Question
Assignment 7 This assignment will use the Employee class that you developed for assignment 6 (see below). Design two sub-classes of Employee...SalariedEmployee and HourlyEmployee. A
Assignment 7
This assignment will use the Employee class that you developed for assignment 6 (see below). Design two sub-classes of Employee...SalariedEmployee and HourlyEmployee. A salaried employee has an annual salary attribute. An hourly employee has an hourly pay rate attribute, an hours worked attribute, and an earnings attribute. An hourly employee that works more than 40 hours gets paid at 1.5 times their hourly pay rate. You will decide how to implement constructors, getters, setters, and any other methods that might be necessary.
1. Draw a UML diagram for the classes.
2. Implement the classes, and write a test program that creates a salaried employee and two hourly employees. One of the hourly employees should have hours worked set to less than 40 and one should have hours worked set to more than 40. The test program should display all attributes for the three employees. To keep things simple, the employee classes dont need to do any editing.
Assignment 6 Code:
import java.util.Scanner;
public class employeeInfo { static class Employee // class employee
{
private int number; // instance variable number
private Name name; // instance object of class Name
private Address address; // instance object of class address
private Date hireDate; // instance object of class hireDate
public int getEmpNumber() // getEmpNumber function method
{
return number;
}
public void setEmpNumber(int n) // setEmpNumber function method
{
this.number = n;
}
public Name getName() // getEmpName function method as Name class
{
return name;
}
public void setName(Name name) // setEmpName function method as Name class
{
this.name = name;
}
public Address getAddress() // getAddress function method as Address class
{
return address;
}
public void setAddress(Address address) // setAddress function method as Address class
{
this.address = address;
}
public Date getHireDate() // getHireDate function method as Date class
{
return hireDate;
}
public void setHireDate(Date hireDate) // setHireDate function method as Date class
{
this.hireDate = hireDate;
}
}
static class Name // this is name class
{
private String FirstName; // its instance variables FirstName and lastName
private String LastName;
public Name() // constructor
{
FirstName = "";
LastName = "";
}
public void setFirstName(String firstName) // setFirstName method
{
FirstName = firstName;
}
public void setLastName(String lastName) // setLastName method
{
LastName = lastName;
}
public String getFirstName() // getFirstName method
{
return FirstName;
}
public String getLastName() // getLastName method
{
return LastName;
}
@Override
public String toString() // toString method
{
return FirstName + " " + LastName;
}
}
static class Address // address class
{
private String fullAddress, street, city, state; // its instance variable as private
private int zip;
public String getStreet() // getStreet method
{
return street;
}
public void setStreet(String street) // setStreet method
{
this.street = street;
}
public String getCity()
{
return city;
}
public void setCity(String city)
{
this.city = city;
}
public String getState()
{
return state;
}
public void setState(String state)
{
this.state = state;
}
public int getZip()
{
return zip;
}
public void setZip(int zip)
{
this.zip = zip;
}
@Override
public String toString() // toString method
{
return (this.street + " " + this.city + ", " + this.state + " " + String.valueOf(this.zip));
}
}
static class Date // date class
{
private int month; // instance variables
private int day;
private int year;
public Date() // constructor
{
month = 0;
year = 0;
day = 0;
}
public void setDay(int dayOfMonth) // setDay method
{
day = dayOfMonth;
}
public void setMonth(int monthOfYear) // setMonth method
{
month = monthOfYear;
}
public void setYear(int whichYear) // setYear method
{
year = whichYear;
}
public int getDay() // getDay method
{
return day;
}
public int getMonth() // getMonth method
{
return month;
}
public int getYear() // getYear method
{
return year;
}
@Override
public String toString() // toString method
{
return String.format("%d-%d-%d", month, day, year);
}
}
public static void main(String[] args)
{
Scanner input = new Scanner(System.in); // creating the object of the scanner class
int numEmployees;
while (true) {
System.out.print("How many employees data do you wish to enter? "); // taking the no of employee
numEmployees = Integer.parseInt(input.nextLine());
if (numEmployees < 0) {
System.out.println("** Invalid entry. Please try again **");
continue;
} else
break;
}
Employee[] employeeArray = new Employee[numEmployees]; // creating the array of the type employee class with // size entered by the user
for (int i = 0; i < numEmployees; i++)
{ Employee e1 = new Employee(); // creating object of employee class
Name name = new Name(); // creating object of name class
Date dateHiring = new Date(); // creating object of date class
Address address = new Address(); // creating object of address class
System.out.println(" Enter the information of Employee " + (i + 1) + " ");
System.out.print("Enter the employee number: "); // taking the employee no
e1.setEmpNumber(Integer.parseInt(input.nextLine())); // call the setEmpNumber method to set the Employee // number of employee class
System.out.print("Enter the first name of the employee: ");
name.setFirstName(input.nextLine()); // call the setFirstName method to set the First name of Name class
System.out.print("Enter the last name of the employee: ");
name.setLastName(input.nextLine()); // call the setLastName method to set the Last name of Name class
e1.setName(name); // now call the setName method of Employee class
System.out.print("Enter the street of the employee: ");
address.setStreet(input.nextLine()); // call the setStreet method to set the Street of Address class
System.out.print("Enter the city of the employee: ");
address.setCity(input.nextLine()); // call the setCity method to set the City of Address class
System.out.print("Enter the state (Ex. CA for California) of the employee: ");
address.setState(input.nextLine()); // call the setState method to set the State of Address class
System.out.print("Enter the 5 digit zip code of the employee: ");
address.setZip(Integer.parseInt(input.nextLine())); // call the setZip method to set the zip code of Address // class
e1.setAddress(address); // now call the setAddress method of Employee class
while (true)
{
System.out.print("Enter the day of hiring: ");
int day = Integer.parseInt(input.nextLine()); // taking the day from the user and check it is valid or // not
if (day < 0 || day > 31)
{
System.out.println("Please enter a valid date.");
continue;
} else
{
dateHiring.setDay(day); // if valid the call setDay method of the Date class
break;
}
}
while (true)
{
System.out.print("Enter the month of hiring in numbers (Ex. 4 for April): "); // taking the month from // the user and check it // is valid or not
int m = Integer.parseInt(input.nextLine());
if (m < 0 || m > 12)
{
System.out.println("Please enter a valid month.");
continue;
} else
{
dateHiring.setMonth(m); // if valid the call setMonth method of the Date class
break;
}
}
while (true)
{
System.out.print("Enter the year of hiring: "); // taking the year from the user and check it is valid // or not
int y = Integer.parseInt(input.nextLine());
if (y < 1990 || y > 2020)
{
System.out.println("Please enter a valid year between 1990 and 2020.");
continue;
} else
{
dateHiring.setYear(y); // if valid the call setYear method of the Date class
break;
}
}
e1.setHireDate(dateHiring); // now call the setHireDate method of Employee class
employeeArray[i] = e1; // now add the object e1 to the employeeArray
}
// Print Employees
int count = 1;
System.out.println("\t\t Employees information ");
System.out.println("--------------------------------------------------------------");
for (Employee e : employeeArray)
{
System.out.println("Employee " + count + " information ");
System.out.println("*************************************************************");
System.out.print("Employee Number is :" + e.getEmpNumber());
System.out.print(" Employee Name is: " + e.getName());
System.out.print(" Employee Full Address is: " + " " + e.getAddress());
System.out.print(" Employee Hire-Date is :" + e.getHireDate());
count++;
System.out.print(" ");
}
input.close(); // close the scanner
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
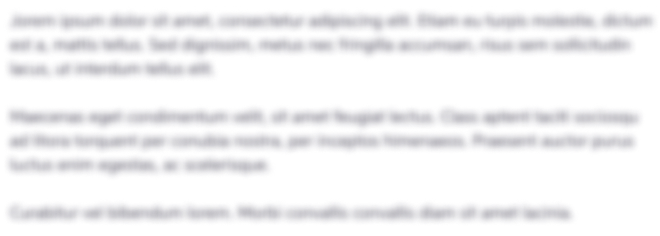
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started