Question
Assembly Programming Review: Write a MIPS Assemble Program for a Solution The goal is to design and implement a project that translates a small, limited
Assembly Programming Review: Write a MIPS Assemble Program for a Solution
The goal is to design and implement a project that translates a small, limited computer language with only a few reserved word and limited arithmetic capabilities and limited functionality that your team creates into assembly code for a specific platform. This assignment prepares students to understand and write assemble code in the MIPS Assembly Code Language. MIPS is a Reduced Instruction Set Computer (RISC) based Assembly Language.
To assist students with this assignment, I provided a link to a Java-based MIPS Compiler and IDE. The name of the IDE with the built-in Compiler is MARS. I provided a link to download the MARS package. To use MARS, students must have the JAVA run time components installed on their computers. Most computer science majors should have a version of the JAVA Development Kit (JDK) installed already. If not, I recommend installing a version of the JDK that will include the JAVA runtime component.
Assignment Topic:
- Convert a base 10 decimal number, represented as a string (e.g. '42'), to its Binary equivalent. (e.g. '101010'),
Program Requirements
- The program should display your name at the top of the output stream
- The program should prompt for the Base 10 Integer input
- The program should covert and display the converted result.
- Link to Download a copy of a Sample Lab Report Template
- Lab Report Template.doc
Submit the Following Artifacts
- Text File containing the Assembly Code for the assignment
- Screenshot of the programs output in your personal development environment
- Lab Report
My MIPS/MARS code so far (the binary conversion does work correctly):
#Decimal To Binary Converter .data prompt: .asciiz "Enter a base 10 integer: " result: .asciiz "The binary equivalent is: " name: .asciiz "Name "
.text .globl main main: # Print name (you need to declare your name above) li $v0, 4 la $a0, name syscall
# Print prompt li $v0, 4 la $a0, prompt syscall
# Read integer li $v0, 5 syscall move $s0, $v0
# Print result li $v0, 4 la $a0, result syscall
# Convert to binary and print result li $t0, 0 # initialize counter loop: and $t1, $s0, 1 # get last bit sll $t2, $t0, 1 # shift counter or $t2, $t2, $t1 # or with last bit move $t0, $t2 # update counter srl $s0, $s0, 1 # right shift input beqz $s0, end # break loop when input is 0 j loop # repeat loop end: li $v0, 1 # print integer move $a0, $t0 syscall
# Exit program li $v0, 10 syscall
Step by Step Solution
There are 3 Steps involved in it
Step: 1
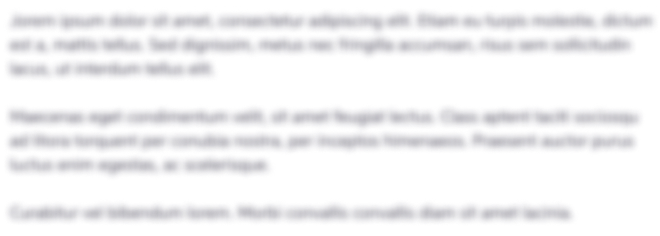
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started