Question
ArrayQueue Code: import exceptions.EmptyCollectionException; public class ArrayQueue implements QueueADT { private T queueArray[]; // Array holding queue private static final int DEFAULT_SIZE = 5; private
ArrayQueue Code:
import exceptions.EmptyCollectionException;
public class ArrayQueue implements QueueADT {
private T queueArray[]; // Array holding queue private static final int DEFAULT_SIZE = 5; private int front; // position of element at the front of the queue private int rear; // First free position at rear of the queue private int size; // number of elements in the queue
@SuppressWarnings("unchecked") public ArrayQueue(int initialSize) { front = -1; rear = 0; queueArray = (T[]) new Object[initialSize]; }
public ArrayQueue() { this(DEFAULT_SIZE); }
@Override public void enqueue(T element) { // TODO Auto-generated method stub if (isFull()) { growQueue(); }
if (isEmpty()) { front = (front + 1) % queueArray.length; }
queueArray[rear] = element; rear = (rear + 1) % queueArray.length; size++;
}
@SuppressWarnings("unchecked") private void growQueue() { Object[] temp = new Object[queueArray.length + DEFAULT_SIZE]; int index = front; for (int i = 0; i
} queueArray = (T[]) temp; } private boolean contains(T element) {
int index = front;
for (int c = 0; c
return false;
}
private int indexOf(T element) {
int index = front;
for (int c = 0; c
return -1;
}
@Override public T dequeue() { // TODO Auto-generated method stub if (isEmpty()) { throw new EmptyCollectionException("array queue"); } T retElement = queueArray[front]; queueArray[front] = null; front = (front + 1) % queueArray.length; size--; return retElement; }
@Override public T first() { // TODO Auto-generated method stub if (isEmpty()) { throw new EmptyCollectionException("array queue"); } return queueArray[front]; }
@Override public boolean isEmpty() { // TODO Auto-generated method stub return (size == 0); }
private boolean isFull() { return (size == queueArray.length); }
@Override public int size() { // TODO Auto-generated method stub return size; }
public String toString() { String retString = "["; int index = front;
for (int c = 0; c
retString += "]"; return retString; }
}
QueueADT Code:
public interface QueueADT {
public void enqueue(T element); public T dequeue(); public T first(); public boolean isEmpty(); public int size(); }
QueueTesterCode:
public class QueueTester {
public static void main(String[] args) { //test both queues
//LinkedQueue
System.out.println("Dequeue [4]: " + lq.dequeue()); System.out.println("First [5]: " + lq.first()); System.out.println("Queue [5 6 7]: " + lq); System.out.println("Dequeue [5]: " + lq.dequeue()); System.out.println("First [6]: " + lq.first()); System.out.println("Queue [6 7]: " + lq); lq.enqueue(8); lq.enqueue(9); System.out.println("Queue [6 7 8 9]: " + lq);
}
}
+ toArray(T[] anArray) : void - Returns an array containing all of the elements in this queue in proper sequence (from front to rear element). This method may throw the following exceptions: a. NullPointerException- if the array is null (use the java NullPointerException) b. ArrayCapacityException - if the size of the array is not sufficient to store the elements of the queue (create this exception) Modify the Stack tester to include scenarios to test the new methods. Make sure you test multiple states in your stack, such as an empty stack, elements occurring or not occurring in the stack, adding enough elements to force the growQueue() method, and returning the array. Use a try/catch block to handle the exceptions appropriately. + toArray(T[] anArray) : void - Returns an array containing all of the elements in this queue in proper sequence (from front to rear element). This method may throw the following exceptions: a. NullPointerException- if the array is null (use the java NullPointerException) b. ArrayCapacityException - if the size of the array is not sufficient to store the elements of the queue (create this exception) Modify the Stack tester to include scenarios to test the new methods. Make sure you test multiple states in your stack, such as an empty stack, elements occurring or not occurring in the stack, adding enough elements to force the growQueue() method, and returning the array. Use a try/catch block to handle the exceptions appropriatelyStep by Step Solution
There are 3 Steps involved in it
Step: 1
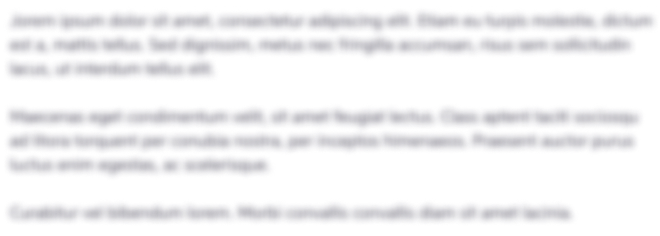
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started