Question
/**** Assignment 1 C PROGRAMMING Program Description: This program appears to be a simple statistics program that calculates and prints the total album sales for
/**** Assignment 1 C PROGRAMMING
Program Description: This program appears to be a simple statistics program that calculates and prints the total album sales for five different artists in four different countries. The program starts by defining several arrays: "countries" contains the names of the four countries being considered "artists" contains the names of the five artists being considered "usa", "canada", "uk", and "australia" are arrays containing the sales data for each artist in their respective country\ The program then uses a nested loop structure to iterate through the sales data arrays, adding up the total sales for each artist and each country. The totals are stored in the "artistTotals" and "countryTotals" arrays. Finally, the program prints the results in a formatted table, with the artist and country names as well as the sales totals for each artist in each country, and the grand total for all artist and countries. ****/
#include
int main(void) { // Initialize arrays to hold names of countries and artists char *countries[] = { "USA", "Canada", "UK", "Australia"}; char *artists[] = {"Ed Sheeran", "Drake", "Eminem","Taylor Swift", "The weekend"}; // Initialize arrays to hold streaming amounts for each artist in each country int usa[] = {871, 1590, 1190, 993, 1140}; int canada[] = {105, 109, 150, 102, 126}; int uk[] = {291, 140 , 229, 98, 157}; double australia[] = {97.7, 43.2, 97.5, 66.5, 71.0}; // Initialize arrays to hold artist & country totals as well as the sum of all stream total double artistTotal[5] = {0}; double countryTotal[4] = {0}; double allStreamTotals [5] = {0}; //Display the title header for the table where all data are stored printf("%25s %s %s" , "YouTube" , "Most Streamed" , " Artists of 2021 "); printf("%27s %s" , "by" , "Country (millions) "); // Printing the header row for the table printf("%-15s %10s %10s %10s %13s %10s " ,"Artist", countries[0], countries[1], countries[2], countries[3], "Total" ); // Loop through each country and Calculate the total streams for the artist across all artists for (int i = 0; i<5; i++){ artistTotal[i] = usa[i] + canada[i] + uk[i] + australia[i]; // Print the artist name and their streaming counts for each country printf("%-15s %10d %8d %13d %10.1f %12.1f " , artists[i], usa[i], canada[i], uk[i], australia[i],artistTotal[i]); } // Loop through each country and Calculate the total streams for the country across all artists // Loop through artistTotal and sum up for (int i = 0; i < 5; i++){ countryTotal[0] = usa[i] + countryTotal[0]; countryTotal[1] = canada[i] + countryTotal[1]; countryTotal[2] = uk[i] + countryTotal[2]; countryTotal[3] = australia[i] + countryTotal[3]; allStreamTotals [0] = artistTotal[0] + artistTotal[1] + artistTotal[2 ]+ artistTotal[3] + artistTotal[4]; } // Print the total streams for each country and the overall total streams across all countries printf("%-15s %10.f %8.f %13.f %10.1f %12.1f ", "Totals", countryTotal[0], countryTotal[1], countryTotal[2], countryTotal[3], allStreamTotals[0]); return 0; }
PROBLEM:
You are to re-write the code for assignment #1, to break it into the following functions (these exact functions must be used):
a function to find the total sales for one country and return that to main( ). This function will be called 4 times while the code executes. Create an array to hold these totals.
you will need 2 versions of this function (code 2 separate functions that are very similar except for the type of the array it receives and works with). One function will work with an int [ ], the other version of the function will receive a float/double [ ]
a function to find the total sales for one artist and return that to main( ). This function will be called 5 times while the code executes. Think carefully about the parameter list. Create an array to hold these totals.
a function that will find the artist that had the highest combined sales (for the 4 countries). This function will output the highest combined sales, and the artist that got that total, AFTER the table.
a function that will find the grand total, in the bottom right corner, and return it to main( ) for printing. What is the most efficient way to do that?
Notes:
the 4 functions described above MUST come after the main( ) function
Function prototypes must be used in your solution.
Think carefully about what the parameter lists must be for each function. The function should be sent only the information it needs to complete its task.
This will be marked out of 5. Marks will be assigned based on the functions used in the code, and if the parameter lists are correct.
Marks will continue to be lost for programs that do not conform to good programming practices.
Global variables are not allowed.
please comment on code for easy understanding
See assignment #1 for a statement of the problem being solved.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
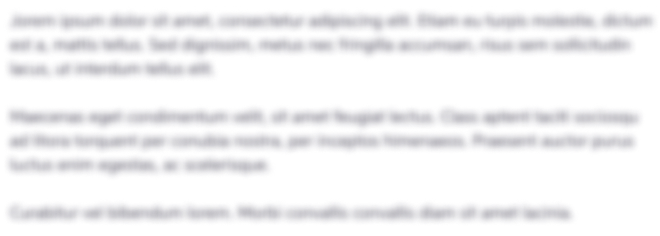
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started