Question
Assignment for Computer Science in python - Making the game Mastermind (text-based) For this assignment you will create a version of the popular game mastermind!
Assignment for Computer Science in python - Making the game Mastermind (text-based)
For this assignment you will create a version of the popular game mastermind! You can play an online version of the game here: Mastermind online (Note, your game will look and behave a bit differently).
The rules for your game are as follows:
The computer generates a random password according to the following rules:
The password is 5 integers long
The password will consist of integers in the range [1,9]
The password should not have any duplicate values.
An example password would be: [9,1,7,4,2]
Every run of the game should generate a different password
The random.sample() and random.shuffle() functions can solve this problem trivially, and so their use will result in a mark of 0 for this portion of the assignment.
The user then has 10 turns to guess the password. Each turn, the user should be notified of how many turns remain.
Each turn the user makes one guess, by entering 5 digits (at a single prompt).
Next, the computer reports the the user's result as follows:
The number of digits in the user's guess that appear (anywhere) in the password.
The number of digits in the user's guess that exactly match the digit in the password (same digit, same position).
If the user guesses all five digits correctly (correct digits and correct positions), the game ends with a win message.
If the user runs out of turns, the game ends with a lose message. This should include a 'cleanly' formatted display of the correct password (e.g., 1 2 3 4 5, not [1,2,3,4,5]).
When the game ends, your program should ask the user if they would like to play again. This should be a yes or no question, and any invalid input should cause the question to be repeated.
You may assume that the user correctly enters 5 numbers each turn, however you must allow them to enter all 5 into a single prompt (separated by spaces as in the examples below). (Tip: the string.split() method might be useful here.)
Your code must be structured in a procedural manner. That is, your code should have a main() method that orchestrates the main logic of your game, and it should have (at minimum) functions to perform the following operations. Please note, your implementation must use the names given here.
generatePassword() - This function should generate a random password according to the rules above, and return it as a list.
getUserGuess() - This function should prompt the user for their guess and return a list of the integers that they entered.
reportResult(password,guess) - This function should compare the user's guess to the secret password and determine the results according to the rules above. It should display to the user their results (see examples below), and it should return a boolean value: True if the user guessed all 5 digits correctly (i.e., the win condition), and False otherwise.
You may use additional functions as you deem necessary, and name them as you wish.
Here are some example runs of the program. User input is highlighted:
>python mastermind.py I've set my password, enter 5 digits in the range [1-9] (e.g. 9 3 2 4 7): 10 guesses remaining. > 1 2 3 4 5 2 of 5 correct digits. 0 of 5 correct locations. 9 guesses remaining. > 2 3 4 5 6 2 of 5 correct digits. 0 of 5 correct locations. 8 guesses remaining. > 3 4 5 6 7 3 of 5 correct digits. 0 of 5 correct locations. 7 guesses remaining. > 4 5 6 7 8 3 of 5 correct digits. 0 of 5 correct locations. 6 guesses remaining. > 5 6 7 8 9 3 of 5 correct digits. 1 of 5 correct locations. 5 guesses remaining. > 5 6 1 2 3 1 of 5 correct digits. 0 of 5 correct locations. 4 guesses remaining. > 3 4 7 8 5 4 of 5 correct digits. 1 of 5 correct locations. 3 guesses remaining. > 6 4 7 9 5 3 of 5 correct digits. 1 of 5 correct locations. 2 guesses remaining. > 4 3 1 8 5 3 of 5 correct digits. 0 of 5 correct locations. 1 guesses remaining. > 8 4 3 7 5 4 of 5 correct digits. 1 of 5 correct locations. You'll never get my treasure! The password was 8 9 7 3 4. Would you like to play again? (y/n) maybe Would you like to play again? (y/n) y I've set my password, enter 5 digits in the range [1-9] (e.g. 9 3 2 4 7): 10 guesses remaining. > 1 2 3 4 5 2 of 5 correct digits. 0 of 5 correct locations. 9 guesses remaining. > 3 4 5 6 7 4 of 5 correct digits. 1 of 5 correct locations. 8 guesses remaining. > 4 3 5 6 8 4 of 5 correct digits. 2 of 5 correct locations. 7 guesses remaining. > 4 3 6 5 9 3 of 5 correct digits. 1 of 5 correct locations. 6 guesses remaining. > 4 3 7 8 5 4 of 5 correct digits. 2 of 5 correct locations. 5 guesses remaining. > 4 7 3 8 6 4 of 5 correct digits. 4 of 5 correct locations. 4 guesses remaining. > 4 7 5 8 6 5 of 5 correct digits. 5 of 5 correct locations. Drat! You guessed my password! The treasure is yours... Would you like to play again? (y/n) n Thanks for playing.
Grading Scheme
/6 marks - generates a unique 5 digit password with no duplicates
/4 marks - correctly accepts user input
/7 marks - correctly reports the result of a user's guess
/4 marks - game loop iterates through each turn of the game correctly
/4 marks - procedural design was used, with required methods implemented
/5 marks - game ending conditions implemented correctly and prompt to play again
/5 marks - Documentation (comments and code-organization).
Total - [/35]
Step by Step Solution
There are 3 Steps involved in it
Step: 1
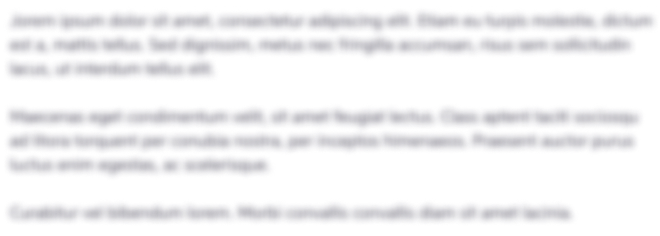
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started