Question
Assignment---------------------------------------------------------------------------- Goal: Create a list-like data structure that knows its own length and can grow as needed. Name the source file for this lab pokedex.cpp.
Assignment----------------------------------------------------------------------------
Goal: Create a list-like data structure that knows its own length and can grow as needed.
Name the source file for this lab pokedex.cpp.
In this lab you'll create a Pokedex object that is a collection of Pokemon. A (((((((((Pokedex is like an array, but it only holds Pokemon,)))))))))))))))
it knows its length, and it grows as we add Pokemon to it. An array can't grow once we've told it how many elements to hold, and it doesn't know its own length. When the program runs, it will output the contents of a Pokedex to the console.
Copy your Pokemon and Move structs from lab-13 into your source code for this lab, we'll be using them again. Make sure the first letter of each struct is capitalized: Pokemon and Move, not pokemon and move.
Declare a new struct called Pokedex with two fields with the following names and types:
- p : A pointer to a Pokemon. - rest: A pointer to a Pokedex.
Remember, to declare a variable that is a pointer to some type::
There are two types of Pokedex values: empty and non-empty. An empty Pokedex will have p and rest equal to the null pointer, NULL, which is a pointer to invalid memory. We use NULL to say: "this points to nothing"::
Pokedex theEmptyPokedex = {NULL, NULL};
A non-empty Pokedex value contains a pointer to a single Pokemon, and then a pointer to the rest of the Pokedex. Conceptually, a Pokedex with three Pokemon in it could be represented by this diagram::
{p=Charizard, rest=-->{p=Treeko, rest=-->{p=Tepig, rest=-->EMPTY_POKEDEX
In the above diagram, Charizard is inside a Pokedex struct as p, and rest points to the Pokedex struct that has Treeko as p, that in turn points to the struct that has Tepig as p, and the struct that has Tepig as p has a rest that points to an empty Pokedex. This empty Pokedex is how we track "the end of the list".
Before writing the first function of this lab, I would recommend adding an empty main function so that you may compile the source as you go along, checking for errors as you go. If you don't include at least an empty main function, the compiler will complain in an opaque way that it can't find a main function.
empty ===== Write a function named empty which returns a pointer to a Pokedex whose two fields are set to NULL. IMPORTANT: Use the new keyword to create the Pokedex value. We use the new keyword so that the object we create is created "on the heap", and will live beyond the life of the function call. If we don't do this, the function will return a pointer to a memory address in a dead stack-frame that no longer exists once the function returns. An example of how to use new to create a new Pokedex object and store the address of the value into a variable::
Pokedex* yourVariableNameHere = new Pokedex {
isEmpty ======= Write a function named isEmpty which accepts as an input a pointer to a Pokedex, and returns true if the Pokedex is empty, and false otherwise. Remember, a Pokedex is empty if it contains null pointers.
If you have a pointer to an object, then you use the '->' operator to access its fields. Example::
CoolObject* foo = new CoolObject{...}; // Create some value of type CoolObject. foo->bar; // We have a pointer, so we access a field named 'bar' like this.
length ====== Write a function named length, which takes a pointer to a Pokedex, and returns the number of Pokemon in that Pokedex as an int. Following are English descriptions of two different approaches to implementing this function:
- Recursive: If I have the empty Pokedex, the length is zero, otherwise the length of the Pokedex is 1 plus the length of the rest of the Pokedex. - Iterative: While I haven't reached the end of the Pokedex, add 1 to the length. Return the length after looping.
prepend ======= Write a function named prepend. Conceptually, prepend adds a Pokemon to the beginning of a Pokedex. prepend takes as input a pointer to a Pokemon and a pointer to a Pokedex. prepend returns a pointer to a *new* Pokedex. Make sure to use the 'new' keyword when creating the new Pokedex you'll return from this function. We want this Pokedex value to live on the heap so it continues to exist after the function returns, and thus the pointer to the new Pokedex will be valid after the function returns.
Hint: A Pokedex is a linked-list of Pokedex values, so the new Pokedex value you create will somehow "point" to the existing Pokedex value you were given.
shortDescription ================ Write a function named shortDescription which takes a **pointer** to a Pokemon as input, and returns a string describing the Pokemon as follows:
Examples:
Charmander (Fire) Noctowl (Flying)
Notice that this shortDescription function is very similar to the shortDescription function from lab-13, except it takes a pointer to a Pokemon value instead of a Pokemon value. Remember to use the -> operator with a pointer to access fields of the object it points to.
index ===== Write a function named index that takes a pointer to a Pokedex and returns a string representing a list of the short descriptions of all Pokemon in the Pokedex. Each short description should have a newline appended. If given a Pokedex containing five example Pokemon, index should return a string that looks like this when sent to the console::
Charmander (Fire) Noctowl (Flying) Tepig (Fire) Zekrom (Dragon) Reshiram (Dragon)
Remember, this is equivalent to saying that the returned string should equal:
"Charmander (Fire) Noctowl (Flying) Tepig (Fire) Zekrom (Dragon) Reshiram (Dragon) "
Following are English descriptions of two different approaches to implementing this function:
- Recursive: If I have the empty Pokedex, then the index is the empty string, otherwise the index is a short description of the current Pokemon with a newline appended, concatenated with the index of the rest of the Pokedex. - Iterative: While I haven't reached the end of the Pokedex, append a short description of the current Pokemon and a newline to the result. Return the result after looping.
main ==== Write a main function that creates 5 Pokemon values. The rest of the function should create a Pokedex and use 'prepend' to add these Pokemon to the Pokedex, and then use 'length' and 'index' to create console output similar to this (but using your own Pokemon)::
There are 5 Pokemon in the Pokedex: Charmander (Fire) Noctowl (Flying) Tepig (Fire) Zekrom (Dragon) Reshiram (Dragon)
Do **NOT** simply output a string that contains a literal "5" in it, you must use the 'length' function to get this value.
There are a couple ways to do this, but there is one important point that you must consider: prepend accepts a pointer to a Pokemon, not a Pokemon. At the beginning of main, you should have created 5 Pokemon values. Well, you can't simply pass these values to prepend, because the types don't match. You have a Pokemon value, but you need a pointer to a Pokemon value. The trick is the "address of" operator, '&'. Given a variable named 'charizard' which is a Pokemon value, &charizard gives you the address of the value in that variable. So instead of doing this::
prepend(charizard, somePokedexPointer);
...you'll do this instead:: prepend(&charizard, somePokedexPointer);
Here is one approach to implementing this function:
- Create a pointer to an empty Pokedex. - Use prepend to prepend a *pointer to* the first pokemon to the Pokedex. - Use prepend to prepend a *pointer to* the second pokemon to the Pokedex. ...etc. Using this approach, there will be a single line of code for each Pokemon to prepend it to the Pokedex.
An alternative approach is to create the entire Pokedex in one statement. You'll call prepend, and the second argument will itself be a call to prepend, and you'll continue to do this until you've prepended all the Pokemon. The last call to prepend will be prepending the Pokemon to the empty Pokedex. Here is an example with 3 Pokemon variables::
// This will return a pointer to a Pokedex containing 3 Pokemon: prepend(&Charizard, prepend(&Treeko, prepend(&Tepig, empty())));
// Same thing, different style. prepend( &Charizard , prepend(&Treeko , prepend(&Tepig , empty())));
// Same thing, different style. prepend ( &Charizard , prepend( &Treeko , prepend( &Tepig , empty() )));
When you run your program, it should output a line that tells us how many Pokemon are in the Pokedex, followed by a list of the Pokemon in the Pokedex, one per line. You must use the 'length' function when outputting the length of the Pokedex to the console. Make sure every field of every object is initialized to some value.
Note that our program has memory-leaks because we never use the 'delete' keyword to deallocate memory allocated by the 'new' keyword. Don't worry about this for now, you'll have plenty of CS courses and maybe a career in C/C++ where you get to worry about that.
codes that I currently did----------------------------------------------------------------------------------
struct Move
{
string name;
int selfHPEffect; //
int otherHPEffect; //
int selfAtkEffect; //
int otherAtkEffect;
int selfDefEffect; //
int otherDefEffect;
};
struct Pokemon{
string name;//1
string type;//2
int centimeterHeight;//3
int gramWeight;//4
int hp;//5
int attack;//6
int defence;//7
Move move1;//8
Move move2;//9
};
struct Pokedex
{
Pokemon* p;
Pokedex* rest;
};
Pokedex* empty()
{
return new Pokedex {NULL, NULL};
}
bool isEmpty(Pokedex* n)
{
if (n -> p == NULL)
{
return true;
}
else
{
return false;
}
}
Pokedex* length(Pokedex* n)
{
if(n -> p == NULL)
{
return 0;
}
else
{
return 1 + length(rest);// i didnt finish this yet...
}
}
int main()
{
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
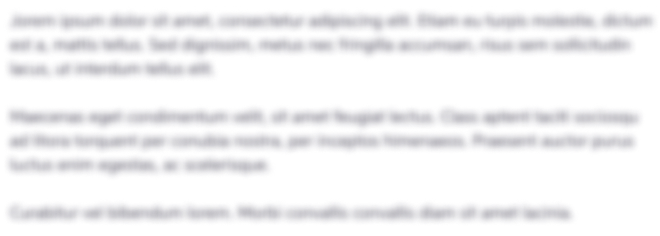
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started