Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment 5 : Implementation of Priority Queue ADT Using NodeListG Implement the Priority Queue Abstract Data Type ( ADT ) using the NodeListG as defined
Assignment : Implementation of Priority Queue ADT Using NodeListG
Implement the Priority Queue Abstract Data Type ADT using the NodeListG as defined in Lesson This
implementation should employ ObjectOriented Programming OOP principles and C templates,
enabling the ADT to manage elements of any type efficiently.
Part : Implementation
NodeListG Implementation
Incorporate all the cpp and h files previously utilized to implement the NodeListG as part of the List
Abstract Data Type ADT from Lesson :
#ifndef DNODEGH
#define DNODEGH
#include
using namespace std;
template
class NodeListG;
template
class IteratorG;
template
class DNodeG
private:
T elem;
DNodeG next;
DNodeG prev;
friend class NodeListG;
friend class IteratorG;
;
#endif
PriorityQueueG Implementation
Utilize PriorityQueueG class Lesson example :
#ifndef PRIORITYQUEUEINTERFACEPRIORITYQUEUEGH
#define PRIORITYQUEUEINTERFACEPRIORITYQUEUEGH
template Element, comparator and Exception
class PriorityQueueG
public:
virtual ~ PriorityQueueG default;
virtual int size const ; number of elements
virtual bool isEmpty const ; is the queue empty?
virtual void insertconst E& e; insert element
virtual const E& min const throwR; minimum element
virtual void removeMin throwR; remove minimum
;
#endif
ListPriorityQueueG Class Implementation
Implement the templated class ListPriorityQueueG to manage elements in a priority queue using
NodeListG from Lesson This class should be flexible to handle any type of elements defined by the
user with the help of a comparator for ordering.
ListPriorityQueueG.h Header File:
Member Variables Private:
o NodeListG L; priority queue contents, E represents the element type
o C comp; comparator
Member Functions Public:
o ListPriorityQueueG;
o virtual ~ListPriorityQueueG;
o int size const; Returns the number of elements in the queue
o bool empty const; Checks if the queue is empty
o void insertconst E& e; insert an element into the queue.
o const E& min const throwR; returns the min element using comp.
o void removeMin throwR; remove the min element using comp.
ListPriorityQueueG.cpp Implementation File:
Implement all member functions declared in ListPriorityQueueG.h
PointD and Comparators Implementation
Utilize PointDSTL rename it PointD and LeftRight comparator classes Lesson example :
#ifndef POINTDSTLH
#define POINTDSTLH
#include
using namespace std;
class PointDSTL
private:
double x y;
public:
PointDSTL
PointDSTLdouble xcoord, double ycoord
x xcoord;
y ycoord;
double getX const return x;
double getY const return y;
friend ostream& operatorostream& out, const PointDSTL& q
out qgetX qgetY;
return out;
;
class LeftRight
public:
bool operatorconst PointDSTL& p const PointDSTL& q const
return pgetX qgetX;
;
#endif
Exception Handling Implementation
Utilize RuntimeException and PriorityQueueException classes Lesson example :
#ifndef STACKEXCEPTIONH
#define STACKEXCEPTIONH
#include
#include "RuntimeException.h
using namespace std;
Exception thrown on performing top or pop of an empty stack.
class PriorityQueueException: public RuntimeException
public:
PriorityQueueExceptionconst string& err : RuntimeExceptionerr
;
#endMain Function Implementation
Implement the main function in a separate cpp file name it main.cpp to demonstrate the functionality
of the ListPriorityQueueG class.
Test ListPriorityQueueG operations in the main function. Inside the main function:
Create an object of type ListPriorityQueueG
Add different PointD objects.
Utilize a while loop to print all PointD objects by calling min and removeMin member
functions.
Create an object of type ListPriorityQueueG
Add different PointD objects.
Utilize a while loop to print all PointD objects by calling min and removeMin member
functions.
Part : Implementation Explanation Document
In addition to the practical coding component of this assignment, you are required to submit a brief
document explaining your implementation. This document should provide insights into your design
choices, the challenges you encountered, and how you tested your program to ensure its correctness.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
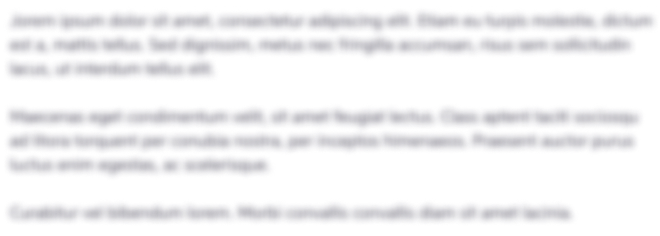
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started