Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment 1 Selection Sort In this assignment, you will write code to read a series of integers into an array, sort the array using the
Assignment Selection Sort
In this assignment, you will write code to read a series of integers into an array, sort the array
using the Selection Sort algorithm, and then print the numbers in a formatted fashion.
Obtaining Assignment Files on Unix
Log in to Unix.
Run the setup script for Assignment by typing:
setup
Files for this assignment can also be downloaded to your local machine from the GradeoMatic assignment page.
Input
The input for this program will be provided on the standard input stream using input
redirection. Input files for this assignment will contain a series of integers separated by
whitespace.
Files We Give You
The setup script will create the directory Assign under your csci directory. You will
receive a file named main.cpp that contains a main function to call the functions you will
write for this assignment described in the section below a makefile named makefile that
can be used to build the executable program, and symbolic links to a number of sample data
files that you can use to test your program.
You should assume that the GradeoMatic may test your program using data files other than
the ones supplied as part of this assignment. The data files are named randomtxt
randomtxt randomtxt etc., with the numeric part of the file name specifying the
number of random values that the file contains.
On Unix, the makefile can be used to build the executable program by simply typing the
word make. Running the command make clean will remove all of the object and executable
files created by the make command.
Files You Must Write
You will write one file for this assignment called selectionsort.cpp This file must contain
definitions for the following three functions:
int buildarrayint array
This function should read a series of integers from the standard input stream using cin
into the elements of the array of integers named array until end of input is reached.
You may assume that there will be a maximum of integers to read and that the
array will be large enough to hold all of them.
The function should return the number of integers that were read into the array.
void printarrayint array int n
This function should print the first n elements of the array of integers named array.
The output should be printed with eight numbers per line, right justified and aligned in
columns. Use the setw manipulator to print numbers with a width of eight characters,
padded with leading spaces. Print a newline character
at the end of each line. Note
that the last line may have less than eight numbers; if so it should still end with a
newline character.
void selectionsortint array int n
This function should sort the first n elements of the array of integers named array in
ascending order using the Selection Sort algorithm. Note that if you use a different
sorting algorithm or call a library function to do the sorting, you will not receive full
credit for this assignment even if your code successfully sorts the integers.
The file selectionsort.cpp is the only file that should be submitted to the GradeoMatic.
When you submit it it must not contain a main function. The autograder will supply its
own version of main to call and test your functions, so if the code that you submit also
contains a main function, you will get a linker error and the executable program will not be
built successfully.
Example Output
To run the executable program on Unix, type a command similar to the following:
selectionsort randomtxt
The command above will run the executable file selectionsort using the data file
randomtxt as redirected input.
Output from the program should look like this:
Note that your program must always supply a newline character at the end of each line, even
if the last line contains less than eight numbers.
Hints
Pseudocode for the Selection Sort algorithm is available on the course web site.
It may be easier to complete this assignment if you break it down into steps. Do one thing at a
time, convince yourself that it works, and then move onto the next step. Below is an ordered
sequence of steps that you might want to try for this part of the assignment.
Write skeleton versions of the three functions. Copy the first line of each function from
the description given above. The function body can either be empty or simply return a
default value of the correct data type, eg:
int buildarrayint array
return ;
Your goal in this step should be to write just enough code to get the program to
successfully build without syntax or linker errors. That should be sufficient to pass the
name and make tests on the GradeoMatic. You should expect the various diff
tests to fail at this stage.
Write code for the buildarray function to read the values into the array, count
Step by Step Solution
There are 3 Steps involved in it
Step: 1
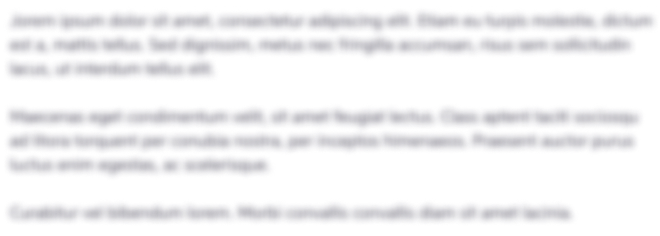
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started