, 9D, AC, 30, 5C] Player 4 gets [45, 105, JS, KS, 2D, 7D, 2C, 8C] Top card from deck: 8H Second game How many players are in the game? 102 How many cards does each player get? 13 Player 1 gets (AS, 45, 85, 95, 105, 105, 7H, 6D, 6D, 10D, KD, AC, 2C] Player 2 gets [39, JS, KS, 2H, 6H, 7H, 9H, QH, KH, KH, AD, 5D, QC] Player 3 gets [35, 5S, 75, 9H, JH, AD, 2D, 3D, JD, OD, 20, 3C, 70] Player 4 gets [2s, os, KS, 3H, 3H, 8H, QH, 7D, 8D, 8D, 9D, QD, AC] Player 5 gets [55, 85, Q5, 6H, 10H, 4D, 10D, JD, 40, 80, 90, JC, KC] Player 6 gets [6S, JS, AH, AH, 5H, 10H, 3D, 5D, 5C, 60, 60, 90, 10C] Player 7 gets [2S, 75, 4H, 8H, JH, KD, 30, 40, 70, 80, JC, QC, KC] Player 8 gets [AS, 45, 65, 9s, 2H, 41, 5H, 2D, 4D, 7D, 9D, 5C, 10C] Player 9 gets [AS, 48, 89, 99, 105, 105, 7H, 6D, 6D, 10D, KD, AC, 2C] Player 10 gets [3S, JS, KS, 2H, 6H, 7H, 9H, QH, KH, KH, AD, 5D, QC] Fourth Sample -- shuffle, sort (example 2) Simple Card Game ================ This game just deals cards. First game How many players are in the game? 42 How many cards does each player get? Player 1 gets [6H, 9H, 107, 7D, 9D, 6C, 10C, KC] Player 2 gets [35, 105, gs, JH, AD, 2D, 10D, AC] Player 3 gets [AS, 55, AH, 7H, 5D, 6D, 30, 5C] Player 4 gets [45, 75, 5H, 4D, 8D, 40, 80, 9C] Top card from deck: 4H Second game How many players are in the game? 102 How many cards does each player get? 132 Player 1 gets [2S, 4S, 89, 7H, 10H, KH, 2D, 4D, 5D, 6D, AC, AC, QC] Player 2 gets [KS, AH, 3H, 5H, AD, 3D, 4D, 7D, 9D, 6C, 8C, JC, QC] Player 3 gets (105, KS, 3H, JH, QH, 2D, 10D, JD, JD, QD, 3C, 6C, JC] Player 4 gets [AS, 35, 5s, 5s, 10s, 47, 6, 8H, 3D, 6D, QD, 2C, 10C] Player 5 gets [AS, 3S, 6S, 9S, AH, 2H, JH, KD, 20, 40, 50, 70, 7C] Player 6 gets [2s, 45, 9s, JS, 4H, 8H, 9H, KH, 5D, 9D, 10D, 8C, KC] Player 7 gets [8S, JS, Qs, 2H, 5H, 6H, 10H, AD, 8D, KD, 30, 40, 9C] Player 8 gets [6S, 75, 75, qs, 7H, 9H, QH, 7D, 8D, 5C, 9C, 10C, KC] Player 9 gets [2S, 48, 89, 7H, 10H, KH, 2D, 4D, 5D, 6D, AC, AC, QC] Player 10 gets [KS, AH, 3H, 5H, AD, 3D, 4D, 7D, 9D, 6C, 80, JC, QC]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
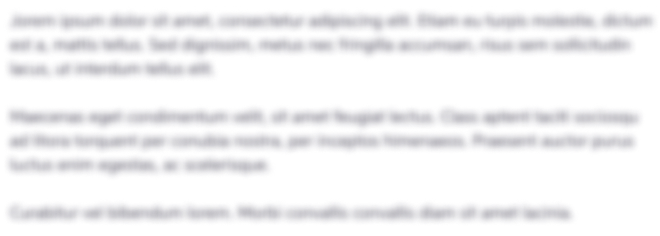
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started