Question
Arrays (array.c) Write a program that tests your mastery of the concept of static array ...not dynamic (heap). Your program will fill a 1000 element
Arrays (array.c)
Write a program that tests your mastery of the concept of static array...not dynamic (heap). Your program will fill a 1000 element integer array with either random numbers (between 1 and 1000) or the numbers from 1000 to 1 (in the decreasing order) based on user choice; and then proceed to find some statistics on these numbers. In the beginning your program should display the following prompt message.
********************OUTPUT***********************************
Enter a choice for the type of array you want to create:
1: Random Number Array
2: Fixed Number Array
Choice:
***************************************
Then based on the user choice you will use one the following functions to create the array of user choice:
void fill random(int *array);
void fill fixed(int *array);
These functions receive an empty array as argument and populate it with either random or fixed numbers. You should use a define preprocessor directive to define a constant with the value 1000 for setting the array size. You will need to write several functions detailed below to include: a sort function (that sorts in the increasing order of the elements), minimum, maximum, median, mean, standard deviation, summation function, and a print function. You will need to find the minimum and maximum numbers from the sorted array (in a sorted array, the minimum element is the first element of the array, and the maximum element is the last element of the array). The choice of sorting algorithms is left up to the programmer.
The IMAGE shows functions that MUST be implemented as they are, WITHOUT changing them.
Program Language in C...not C++
************This is what I have so far if it helps....but I don't know what I'm really doing. Im trying to figure it out. ***********
#include
#define CAPACITY 1000
void sort(int *array); void print(int *array); double mean(int *array); double median(int *array); double min(int *array); double max(int *array); void fill_random(int *array); void fill_fixed(int *array); int sum(int *array); double stdDev(int *array);
int main(void) { // ENTER VARIABLES srand(time(NULL)); int choice, size; int sum = 0; int min, max; double median = 0, mean = 0, stdDev; int myArray[1000]; // GET ARRAY CHOICE printf("Enter a choice for the type of array you want to create: "); printf("1: Random Number Array "); printf("2: Fixed Number Array "); printf("Choice: "); scanf("%d", &choice); switch (choice) { case 1: printf("Enter the amount of numbers you'd like to find stats for: "); scanf("%d", &size); fill_random(&myArray); case 2: printf("Enter the amount of numbers you'd like to find stats for: "); scanf("%d", &size); fill_fixed(&myArray); default : printf("Invalid Input! "); exit(1); } mean = mean(myArray); median = median(myArray); min = min(myArray); max = max(myArray); sum = sum(myArray); stdDev = stdDev(myArray); print(myArray); sort(myArray); printf("Median: %.2f ", median); printf("Mean: %.2f ", mean); printf("Minimum: %d ", min); printf("Maximum: %d ", max); printf("Standard Deviation: %.2f ", stdDev); return 0; }
void sort(int *array) { int i, j, t; int size; for (i = 1; i = array [j]) { t = array[j - 1]; array[j - 1] = array[j]; array[j] = t; } } } }
void print(int *array) { int i; int size; printf("["); for (i = 0; i 0) { printf("%d", array[size - 1]); } printf("] "); }
double mean(int *array) { int size; return sum / size; }
double median(int *array) { sort(array); int median = array[size/2]; return median; }
double min(int *array) { sort(array); int double max(int *array){
}
// Generate Random Array void fill_random(int *array) { int i; int size; int *array = NULL; array = (int *) malloc(sizeof(int) * size); for (i = 0; i
// Generate Fixed Number of Array void fill_fixed(int *array) { int i; int size; printf("Enter your list of numbers: "); for (i = 0; i
int sum(int *array) { int sum = 0; int i = 0; int size; for (i = 0; i
double stdDev(int *array) {
Implement these functions: void sort (int array); void print (int array); double mean (int array); double median (int *array); int min int *array) int max int *array) void fill random (int *array) void fill-fixed int *array) int sum (int *array) double stdDev (int *array) You main function should follow the following flow of the program Get array choice Fill array using a function Print unsorted array Sort the array using a function Print sorted array Using appropriate functions compute median, mean, minimum, maximum, and standard deviation Print median Print mean Print minimum Print maximum Print standard deviation
Step by Step Solution
There are 3 Steps involved in it
Step: 1
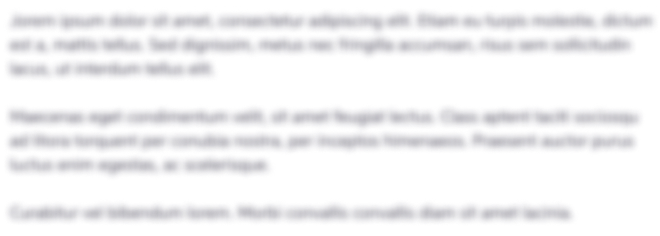
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started