Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment Description In this assignment you will create a password tester. The program will ask the user for a password and then determine if the
Assignment Description
In this assignment you will create a password tester. The program will ask the user for a password and then determine if the password meets the requirements. A valid password must adhere to the following four rules in this order:
Must be between and characters.
Must contain only letters and digits.
Must contain and only digits.
Must not be the same as the last two previous passwords.
o For this assignment, you can hard code the following as the previous passwords:
final String PREVIOUS "cooper;
final String PREVIOUStomac;
The program will allow the user attempts to enter a password that meets the requirements.
After the th try if a valid password was not entered the program will end.
Specifications
Add this assignment to your project called CS
Create a Java class within that project called LastNameFirstNameAssignment
Follow CS Programming Assignments Policy"
Write code that:
Allows the user to repeatedly enter a password up to attempts.
Gracefully handles an invalid password one that doesnt meet the requirements:
o Handling an invalid password must be done in main.
o Each test method returns a boolean.
o For the password to be valid it must pass all tests otherwise another loop iteration needs to occur where the user reenters the password, and the tests are run again.
o See Output examples for more details.
Step : Declare and initialize any constants. Declare any variables needed before the loop.
Step : Create a loop in main that allows the user to repeatedly enter a password.
o The loop continues while the password is invalid and attempts have not occurred.
o The loop could look something like the following:
boolean passwordPassedTests false;
while passwordPassedTests && numberAttempts ATTEMPTSALLOWED
Ask user to reenter the password and perform the tests
If all tests pass, set passwordPassedTests true
o The code inside the loop must:
Prompt the user to enter the password and store the password in a String.
Update the number of attempts counter.
Test the password against each rule in the order shown above.
Each password test has a method see # below.
If any test fails
Skip all remaining tests after that point and start the loop again
Step : When loop completes display a message indicting if the password meets all requirements valid or failed the requirements invalid
Design your program to use the following methods:
Perform test to determine if passwords length is between minLength and maxLength
public static boolean lengthTest String password, int minLength, int maxLength
Perform test to determine if password contains only digits and letters
public static boolean onlyLettersAndDigitsTest String password
Perform test to determine if password contains only digits
public static boolean containsTwoDigitsTest String password
Perform test to determine if password is different than previous two passwords
public static boolean differentThanLastTwoPasswordsTest String previousPassword
String previousPassword
String password
Display a specific error message based on the error codes :
length test error
only lettersdigits test error
only twodigits test error
different than previous passwords error
Requires using a switch statement
public static void printErrorMessage int errorCode
Must Do and Tips
Must Do: In your design notebook, you must include pseudocode for main and these methods:
onlyLettersAndDigitsTest
containsTwoDigitsTest
Must Do: Use constants for the different errors:
There are several constants that can be created for this assignment.
At a minimum, you must create constants for each error, for example:
final int LENGTHERROR ;
Must Do: Create each method as defined in assignment sheet.
Create the methods as defined in step with the given return type and parameters.
You can add more methods, but you must write all the methods in step as shown.
Do not change the return types or parameters of these methods.
These methods but all be used in your solution to perform the different tests.
Must Do: Call printErrorMessage only in main.
The printErrorMessage method is the only place in the code where the test results are displayed.
The method should only be called in main.
The method must use a switch statement.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
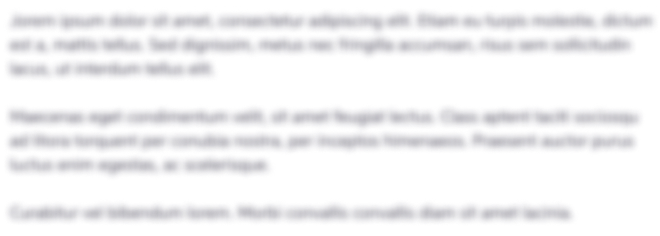
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started