Question
Assignment 4 Statement: Write a program in a file named Scan4.cpp that reads the inventory for a hardware store from a sequential access file named
Assignment 4 Statement: Write a program in a file named Scan4.cpp that reads the inventory for a hardware store from a sequential access file named scan.txt. Each line of the input file contains a string and a double separated by a blank character (you are guaranteed that the string has no embedded blanks). The string value should be interpreted as the name of a tool and the double value should be interpreted as the price of that tool. Your program should display each line of the file on the monitor using appropriate formatting. When all of the lines of the file have been processed, display the total number of tools in the file and the sum of all of the costs for all of the tools that were stored in the file. All output for this program should be displayed on the monitor.
Since the input string will not contain embedded blanks, we can use the usual input operator ( >>) for input from scan.txt. Associated with this solution is the use of a List Reduction Algorithm that takes a list of 1 numbers and accumulates them one at a time. The algorithm requires a repetition structure so that we can visit every element in the list. It can be described in the following steps.
I. initialize an accumulator to 0
II. loop to visit each list element
III. update the accumulator as you visit each list element
Well use this algorithm to accumulate the double value that is stored on each line. Assignment 4 Specifications: Input Read a tool name and a tool cost from the input file scan.txt Output Display on the monitor the tool name and tool cost for each input line Output Display on the monitor the total number of tools and the total costs of all the tools
Object Analysis and Algorithmic Development Well need at least a string and a double object to read the data in from the file. In addition, well need an ifstream to connect to our input file. Finally, well need another double to accumulate the tool prices that are stored in the file as well as an int to accumulate the number of tools in the file. Lets start our algorithmic development at the usual place. 1. Input 2. Process 3. Output
Well need to do some preparation for the input in that well need to connect to the file and well also need to initialize our accumulators for the List Reduction Algorithm described above. In order to process every line within the file without knowing how many lines are in the file, well need to implement an end-of-file loop. This is accommodated by attempting to read the first line of the file and then entering a loop that is controlled by the ifstream.eof() function. Within that loop, well need to update our accumulators and display the current input line before we attempt to read another line from the file. After the loop, we simply display our accumulators with appropriate labels. Our algorithm is looking like the following sequence. 1. process initialize file and accumulator variables 2. output display a descriptive message about the program and the output headings 3. input attempt to read the string of the first item of the file 4. process determine if the end-of-file condition is true (a) input read the double value cost from the current input line (b) process to update the total cost of the tools (c) process to update the total number of tools (d) output display the tool name and cost for the current line (e) input attempt to read the string of the item from the next line of the file
5. output the total cost and the total number of tools in the file 6. process disconnect from the input file
Steps 4.(a), through 4.(e) are indented since they will be repeated for each line of input in the file scan.txt. Our final algorithm, with some renumbering, will look like the following. Note that lines in blue represent areas of code that require you to modify or write code to complete the assignment.
Statment | Data Objects |
---|---|
1) connect the input file object to scan.txt | ifstream objects and string constants |
2) initialize accumulators to 0 | double and int objects and constants |
3) display a descriptive message and headings | string constants |
4) attempt to input the first tool name from the file | ifstream & string objects |
5) test the ifstream.eof() condition | bool method and ifstream object |
6) input the cost from the file | ifstream & double objects |
7) update the total cost | double objects |
8) update the total number of tools | int object and constant |
9) display the current tool and its cost | ifstream, string and double objects and string constants |
10) attempt to read another tool name | ifstream & string objects |
11) display the total number of tool costs and the total number of tools | double and int objects and string constants |
12) disconnect from the input file | ifstream object and close() method |
Step 6 through Step 10 are in the while loop determined at Step 5.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
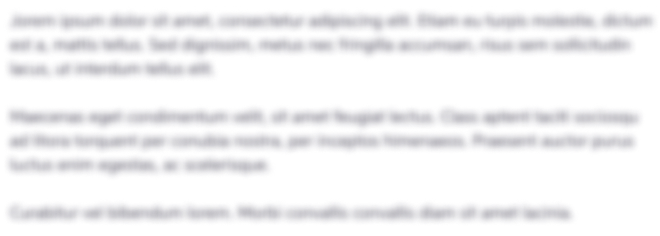
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started