Question
Assignment 5a: Item Design a class that stores information about products that are sold in a store. Write a class named Item that has (at
Assignment 5a: Item Design a class that stores information about products that are sold in a store. Write a class named Item that has (at least) the following member variables: name: a String that holds the name of the inventory item. vendor: a String that stores the name of the vendor for the product. salePrice: a double that stores the current selling price of the item (how much the customer pays to buy it). costPrice: a double that stores the current cost price of the item (how much the store pays to acquire it). weight: a double that stores the weight of a single item of this type. taxable: a boolean field that indicates whether or not tax is charged on the item. Make sure to choose appropriate access specifiers for the data members and methods in your Item class. In addition, the class should have the following member methods: Constructor. The constructor should accept the items name, cost price, and selling price as arguments and assign these values to the object's name, costPrice, and salePrice member variables. The constructor should initialize the weight to 1 andtaxable to true. Accessors. Appropriate accessor methods should be created to allow values to be retrieved from an object's name, vendor, salePrice, weight, and taxable member variables. Mutators. Appropriate mutator methods should be created to allow values to be changed in an objects weight andtaxable fields. increaseCost. This method should increase the cost price by 1 dollar. This is a void method since it modifies the current state of the object. profit. This method should accept no parameters and return the profit on the item, which is calculated as the cost price subtracted from the selling price. Demonstrate the class in a program that creates an Item object. Then increase the cost 3 times by a dollar, calculate the profit and display it on the screen. Call the mutator method that sets the weight to a number you specify. Create several other Item objects and see that the fields have different values. If your Item class is written properly, the following lines of code should be valid in your main method: Item chair = new Item("Desk Chair", 30, 55); //increase cost price by $3. chair.increaseCost(); chair.increaseCost(); chair.increaseCost(); //display the profit System.out.println("The chairs profit is now $" + chair.profit()); //set the chairs weight to 7 lb chair.setWeight(7); Item table = new Item("Picnic Table, 70, 88); System.out.println("The tables profit is now $" + table.profit()); Assignment 5b: Item cont. Part I: This is a continuation of the Item class we designed in the last assignment. Add a toString method to your Item class. This method does not accept any parameters and returns a String reflecting the current state of the object. Once you have written the toString method, the following statement will display the contents of all member variables in an Item object called chair: System.out.println(chair); Part II: Create an array of Item objects. Then write a loop that calculates the total weight of the Items in the array. Since the weight is a private data member of the Item class, the loop will need to call an accessor method to query the weight of each Item in the array. //Here my solution for Assignment# 5a:
public class Item {
private String name;
private String vendor;
private double salePrice;
private double costPrice;
private double weight;
private boolean taxable;
public Item(String name, double sPrice, double cPrice) //constructor 3 parameters
{ this.name=name; salePrice=sPrice;
costPrice=cPrice; taxable=true; weight=1; }
public String getName() //accessors {
return name; }
public String getVendor() {
return vendor; }
public double getSalePrice() {
return salePrice; }
public double getWeight() {
return weight; }
public boolean isTaxable() {
return taxable; }
public void setWeight(double weight) //mutator {
this.weight=weight; }
public void setTaxable(boolean t) {
taxable=t; }
public void increaseCost() {
costPrice++; }
public double profit() {
return salePrice-costPrice; }
public String toString() {
return "Item name: "+name+", weight is: "+weight+", sale price: "+salePrice+", cost price is: "+costPrice+", taxable: "+taxable+", vendor is: "+vendor; }}
//and my ItemRun:
public class ItemRun {
public static void main(String[]args) {
Item table=new Item("Table ", 55, 30);
table.increaseCost();
table.increaseCost();
table.increaseCost();
System.out.println("The table profit is: $ "+table.profit());
table.setWeight(7);
Item chair=new Item("Chair ",12, 10);
chair.setWeight(4);
chair.increaseCost();
System.out.println("The chair profit is: $ "+chair.profit());
Item desk=new Item("Desk ", 20, 15);
desk.setWeight(5);
desk.increaseCost();
desk.increaseCost();
System.out.println("Desk profit is: $ "+desk.profit()); }}
//Now I need to do Assignment #5b (continuation from assignment 5a, Part 1 and Part 2 //Thank you
Step by Step Solution
There are 3 Steps involved in it
Step: 1
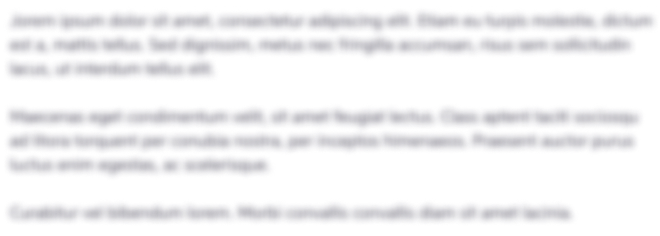
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started