Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Assignment Description Write a program to create license plates for Colorado, Wyoming, and Utah. The program will prompt the user to enter two values a
Assignment Description
Write a program to create license plates for Colorado, Wyoming, and Utah. The program will prompt the user to enter two values a character and a number and then displays a randomly generated license plate based on those two characters. The character represents the state, and the number represents the type of license plate. For this assignment, use following characters to denote the state and plate type:
C: Colorado
W: Wyoming
U: Utah
: Regular license plate
: Adopt a Shelter Pet license plate
: Fire Fighter license plate
Once state and plate type are BOTH obtained, generate a random license plate using the following given patterns. Be sure to notice where there are spaces and dashes in the different license plates:
State
Description
Template
Example
Colorado
digits, a dash, letters
###XXX
TXV
Wyoming
digit, a space, digits
# #####
Utah
letters a space, digit, letter
XXX #X
PWW M
# represents a number X represents a letter
Specifications
Add this assignment to your project called CS
Create a Java class within that project called LastNameFirstNameAssignment
Follow CS Programming Assignments Policy"
Write code that:
Prompts user for the state and plate type
Read the entered information as one string eg user enters C for Colorado Adopt a Pet
Extracts from the string the state the character CW or U and ensures it is valid.
Extracts from the string the type the character or and ensures it is valid.
Once state and type are validated:
o Generate a random license plate based on the state and plate type.
o Display state name, number, and plate type.
o For example: Wyoming Adopt a Shelter Pet
The code must handle user validation for:
State
o If an invalid state is entered, MUST print message and END PROGRAM
Type
o If invalid plate type is entered, MUST print message and END PROGRAM
Note:
o END PROGRAM means: once an input error occurs, the program must display an error message and perform no additional processing.
o For example:
If the user enters P when asked for the characters, the program must display an invalid state error message and complete at that point.
At this point, your code must NOT process the type of license plate.
See error output example # below.
o Use NESTED IF statements to properly handle errors in user input.
The if statement performs a test say on the state character and if the test fails the else clause executes, displays the error message, and the program terminates.
DO NOT use System.exit to exit program if an error occurs.
DO NOT use break or return statements if an error occurs.
Using System.exit, return, or break will result in loss of points for correctness.
The purpose is to learn to write properly nested ifstatements.
Must Do and Tips
Must Do: General
Reading user input
o The state and plate type must be entered on the same line.
This means both values must be entered before the enter key is pressed.
o Read user input for the state and plate type as a String
Use the nextLine method in Scanner class to read state and type as a String.
Next extract each character from the string using the charAt method.
Your code must accept lowercase or uppercase values for the state character.
Use correct data types and constants
o To eliminate magic numbers use constants to increase code readability.
Magic numbers are numeric values in the code whose meaning is not known.
o Constants should be used for all the different states, for example:
final char COLORADO C;
Must use nestedif and switch statement
o Must use nested ifstatements for the main structure of the code, not a multiwayif
o Uses a switch statement when printing each license plate see lecture #
Must Do: Use random method provided in Math class
Use the random method in the Math class Math.random read all about it in section
Generate different letters and numbers for each number or letter in the license plate.
DO NOT generate one letter or number and use it repeatedly.
DO NOT use the Random class provided by Java.
DO NOT create a string of numbers or letters and then randomly get values out of that string.
DO NOT create an array of numbers or letters and then randomly get values out of the array.
Tip: Play with the random method to learn how it works
Use Math.random and the ASCII table in Appendix B to create the necessary values.
To create numbers:
o You do not need to worry about generating numbers with leading zeros such as
o You may assume the following:
When creating digit value, the values run between and
When creating digit value, the values run between and
When creating digit value, the values run between and
When creating digit value, the values run between and
Step by Step Solution
There are 3 Steps involved in it
Step: 1
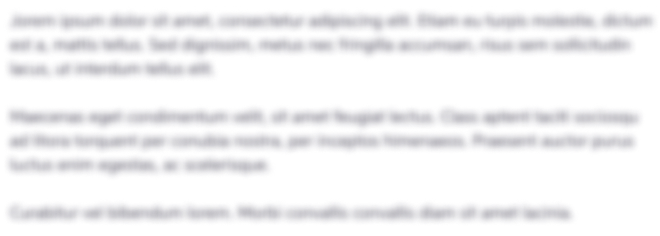
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started