Question
Assignment 2 In chapter 9 of your Deitel & Deitel text, you studied an inheritance hierarchy in which class BasePlusCommissionEmployee inherited from class CommissionEmployee. However,
Assignment 2
In chapter 9 of your Deitel & Deitel text, you studied an inheritance hierarchy in which class BasePlusCommissionEmployee inherited from class CommissionEmployee. However, not all types of employees are CommissionEmployees.
In this exercise, youll create a more general Employee superclass that factors out the attributes and behaviors in class CommissionEmployee that are common to all Employees. The common attributes and behaviors for all Employees are firstName, lastName, socialSecurityNumber, getFirstName, getLastName, getSocialSecurityNumber and a portion of method toString.
Create a new superclass Employee that contains these instance variables and methods and a constructor.
Next, rewrite class CommissionEmployee from Section 9.4.5 as a subclass of Employee. Class CommissionEmployee should contain only the instance variables and methods that are not declared in superclass Employee. Class CommissionEmployees constructor should invoke class Employees constructor and CommissionEmployees toString method should invoke Employees toString method.
Once youve completed these modifications, run the BasePlusCommissionEmployeeTest app using these new classes to ensure that the app still displays the same results for a BasePlusCommissionEmployee object.
// Fig. 9.11: BasePlusCommissionEmployee.java // BasePlusCommissionEmployee class inherits from CommissionEmployee // and accesses the superclasss private data via inherited // public methods. public class BasePlusCommissionEmployee extends CommissionEmployee { private double baseSalary; // base salary per week // six-argument constructor public BasePlusCommissionEmployee(String firstName, String lastName, String socialSecurityNumber, double grossSales, double commissionRate, double baseSalary) { super(firstName, lastName, socialSecurityNumber, grossSales, commissionRate); // if baseSalary is invalid throw exception if (baseSalary < 0.0) throw new IllegalArgumentException( "Base salary must be >= 0.0"); this.baseSalary = baseSalary; } // set base salary public void setBaseSalary(double baseSalary) { if (baseSalary < 0.0) throw new IllegalArgumentException( "Base salary must be >= 0.0"); this.baseSalary = baseSalary; } // return base salary public double getBaseSalary() { return baseSalary; } // calculate earnings @Override public double earnings() { return getBaseSalary() + super.earnings(); } // return String representation of BasePlusCommissionEmployee @Override public String toString() { return String.format("%s %s%n%s: %.2f", "base-salaried", super.toString(), "base salary", getBaseSalary()); } } // end class BasePlusCommissionEmployee
-----------------------------------------------------------------------------------------------------------------
// BasePlusCommissionEmployeeTest.java // Testing class BasePlusCommissionEmployee. public class BasePlusCommissionEmployeeTest { public static void main(String[] args) { // instantiate CommissionEmployee object CommissionEmployee employee1 = new CommissionEmployee( "Sue", "Jones", "222-22-2222", 10000, .06); // get commission employee data System.out.println( "Employee information obtained by get methods:"); System.out.printf("%n%s %s%n", "First name is", employee1.getFirstName()); System.out.printf("%s %s%n", "Last name is", employee1.getLastName()); System.out.printf("%s %s%n", "Social security number is", employee1.getSocialSecurityNumber()); System.out.printf("%s %.2f%n", "Gross sales is", employee1.getGrossSales()); System.out.printf("%s %.2f%n", "Commission rate is", employee1.getCommissionRate()); employee1.setGrossSales(5000); employee1.setCommissionRate(.1); System.out.printf("%n%s:%n%n%s%n", "Updated employee information obtained by toString", employee1); // instantiate BasePlusCommissionEmployee object BasePlusCommissionEmployee employee2 = new BasePlusCommissionEmployee( "Bob", "Lewis", "333-33-3333", 5000, .04, 300); // get base-salaried commission employee data System.out.println( "Employee information obtained by get methods:"); System.out.printf("%n%s %s%n", "First name is", employee2.getFirstName()); System.out.printf("%s %s%n", "Last name is", employee2.getLastName()); System.out.printf("%s %s%n", "Social security number is", employee2.getSocialSecurityNumber()); System.out.printf("%s %.2f%n", "Gross sales is", employee2.getGrossSales()); System.out.printf("%s %.2f%n", "Commission rate is", employee2.getCommissionRate()); System.out.printf("%s %.2f%n", "Base salary is", employee2.getBaseSalary()); employee2.setBaseSalary(1000); System.out.printf("%n%s:%n%n%s%n", "Updated employee information obtained by toString", employee2.toString()); } // end main } // end class BasePlusCommissionEmployeeTest
Step by Step Solution
There are 3 Steps involved in it
Step: 1
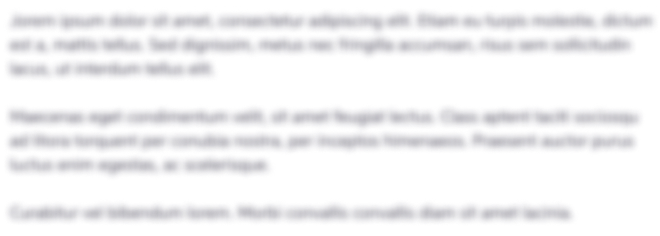
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started