Answered step by step
Verified Expert Solution
Question
1 Approved Answer
As a convenience for you we have already created an inner / nested class for you called SListNode. You are free to modify this class
As a convenience for you we have already created an innernested class for you called SListNode. You are free to modify this class as you see fit, but it should remain an inner class to the SList class. Note that this kind of encapsulation is great for readability, maintainability, and portability. Step The SList Class The SList class is the most significant piece of this project. As mentioned above you would be wise to implement this class as though it will only ever store int values. This will make it easier to think about, and we will see that thanks to dynamic typing will be enough to make it work in the general case as well if we are just a little bit careful. SList must implement the following methods: insertitem : item is inserted in the list at the appropriate location to maintain a nondecreasing ordering based on whatever key the item is compared on If two items are considered identical, the newer item should appear AFTER the older item ie ascending order of arrival time For instance if the list contains the integers and in this case the value of the integer is used to establish an ordering and an additional is inserted, then the list would contain and the second would be the newly inserted For simple integers this doesnt matter but for more complex objects it might. This function does not return a value removekey : Removes the first occurrence of an item as indicated by the key from the list. Returns True if an item was found and removed, False if not. removeallkey : Removes ALL occurrences of an item as indicated by the key from the list. Doesnt return a value. size : Returns the current number of itemsnodes in the list. findkey : Searches for the first occurrence of an item as indicated by the key value in the list. If found, the item is returned; returns None otherwise. str : Returns a string representation of the contents of the list. This string should start with and end with and the string representations of the list items should be comma separated, and listed in order. Note the somewhat recursive nature of this method as it will need to call str on the individual list items... We recommend implementing these methods and creating simple unit tests on your own to validate their behavior. Step Make it Iterable In addition to the methods listed above, your SList class must support several of the goodies that Python lists make available to us The first of these is that your SList class must be iterable so that it can be used in for loops etc. as we are accustomed to doing with regular Python lists. For a class to be iterable it must implement or otherwise provide access to the following methods: iter : Returns an iterator object something that has implemented next next : Returns or yields the next value in a sequence in this cas the next value in the list. may not be explicitly necessary if you choose to implement a generator function Step Show us the Brackets! Being able to access an item at a give position in a list is an extremely useful capability. You must extend SList to support position based indexing via the square bracket notation we are used to eg theList To do this you must implement the getitem magic method as follows getitemindex : Returns the value stored at position index in the list. Once again we suggest that you implement these methods and test your code to validate its performance. The issorted function provided in the supplied pmaintemplate.py file may be useful to you at this point.
As a convenience for you we have already created an innernested class for you called SListNode. You are free to modify this class as you see fit, but it should remain an inner class to the SList class. Note that this kind of encapsulation is great for readability, maintainability, and portability.
Step The SList Class
The SList class is the most significant piece of this project. As mentioned above you would be wise to implement this class as though it will only ever store int values. This will make it easier to think about, and we will see that thanks to dynamic typing will be enough to make it work in the general case as well if we are just a little bit careful. SList must implement the following methods:
insertitem : item is inserted in the list at the appropriate location to maintain a nondecreasing ordering based on whatever key the item is compared on If two items are considered identical, the newer item should appear AFTER the older item ie ascending order of arrival time For instance if the list contains the integers and in this case the value of the integer is used to establish an ordering and an additional is inserted, then the list would contain and the second would be the newly inserted For simple integers this doesnt matter but for more complex objects it might. This function does not return a value
removekey : Removes the first occurrence of an item as indicated by the key from the list. Returns True if an item was found and removed, False if not.
removeallkey : Removes ALL occurrences of an item as indicated by the key from the list. Doesnt return a value.
size : Returns the current number of itemsnodes in the list.
findkey : Searches for the first occurrence of an item as indicated by the key value in the list. If found, the item is returned; returns None otherwise.
str : Returns a string representation of the contents of the list. This string should start with and end with and the string representations of the list items should be comma separated, and listed in order. Note the somewhat recursive nature of this method as it will need to call str on the individual list items...
We recommend implementing these methods and creating simple unit tests on your own to validate their behavior.
Step Make it Iterable
In addition to the methods listed above, your SList class must support several of the goodies that Python lists make available to us The first of these is that your SList class must be iterable so that it can be used in for loops etc. as we are accustomed to doing with regular Python lists. For a class to be iterable it must implement or otherwise provide access to the following methods:
iter : Returns an iterator object something that has implemented next
next : Returns or yields the next value in a sequence in this cas the next value in the list. may not be explicitly necessary if you choose to implement a generator function
Step Show us the Brackets!
Being able to access an item at a give position in a list is an extremely useful capability. You must extend SList to support position based indexing via the square bracket notation we are used to eg theList To do this you must implement the getitem magic method as follows
getitemindex : Returns the value stored at position index in the list. Once again we suggest that you implement these methods and test your code to validate its performance. The issorted function provided in the supplied pmaintemplate.py file may be useful to you at this point.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
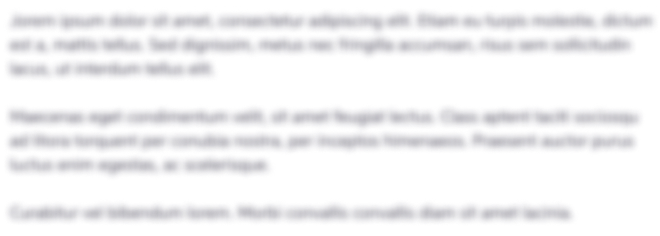
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started