Question
As in previous homeworks, you must use the names of fields (and all other variables and methods) as they are exactly specified in this document.
As in previous homeworks, you must use the names of fields (and all other variables and methods) as they are exactly specified in this document.
Note also for method names that if you have a variable called numberOfOwners, then a mutator for this field is called setNumberOfOwners and the corresponding accessor is called getNumberOfOwners.
Scenario: The Thrifty Club is a private members-only club that runs grocery stores. To gain entry to a store, a member has to place his membership card in a card reader and enter his pin number. The member can then enter the store, select an item of food or drink and go to the checkout to pay for the item. For this part of the homework, any member is restricted to selecting a single item.
You are to model this scenario using a BlueJ project called Thrifty Club following the steps below.
Step 1: a simple Member class
Write a simple Member class which has three private fields called name, id and pinNumber. The member ID consists of a unique sequence of letters and digits* of length at most 10 and the pin number consists of a sequence of four digits (which could start with the digit zero. The constructor for the class should be passed suitable arguments to initialise these three fields in the order specified above whenever a Member object is created.
The class should include suitable accessor & mutator methods. It should also include 2 methods for checking the correct input for memberID and pinNumber(). If the criteria for the memberID and pinNumber, is not met, the member should not be created. In future steps, you will not necessarily be reminded to include, for example, accessor methods. You will be expected to provide any necessary methods and write sensible code following the general principles and conventions you have been studying in this module. Note also, the general programming principle here of keeping the number of fields to only those that are needed. Do this throughout this project.
Step 2: A simple Store class
- Write a simple Store class to represent the store. The constructor for the class should be passed the name of the store which is then stored in a field called storeName. A second field total should record the amount of money taken at the checkout.
- Write a method for the class, called memberRegister1() which allows the member to gain entry to the store. This method is passed an argument that is a pointer to a Member object. At this stage, simply get this method to output a welcome message to a terminal window in a format identical to the example below:
Salford Thrifty Store welcomes Andy (id:SGT006732)
where Salford Thrifty Store is the name of the store, Andy is the name of the member whose ID then follows.
c) Next, write a method for the class, called memberRegister2() which also allows the member to gain entry to the store. However, this method differs from the previous method in that is passed the member's name, id and pin number as parameters in this particular order and then creates a Member object which, for now, is temporarily stored in a local variable called member.
Again, write the same welcome message in a format identical to the example above.
Now you have two ways of allowing a member to enter the store but at the moment the Store object does not record which member has entered the store and, conversely, the Member object also keeps no details of which store it has entered. Lets deal with that now.
Step 3: Enhancements to the Store and Member classes
- Add a fourth field store to the Member class. This field is of type Store and will be used to hold a reference to the store the member has entered.
- Write a mutator method setStore()which is passed a pointer called store to the store into which the member has entered.
- Read the whole of this part c) before trying to write any code.
Now this method, setStore() needs to be called from both memberRegister() methods which are in the class Store. So, each of these calls is a call to a method in another class and so both are external method calls.
Next, for each of these calls, think about what the argument to this method should be.
Well, setStore() needs to be passed, as an argument, a pointer to the Store object that you are currently in.
In fact, the Store object needs to be able to tell the Member object "point to me". To do this, you need to use a self-referencing pointer.
Now if you understand the explanation above you can write both calls to setStore() in Store.
In the following steps, examples are shown using prices and other amounts of money that may be less than one pound (100p) in which case they are shown in the form 65p or they may be greater than or equal to a pound in which case they are shown in the form 309p.
Step 4: Add a simple Item class and allow a member to select an item.
- Write a simple Item class to represent an item of food or drink. An Item object has two fields, name and price -- the latter should be stored as an int representing pence. The constructor for the class should be passed arguments to initialise these two fields in the order specified above whenever an Item object is created.
- Write an accessor method getPriceString() that simply returns the price of an item as a String in pounds and pennies.
- Add a selectItem() method to the Member class which is passed an Item object as a parameter. You should declare a new field item in the Member class in order to store the item selected. Finally, for this step, make the selectItem() method print a message to a terminal window which outputs the name of the member, the item purchased and the cost of that item on a single line in the exact format given here:
Andy has selected Wholemeal Medium Sliced Loaf at 54p
or
Andy has selected Fresh Whole Chicken at 304p
depending on whether or not the item costs less (or more) than a pound.
Do not proceed to Step 5 until you have successfully tested Step 4 by creating a Member object and an Item object and tested selectItem().
Step 5: Allow a member to buy the item.
- Add an extra field money to the Member class to represent the amount of money the member has in their wallet or purse. Initialise the field with 500 pence.
- Add a method checkout() to the Store class which is passed two parameters: an int representing the amount of money tendered to pay for the item and a Member object representing the member at the checkout in that order. This amount of money may be different to the cost of the item e.g. the member may tender a 1 coin (represented by 100p) to pay for an item of value 54p. The checkout() method should print a message to a terminal window similar to the example shown below:
Salford Thrifty Store: Serving Andy
Your basket contains
Fresh Whole Chicken at 304p
You have tendered 400p
Your change is 96p
- Add a method goToCheckout() to the Member class which is passed an int parameter called paymentrepresenting the money the member is tendering to pay for the item. This amount should be checked and appropriate messages to be printed for different situations based on the price of the item. This method then calls the method checkout() of the Store class: again an external method call very similar to the call in Step 3 c) above.
Finally !
Note that not every detail to make this code more realistic is mentioned here ! -- you need to think more about the shopping situation: re-read the specification and see whether there are any parts that you have not yet fully implemented.
[1] You may assume, without checking, that the customer id is unique and does consist of letters and digits.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
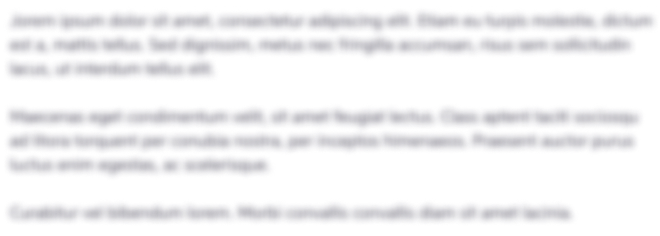
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started